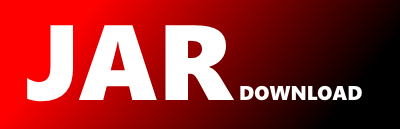
name.didier.david.test4j.junit.TestResourceRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ndd-test4j Show documentation
Show all versions of ndd-test4j Show documentation
Test4J provides testing support.
The newest version!
package name.didier.david.test4j.junit;
import java.io.File;
import java.lang.reflect.Method;
import java.net.URL;
import org.junit.rules.MethodRule;
import org.junit.runners.model.FrameworkMethod;
import org.junit.runners.model.Statement;
import name.didier.david.test4j.unit.TestResourceHandler;
/**
* Provide resource injection explicitly or through {@link name.didier.david.test4j.unit.TestResource} annotations.
*
*
* The pattern is a custom string which may use the following placeholders:
*
*
*
* {c}
is the name of the current test class, given by {@link Class#getSimpleName()}, e.g.
* MyTestCase
or MyTestCase-MyInnerClass
* {f}
is the name of the annotated field, given by {@link java.lang.reflect.Field#getName()}
* {m}
is the name of the current test method, given by {@link java.lang.reflect.Method#getName()}
* {p}
is the package name of the current test class as a path, given by {@link Package#getName()},
* e.g. name/didier/david/test4j/junit
*
*
*
* Usage:
*
*
*
package name.didier.david.test4j.junit;
public class TestResourceRuleTest {
@Rule
public TestResourceRule testResource = new TestResourceRule();
// resolves to name/didier/david/test4j/junit/TestResourceRuleTest-data/<method_name>
// with <method_name> the name ot the current running test method, e.g. "test"
@TestResource("/{p}/{c}-data/{m}")
private File fileField;
// resolves to the content of name/didier/david/test4j/testng/TestNgResourceListenerTest-data/<method_name>
// with <method_name> the name ot the current running test method, e.g. "test"
@TestResource("/{p}/{c}-data/{m}")
private String stringField;
@Test
public void test() {
// resolves to name/didier/david/test4j/junit/TestResourceRuleTest-data/test
File file = testResource.asFile("/{p}/{c}-data/{m}");
// resolves to the content of name/didier/david/test4j/junit/TestResourceRuleTest-data/test
String fileContent = testResource.asContent("/{p}/{c}-data/{m}");
...
}
}
*
*
* @author ddidier
*/
public class TestResourceRule
implements MethodRule {
/** Handle {@link name.didier.david.test4j.unit.TestResource}s. */
private final TestResourceHandler handler;
/** The current instance under test. */
private Object testInstance;
/** The current method under test. */
private Method testMethod;
/** Default constructor. */
public TestResourceRule() {
handler = new TestResourceHandler();
}
@Override
public Statement apply(Statement base, FrameworkMethod method, Object target) {
return new Statement() {
@Override
public void evaluate()
throws Throwable {
before(base, method, target);
try {
base.evaluate();
} finally {
after(base, method, target);
}
}
};
}
/**
* Find the resource with the given pattern as a {@link File}, relative to the current test class and method.
*
* @param pattern the pattern to search for.
* @return the file if found.
* @throws name.didier.david.test4j.unit.TestResourceException if the file cannot be found.
*/
public File asFile(String pattern) {
return handler.findFile(pattern, testInstance, testMethod);
}
/**
* Find the resource with the given pattern as a {@link URL}, relative to the current test class and method.
*
* @param pattern the pattern to search for.
* @return the URL if found.
* @throws name.didier.david.test4j.unit.TestResourceException if the URL cannot be found.
*/
public URL asUrl(String pattern) {
return handler.findUrl(pattern, testInstance, testMethod);
}
/**
* Load the content of the resource with the given pattern, relative to the current test class and method.
*
* @param pattern the pattern to search for.
* @return the content of the resource if found.
* @throws name.didier.david.test4j.unit.TestResourceException if the resource cannot be found.
*/
public String asContent(final String pattern) {
return handler.loadContent(pattern, testInstance, testMethod);
}
/**
* Interpolate the pattern as a relative path, relative to the current test class and method. See
* {@link name.didier.david.test4j.unit.TestResource}.
*
* @param pattern the pattern to interpolate.
* @return the interpolated pattern as a relative path.
*/
public String toPath(final String pattern) {
return handler.toPath(pattern, testInstance, testMethod);
}
/**
* Called before test invocation.
*
* @param base the {@link Statement} to be modified.
* @param method the method to be run.
* @param target the object on which the method will be run.
*/
protected void before(Statement base, FrameworkMethod method, Object target) {
testInstance = target;
testMethod = method.getMethod();
handler.inject(testInstance, testMethod);
}
/**
* Called after test invocation.
*
* @param base the {@link Statement} to be modified.
* @param method the method to be run.
* @param target the object on which the method will be run.
*/
protected void after(Statement base, FrameworkMethod method, Object target) {
handler.clear(testInstance, testMethod);
// testInstance = null;
// testMethod = null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy