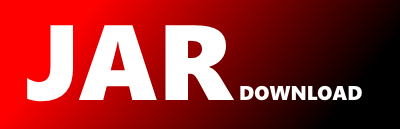
name.didier.david.test4j.unit.DataProviders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ndd-test4j Show documentation
Show all versions of ndd-test4j Show documentation
Test4J provides testing support.
The newest version!
package name.didier.david.test4j.unit;
import static org.apache.commons.lang3.StringUtils.EMPTY;
import static org.apache.commons.lang3.StringUtils.SPACE;
import java.io.File;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* Useful data providers for common testing patterns.
*
* @author ddidier
*/
public final class DataProviders {
/** Utility class. */
private DataProviders() {
super();
}
// -----------------------------------------------------------------------------------------------------------------
/**
* @return {@code null} strings.
*/
public static String[][] nullStrings() {
return new String[][] { { null } };
}
/**
* @return {@code null} and empty strings.
*/
public static String[][] emptyStrings() {
return new String[][] { { null }, { EMPTY } };
}
/**
* @return {@code null}, empty and blank strings.
*/
public static String[][] blankStrings() {
return new String[][] { { null }, { EMPTY }, { SPACE } };
}
/**
* @return empty and blank strings.
*/
public static String[][] emptyButNotNullStrings() {
return new String[][] { { EMPTY }, { SPACE } };
}
// -----------------------------------------------------------------------------------------------------------------
/**
* @return strictly negative numbers.
*/
public static Number[][] strictlyNegativeNumbers() {
return new Number[][] { { -1000 }, { -100 }, { -10 }, { -1 } };
}
/**
* @return negative or zero numbers.
*/
public static Number[][] negativeNumbers() {
return new Number[][] { { -1000 }, { -100 }, { -10 }, { -1 }, { 0 } };
}
/**
* @return strictly positive numbers.
*/
public static Number[][] strictlyPositiveNumbers() {
return new Number[][] { { 1 }, { 10 }, { 100 }, { 1000 } };
}
/**
* @return positive or zero numbers.
*/
public static Number[][] positiveNumbers() {
return new Number[][] { { 0 }, { 1 }, { 10 }, { 100 }, { 1000 } };
}
// -----------------------------------------------------------------------------------------------------------------
/**
* @param the type of the elements in the list.
* @return {@code null} and empty {@link List}.
*/
@SuppressWarnings("unchecked")
public static List[][] emptyLists() {
return (List[][]) new List>[][] { { null }, { new ArrayList<>() } };
}
/**
* @param the type of the elements in the set.
* @return {@code null} and empty {@link Set}.
*/
@SuppressWarnings("unchecked")
public static Set[][] emptySets() {
return (Set[][]) new Set>[][] { { null }, { new HashSet<>() } };
}
// -----------------------------------------------------------------------------------------------------------------
/**
* @return non existing files: empty path, blank path, invalid path.
*/
public static File[][] nonExistingFiles() {
// @formatter:off
return new File[][] {
{ new File(EMPTY) },
{ new File(SPACE) },
{ new File("some_hopefully_invalid_file@@@123") },
};
// @formatter:on
}
// -----------------------------------------------------------------------------------------------------------------
// Digests and conversions done with:
// - http://www.freeformatter.com/hmac-generator.html
// - http://tomeko.net/online_tools/hex_to_base64.php?lang=en
/**
* @return a pair message/signature (HMAC using MD5 encoded in hexadecimal).
*/
public static String[][] hmacMd5Base16() {
// @formatter:off
return new String[][] {
{ "something to sign MD5/16", "92a98473515a3c1603dadf62f74f3919" },
{ "another thing to sign MD5/16", "b20e2291beec4c7ef905717425f68bb9" },
{ "something to sign MD5/16", "92a98473515a3c1603dadf62f74f3919" },
{ "another thing to sign MD5/16", "b20e2291beec4c7ef905717425f68bb9" },
};
// @formatter:on
}
/**
* @return a pair message/signature (HMAC using MD5 encoded in base 64).
*/
public static String[][] hmacMd5Base64() {
// @formatter:off
return new String[][] {
{ "something to sign MD5/64", "rV/ZLc5nCerkL+p8URqmVg==" },
{ "another thing to sign MD5/64", "W6wfT321SH/YVjlNHQYs9w==" },
{ "something to sign MD5/64", "rV/ZLc5nCerkL+p8URqmVg==" },
{ "another thing to sign MD5/64", "W6wfT321SH/YVjlNHQYs9w==" },
};
// @formatter:on
}
/**
* @return a pair message/signature (HMAC using SHA256 encoded in hexadecimal).
*/
public static String[][] hmacSha256Base16() {
// @formatter:off
return new String[][] {
{ "something to sign SHA256/16", "c94011f098e4351e77cfdd317d5f0f29d2697abf4ae52c91e5fc1aeaac7dbacc" },
{ "another thing to sign SHA256/16", "a67d2c56cd9f7ef1f0e1bd8c67ba688d27dbb1fe4accb86cdbfc813feec8659d" },
{ "something to sign SHA256/16", "c94011f098e4351e77cfdd317d5f0f29d2697abf4ae52c91e5fc1aeaac7dbacc" },
{ "another thing to sign SHA256/16", "a67d2c56cd9f7ef1f0e1bd8c67ba688d27dbb1fe4accb86cdbfc813feec8659d" },
};
// @formatter:on
}
/**
* @return a pair message/signature (HMAC using SHA256 encoded in base 64).
*/
public static String[][] hmacSha256Base64() {
// @formatter:off
return new String[][] {
{ "something to sign SHA256/64", "Ljqk7O9pqM/K9meMcOgo6bJZe4dPE6UWnaRfcU6P2jA=" },
{ "another thing to sign SHA256/64", "ld8Iguof3vAG+2BVOnXlR6iSCQb6sI0Vjyv1jOBtisQ=" },
{ "something to sign SHA256/64", "Ljqk7O9pqM/K9meMcOgo6bJZe4dPE6UWnaRfcU6P2jA=" },
{ "another thing to sign SHA256/64", "ld8Iguof3vAG+2BVOnXlR6iSCQb6sI0Vjyv1jOBtisQ=" },
};
// @formatter:on
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy