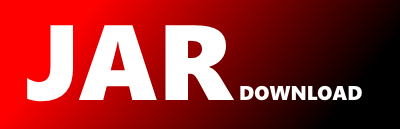
name.didier.david.test4j.utils.JsonUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ndd-test4j Show documentation
Show all versions of ndd-test4j Show documentation
Test4J provides testing support.
The newest version!
package name.didier.david.test4j.utils;
import java.io.IOException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
/**
* Utilities dealing with JSON.
*
* @author ddidier
*/
public final class JsonUtils {
/** Serialize JSON. */
private static final ObjectMapper MAPPER = new ObjectMapper();
/** Serialize JSON with pretty print. */
private static final ObjectMapper MAPPER_PP = new ObjectMapper().enable(SerializationFeature.INDENT_OUTPUT);
/** Default constructor. */
private JsonUtils() {
super();
}
/**
* Join the elements and replace all custom quotes with double quotes. Useful to embed JSON strings in code since
* Java doesn't support hereDoc. For example:
*
*
* json('_', "{_id_: 123, _name_: _a cool name_}"
*
* OR
*
* json('_',
* "{",
* " _id_: 123,",
* " _name_: _a cool name_",
* "}")
*
*
* @param doubleQuotes the character to replace with single quote.
* @param elements the strings to join.
* @return the JSON string.
*/
public static String json(char doubleQuotes, CharSequence... elements) {
return String.join("\n", elements).replace(doubleQuotes, '"');
}
/**
* Join the elements and replace all simple quotes with double quotes. Useful to embed JSON strings in code since
* Java doesn't support hereDoc. For example:
*
*
* json("{'id': 123, 'name': 'a cool name'}"
*
* OR
*
* json(
* "{",
* " 'id': 123,",
* " 'name': 'a cool name'",
* "}")
*
*
* @param elements the strings to join.
* @return the JSON string.
*/
public static String json(CharSequence... elements) {
return json('\'', elements);
}
// -----------------------------------------------------------------------------------------------------------------
/**
* Normalize the given JSON string.
*
* @param json the JSON to normalize.
* @return the normalized JSON string.
*/
public static String normalize(String json) {
return parseJson(json, MAPPER);
}
/**
* Normalize the given JSON string resulting of {@link #json(CharSequence...)}.
*
* @param elements the JSON to join then normalize.
* @return the normalized JSON string.
*/
public static String normalizeJson(CharSequence... elements) {
return normalize(json(elements));
}
/**
* Normalize and pretty print the given JSON string.
*
* @param json the JSON to pretty print.
* @return the pretty printed JSON string.
*/
public static String prettyPrint(String json) {
return parseJson(json, MAPPER_PP);
}
/**
* Normalize and pretty print the given JSON string resulting of {@link #json(CharSequence...)}.
*
* @param elements the JSON to join then normalize.
* @return the pretty printed JSON string.
*/
public static String prettyPrintJson(CharSequence... elements) {
return prettyPrint(json(elements));
}
/**
* Normalize the given JSON string.
*
* @param json the JSON to normalize.
* @param mapper the JSON parser.
* @return the normalized JSON string.
*/
private static String parseJson(String json, ObjectMapper mapper) {
try {
return mapper.writeValueAsString(mapper.readValue(json, Object.class));
} catch (IOException e) {
throw new RuntimeException("Error while parsing JSON", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy