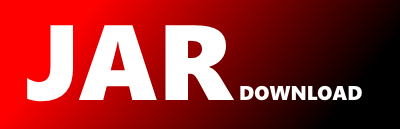
name.kevinlocke.appveyor.api.ProjectApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appveyor-swagger Show documentation
Show all versions of appveyor-swagger Show documentation
AppVeyor REST API Client generated from the unofficial Swagger API
definition in Java.
The newest version!
/*
* AppVeyor REST API
* AppVeyor is a hosted continuous integration service which runs on Microsoft Windows. The AppVeyor REST API provides a RESTful way to interact with the AppVeyor service. This includes managing projects, builds, deployments, and the teams that build them. Additional help and discussion of the AppVeyor REST API is available at http://help.appveyor.com/discussions This Swagger definition is an **unofficial** description of the AppVeyor REST API maintained at https://github.com/kevinoid/appveyor-swagger Please report any issues or suggestions for this Swagger definition at https://github.com/kevinoid/appveyor-swagger/issues/new #### API Conventions Fields which are missing from update operations (`PUT` requests) are typically reset to their default values. So although most fields are not technically required, they should usually be specified in practice.
*
* OpenAPI spec version: 0.20170106.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package name.kevinlocke.appveyor.api;
import name.kevinlocke.appveyor.ApiCallback;
import name.kevinlocke.appveyor.ApiClient;
import name.kevinlocke.appveyor.ApiException;
import name.kevinlocke.appveyor.ApiResponse;
import name.kevinlocke.appveyor.Configuration;
import name.kevinlocke.appveyor.Pair;
import name.kevinlocke.appveyor.ProgressRequestBody;
import name.kevinlocke.appveyor.ProgressResponseBody;
import name.kevinlocke.appveyor.BeanValidationException;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import javax.validation.constraints.*;
import javax.validation.ConstraintViolation;
import javax.validation.Validation;
import javax.validation.ValidatorFactory;
import javax.validation.executable.ExecutableValidator;
import java.util.Set;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import name.kevinlocke.appveyor.model.EncryptRequest;
import name.kevinlocke.appveyor.model.Error;
import java.io.File;
import name.kevinlocke.appveyor.model.Project;
import name.kevinlocke.appveyor.model.ProjectAddition;
import name.kevinlocke.appveyor.model.ProjectBuildNumberUpdate;
import name.kevinlocke.appveyor.model.ProjectBuildResults;
import name.kevinlocke.appveyor.model.ProjectDeploymentsResults;
import name.kevinlocke.appveyor.model.ProjectHistory;
import name.kevinlocke.appveyor.model.ProjectSettingsResults;
import name.kevinlocke.appveyor.model.ProjectWithConfiguration;
import name.kevinlocke.appveyor.model.StoredNameValue;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ProjectApi {
private ApiClient apiClient;
public ProjectApi() {
this(Configuration.getDefaultApiClient());
}
public ProjectApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/* Build call for addProject */
private com.squareup.okhttp.Call addProjectCall(ProjectAddition body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/projects".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call addProjectValidateBeforeCall(ProjectAddition body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { body };
Method method = this.getClass().getMethod("addProjectWithHttpInfo", ProjectAddition.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = addProjectCall(body, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Add project
*
* @param body (required)
* @return Project
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public Project addProject(ProjectAddition body) throws ApiException {
ApiResponse resp = addProjectWithHttpInfo(body);
return resp.getData();
}
/**
* Add project
*
* @param body (required)
* @return ApiResponse<Project>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse addProjectWithHttpInfo( @NotNull ProjectAddition body) throws ApiException {
com.squareup.okhttp.Call call = addProjectValidateBeforeCall(body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add project (asynchronously)
*
* @param body (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call addProjectAsync(ProjectAddition body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = addProjectValidateBeforeCall(body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for deleteProject */
private com.squareup.okhttp.Call deleteProjectCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deleteProjectValidateBeforeCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug };
Method method = this.getClass().getMethod("deleteProjectWithHttpInfo", String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = deleteProjectCall(accountName, projectSlug, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Delete project
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteProject(String accountName, String projectSlug) throws ApiException {
deleteProjectWithHttpInfo(accountName, projectSlug);
}
/**
* Delete project
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteProjectWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug) throws ApiException {
com.squareup.okhttp.Call call = deleteProjectValidateBeforeCall(accountName, projectSlug, null, null);
return apiClient.execute(call);
}
/**
* Delete project (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deleteProjectAsync(String accountName, String projectSlug, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deleteProjectValidateBeforeCall(accountName, projectSlug, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for deleteProjectBuildCache */
private com.squareup.okhttp.Call deleteProjectBuildCacheCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/buildcache".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deleteProjectBuildCacheValidateBeforeCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug };
Method method = this.getClass().getMethod("deleteProjectBuildCacheWithHttpInfo", String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = deleteProjectBuildCacheCall(accountName, projectSlug, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Delete project build cache
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteProjectBuildCache(String accountName, String projectSlug) throws ApiException {
deleteProjectBuildCacheWithHttpInfo(accountName, projectSlug);
}
/**
* Delete project build cache
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteProjectBuildCacheWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug) throws ApiException {
com.squareup.okhttp.Call call = deleteProjectBuildCacheValidateBeforeCall(accountName, projectSlug, null, null);
return apiClient.execute(call);
}
/**
* Delete project build cache (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deleteProjectBuildCacheAsync(String accountName, String projectSlug, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deleteProjectBuildCacheValidateBeforeCall(accountName, projectSlug, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for encryptValue */
private com.squareup.okhttp.Call encryptValueCall(EncryptRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/account/encrypt".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call encryptValueValidateBeforeCall(EncryptRequest body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { body };
Method method = this.getClass().getMethod("encryptValueWithHttpInfo", EncryptRequest.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = encryptValueCall(body, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Encrypt a value for use in StoredValue.
*
* @param body (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String encryptValue(EncryptRequest body) throws ApiException {
ApiResponse resp = encryptValueWithHttpInfo(body);
return resp.getData();
}
/**
* Encrypt a value for use in StoredValue.
*
* @param body (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse encryptValueWithHttpInfo( @NotNull EncryptRequest body) throws ApiException {
com.squareup.okhttp.Call call = encryptValueValidateBeforeCall(body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Encrypt a value for use in StoredValue. (asynchronously)
*
* @param body (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call encryptValueAsync(EncryptRequest body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = encryptValueValidateBeforeCall(body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectArtifact */
private com.squareup.okhttp.Call getProjectArtifactCall(String accountName, String projectSlug, String artifactFileName, String branch, String tag, String job, Boolean all, Boolean pr, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/artifacts/{artifactFileName}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()))
.replaceAll("\\{" + "artifactFileName" + "\\}", apiClient.escapeString(artifactFileName.toString()));
List localVarQueryParams = new ArrayList();
if (branch != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "branch", branch));
if (tag != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "tag", tag));
if (job != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "job", job));
if (all != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "all", all));
if (pr != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "pr", pr));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/octet-stream"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectArtifactValidateBeforeCall(String accountName, String projectSlug, String artifactFileName, String branch, String tag, String job, Boolean all, Boolean pr, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug, artifactFileName, branch, tag, job, all, pr };
Method method = this.getClass().getMethod("getProjectArtifactWithHttpInfo", String.class, String.class, String.class, String.class, String.class, String.class, Boolean.class, Boolean.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectArtifactCall(accountName, projectSlug, artifactFileName, branch, tag, job, all, pr, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get last successful build artifact
* The `job` parameter is mandatory if the build contains multiple jobs.
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param artifactFileName File name (or path) of a build artifact file. Corresponds to the `fileName` property of `ArtifactModel`. URL-encoding of slashes in parameter values is optional. (required)
* @param branch Repository Branch (optional)
* @param tag A git (or other VCS) tag (optional)
* @param job Name of the build job. (optional)
* @param all Include not only `successful`, but also jobs with `failed`, and `cancelled` status. (optional, default to false)
* @param pr Include PR builds in the search results? `true` - take artifact from PR builds only; `false` - do not look for artifact in PR builds; default/unspecified - look for artifact in both PR an non-PR builds. (optional)
* @return File
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public File getProjectArtifact(String accountName, String projectSlug, String artifactFileName, String branch, String tag, String job, Boolean all, Boolean pr) throws ApiException {
ApiResponse resp = getProjectArtifactWithHttpInfo(accountName, projectSlug, artifactFileName, branch, tag, job, all, pr);
return resp.getData();
}
/**
* Get last successful build artifact
* The `job` parameter is mandatory if the build contains multiple jobs.
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param artifactFileName File name (or path) of a build artifact file. Corresponds to the `fileName` property of `ArtifactModel`. URL-encoding of slashes in parameter values is optional. (required)
* @param branch Repository Branch (optional)
* @param tag A git (or other VCS) tag (optional)
* @param job Name of the build job. (optional)
* @param all Include not only `successful`, but also jobs with `failed`, and `cancelled` status. (optional, default to false)
* @param pr Include PR builds in the search results? `true` - take artifact from PR builds only; `false` - do not look for artifact in PR builds; default/unspecified - look for artifact in both PR an non-PR builds. (optional)
* @return ApiResponse<File>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectArtifactWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug, @NotNull @Size(min=1) String artifactFileName, @Size(min=1) String branch, @Size(min=1) String tag, String job, Boolean all, Boolean pr) throws ApiException {
com.squareup.okhttp.Call call = getProjectArtifactValidateBeforeCall(accountName, projectSlug, artifactFileName, branch, tag, job, all, pr, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get last successful build artifact (asynchronously)
* The `job` parameter is mandatory if the build contains multiple jobs.
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param artifactFileName File name (or path) of a build artifact file. Corresponds to the `fileName` property of `ArtifactModel`. URL-encoding of slashes in parameter values is optional. (required)
* @param branch Repository Branch (optional)
* @param tag A git (or other VCS) tag (optional)
* @param job Name of the build job. (optional)
* @param all Include not only `successful`, but also jobs with `failed`, and `cancelled` status. (optional, default to false)
* @param pr Include PR builds in the search results? `true` - take artifact from PR builds only; `false` - do not look for artifact in PR builds; default/unspecified - look for artifact in both PR an non-PR builds. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectArtifactAsync(String accountName, String projectSlug, String artifactFileName, String branch, String tag, String job, Boolean all, Boolean pr, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectArtifactValidateBeforeCall(accountName, projectSlug, artifactFileName, branch, tag, job, all, pr, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectBranchStatusBadge */
private com.squareup.okhttp.Call getProjectBranchStatusBadgeCall(String statusBadgeId, String buildBranch, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/status/{statusBadgeId}/branch/{buildBranch}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "statusBadgeId" + "\\}", apiClient.escapeString(statusBadgeId.toString()))
.replaceAll("\\{" + "buildBranch" + "\\}", apiClient.escapeString(buildBranch.toString()));
List localVarQueryParams = new ArrayList();
if (svg != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "svg", svg));
if (retina != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "retina", retina));
if (passingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "passingText", passingText));
if (failingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "failingText", failingText));
if (pendingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "pendingText", pendingText));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"image/svg+xml", "image/png"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectBranchStatusBadgeValidateBeforeCall(String statusBadgeId, String buildBranch, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { statusBadgeId, buildBranch, svg, retina, passingText, failingText, pendingText };
Method method = this.getClass().getMethod("getProjectBranchStatusBadgeWithHttpInfo", String.class, String.class, Boolean.class, Boolean.class, String.class, String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectBranchStatusBadgeCall(statusBadgeId, buildBranch, svg, retina, passingText, failingText, pendingText, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project branch status badge image
*
* @param statusBadgeId ID of the status badge (`statusBadgeId` from `ProjectWithConfiguration`). (required)
* @param buildBranch Build Branch (required)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @return File
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public File getProjectBranchStatusBadge(String statusBadgeId, String buildBranch, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText) throws ApiException {
ApiResponse resp = getProjectBranchStatusBadgeWithHttpInfo(statusBadgeId, buildBranch, svg, retina, passingText, failingText, pendingText);
return resp.getData();
}
/**
* Get project branch status badge image
*
* @param statusBadgeId ID of the status badge (`statusBadgeId` from `ProjectWithConfiguration`). (required)
* @param buildBranch Build Branch (required)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @return ApiResponse<File>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectBranchStatusBadgeWithHttpInfo( @NotNull @Size(min=1) String statusBadgeId, @NotNull String buildBranch, Boolean svg, Boolean retina, @Size(min=1) String passingText, @Size(min=1) String failingText, @Size(min=1) String pendingText) throws ApiException {
com.squareup.okhttp.Call call = getProjectBranchStatusBadgeValidateBeforeCall(statusBadgeId, buildBranch, svg, retina, passingText, failingText, pendingText, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project branch status badge image (asynchronously)
*
* @param statusBadgeId ID of the status badge (`statusBadgeId` from `ProjectWithConfiguration`). (required)
* @param buildBranch Build Branch (required)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectBranchStatusBadgeAsync(String statusBadgeId, String buildBranch, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectBranchStatusBadgeValidateBeforeCall(statusBadgeId, buildBranch, svg, retina, passingText, failingText, pendingText, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectBuildByVersion */
private com.squareup.okhttp.Call getProjectBuildByVersionCall(String accountName, String projectSlug, String buildVersion, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/build/{buildVersion}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()))
.replaceAll("\\{" + "buildVersion" + "\\}", apiClient.escapeString(buildVersion.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectBuildByVersionValidateBeforeCall(String accountName, String projectSlug, String buildVersion, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug, buildVersion };
Method method = this.getClass().getMethod("getProjectBuildByVersionWithHttpInfo", String.class, String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectBuildByVersionCall(accountName, projectSlug, buildVersion, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project build by version
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param buildVersion Build Version (`version` property of `Build`) (required)
* @return ProjectBuildResults
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ProjectBuildResults getProjectBuildByVersion(String accountName, String projectSlug, String buildVersion) throws ApiException {
ApiResponse resp = getProjectBuildByVersionWithHttpInfo(accountName, projectSlug, buildVersion);
return resp.getData();
}
/**
* Get project build by version
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param buildVersion Build Version (`version` property of `Build`) (required)
* @return ApiResponse<ProjectBuildResults>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectBuildByVersionWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug, @NotNull String buildVersion) throws ApiException {
com.squareup.okhttp.Call call = getProjectBuildByVersionValidateBeforeCall(accountName, projectSlug, buildVersion, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project build by version (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param buildVersion Build Version (`version` property of `Build`) (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectBuildByVersionAsync(String accountName, String projectSlug, String buildVersion, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectBuildByVersionValidateBeforeCall(accountName, projectSlug, buildVersion, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectDeployments */
private com.squareup.okhttp.Call getProjectDeploymentsCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/deployments".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectDeploymentsValidateBeforeCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug };
Method method = this.getClass().getMethod("getProjectDeploymentsWithHttpInfo", String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectDeploymentsCall(accountName, projectSlug, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project deployments
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ProjectDeploymentsResults
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ProjectDeploymentsResults getProjectDeployments(String accountName, String projectSlug) throws ApiException {
ApiResponse resp = getProjectDeploymentsWithHttpInfo(accountName, projectSlug);
return resp.getData();
}
/**
* Get project deployments
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ApiResponse<ProjectDeploymentsResults>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectDeploymentsWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug) throws ApiException {
com.squareup.okhttp.Call call = getProjectDeploymentsValidateBeforeCall(accountName, projectSlug, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project deployments (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectDeploymentsAsync(String accountName, String projectSlug, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectDeploymentsValidateBeforeCall(accountName, projectSlug, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectEnvironmentVariables */
private com.squareup.okhttp.Call getProjectEnvironmentVariablesCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/settings/environment-variables".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectEnvironmentVariablesValidateBeforeCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug };
Method method = this.getClass().getMethod("getProjectEnvironmentVariablesWithHttpInfo", String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectEnvironmentVariablesCall(accountName, projectSlug, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project environment variables
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return List<StoredNameValue>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List getProjectEnvironmentVariables(String accountName, String projectSlug) throws ApiException {
ApiResponse> resp = getProjectEnvironmentVariablesWithHttpInfo(accountName, projectSlug);
return resp.getData();
}
/**
* Get project environment variables
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ApiResponse<List<StoredNameValue>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> getProjectEnvironmentVariablesWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug) throws ApiException {
com.squareup.okhttp.Call call = getProjectEnvironmentVariablesValidateBeforeCall(accountName, projectSlug, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project environment variables (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectEnvironmentVariablesAsync(String accountName, String projectSlug, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectEnvironmentVariablesValidateBeforeCall(accountName, projectSlug, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectHistory */
private com.squareup.okhttp.Call getProjectHistoryCall(String accountName, String projectSlug, Integer recordsNumber, Integer startBuildId, String branch, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/history".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
if (recordsNumber != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "recordsNumber", recordsNumber));
if (startBuildId != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "startBuildId", startBuildId));
if (branch != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "branch", branch));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectHistoryValidateBeforeCall(String accountName, String projectSlug, Integer recordsNumber, Integer startBuildId, String branch, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug, recordsNumber, startBuildId, branch };
Method method = this.getClass().getMethod("getProjectHistoryWithHttpInfo", String.class, String.class, Integer.class, Integer.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectHistoryCall(accountName, projectSlug, recordsNumber, startBuildId, branch, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project history
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param recordsNumber Number of results to include in the response. (required)
* @param startBuildId Maximum `buildId` to include in the results (exclusive). (optional)
* @param branch Repository Branch (optional)
* @return ProjectHistory
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ProjectHistory getProjectHistory(String accountName, String projectSlug, Integer recordsNumber, Integer startBuildId, String branch) throws ApiException {
ApiResponse resp = getProjectHistoryWithHttpInfo(accountName, projectSlug, recordsNumber, startBuildId, branch);
return resp.getData();
}
/**
* Get project history
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param recordsNumber Number of results to include in the response. (required)
* @param startBuildId Maximum `buildId` to include in the results (exclusive). (optional)
* @param branch Repository Branch (optional)
* @return ApiResponse<ProjectHistory>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectHistoryWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug, @NotNull/* @Min(0) */ Integer recordsNumber, /* @Min(0) */ Integer startBuildId, @Size(min=1) String branch) throws ApiException {
com.squareup.okhttp.Call call = getProjectHistoryValidateBeforeCall(accountName, projectSlug, recordsNumber, startBuildId, branch, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project history (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param recordsNumber Number of results to include in the response. (required)
* @param startBuildId Maximum `buildId` to include in the results (exclusive). (optional)
* @param branch Repository Branch (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectHistoryAsync(String accountName, String projectSlug, Integer recordsNumber, Integer startBuildId, String branch, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectHistoryValidateBeforeCall(accountName, projectSlug, recordsNumber, startBuildId, branch, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectLastBuild */
private com.squareup.okhttp.Call getProjectLastBuildCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectLastBuildValidateBeforeCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug };
Method method = this.getClass().getMethod("getProjectLastBuildWithHttpInfo", String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectLastBuildCall(accountName, projectSlug, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project last build
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ProjectBuildResults
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ProjectBuildResults getProjectLastBuild(String accountName, String projectSlug) throws ApiException {
ApiResponse resp = getProjectLastBuildWithHttpInfo(accountName, projectSlug);
return resp.getData();
}
/**
* Get project last build
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ApiResponse<ProjectBuildResults>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectLastBuildWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug) throws ApiException {
com.squareup.okhttp.Call call = getProjectLastBuildValidateBeforeCall(accountName, projectSlug, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project last build (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectLastBuildAsync(String accountName, String projectSlug, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectLastBuildValidateBeforeCall(accountName, projectSlug, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectLastBuildBranch */
private com.squareup.okhttp.Call getProjectLastBuildBranchCall(String accountName, String projectSlug, String buildBranch, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/branch/{buildBranch}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()))
.replaceAll("\\{" + "buildBranch" + "\\}", apiClient.escapeString(buildBranch.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectLastBuildBranchValidateBeforeCall(String accountName, String projectSlug, String buildBranch, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug, buildBranch };
Method method = this.getClass().getMethod("getProjectLastBuildBranchWithHttpInfo", String.class, String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectLastBuildBranchCall(accountName, projectSlug, buildBranch, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project last branch build
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param buildBranch Build Branch (required)
* @return ProjectBuildResults
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ProjectBuildResults getProjectLastBuildBranch(String accountName, String projectSlug, String buildBranch) throws ApiException {
ApiResponse resp = getProjectLastBuildBranchWithHttpInfo(accountName, projectSlug, buildBranch);
return resp.getData();
}
/**
* Get project last branch build
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param buildBranch Build Branch (required)
* @return ApiResponse<ProjectBuildResults>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectLastBuildBranchWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug, @NotNull String buildBranch) throws ApiException {
com.squareup.okhttp.Call call = getProjectLastBuildBranchValidateBeforeCall(accountName, projectSlug, buildBranch, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project last branch build (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param buildBranch Build Branch (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectLastBuildBranchAsync(String accountName, String projectSlug, String buildBranch, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectLastBuildBranchValidateBeforeCall(accountName, projectSlug, buildBranch, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectSettings */
private com.squareup.okhttp.Call getProjectSettingsCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/settings".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectSettingsValidateBeforeCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug };
Method method = this.getClass().getMethod("getProjectSettingsWithHttpInfo", String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectSettingsCall(accountName, projectSlug, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project settings
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ProjectSettingsResults
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ProjectSettingsResults getProjectSettings(String accountName, String projectSlug) throws ApiException {
ApiResponse resp = getProjectSettingsWithHttpInfo(accountName, projectSlug);
return resp.getData();
}
/**
* Get project settings
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ApiResponse<ProjectSettingsResults>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectSettingsWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug) throws ApiException {
com.squareup.okhttp.Call call = getProjectSettingsValidateBeforeCall(accountName, projectSlug, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project settings (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectSettingsAsync(String accountName, String projectSlug, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectSettingsValidateBeforeCall(accountName, projectSlug, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectSettingsYaml */
private com.squareup.okhttp.Call getProjectSettingsYamlCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/settings/yaml".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectSettingsYamlValidateBeforeCall(String accountName, String projectSlug, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug };
Method method = this.getClass().getMethod("getProjectSettingsYamlWithHttpInfo", String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectSettingsYamlCall(accountName, projectSlug, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project settings in YAML
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String getProjectSettingsYaml(String accountName, String projectSlug) throws ApiException {
ApiResponse resp = getProjectSettingsYamlWithHttpInfo(accountName, projectSlug);
return resp.getData();
}
/**
* Get project settings in YAML
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectSettingsYamlWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug) throws ApiException {
com.squareup.okhttp.Call call = getProjectSettingsYamlValidateBeforeCall(accountName, projectSlug, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project settings in YAML (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectSettingsYamlAsync(String accountName, String projectSlug, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectSettingsYamlValidateBeforeCall(accountName, projectSlug, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjectStatusBadge */
private com.squareup.okhttp.Call getProjectStatusBadgeCall(String statusBadgeId, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/status/{statusBadgeId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "statusBadgeId" + "\\}", apiClient.escapeString(statusBadgeId.toString()));
List localVarQueryParams = new ArrayList();
if (svg != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "svg", svg));
if (retina != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "retina", retina));
if (passingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "passingText", passingText));
if (failingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "failingText", failingText));
if (pendingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "pendingText", pendingText));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"image/svg+xml", "image/png"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectStatusBadgeValidateBeforeCall(String statusBadgeId, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { statusBadgeId, svg, retina, passingText, failingText, pendingText };
Method method = this.getClass().getMethod("getProjectStatusBadgeWithHttpInfo", String.class, Boolean.class, Boolean.class, String.class, String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectStatusBadgeCall(statusBadgeId, svg, retina, passingText, failingText, pendingText, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get project status badge image
*
* @param statusBadgeId ID of the status badge (`statusBadgeId` from `ProjectWithConfiguration`). (required)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @return File
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public File getProjectStatusBadge(String statusBadgeId, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText) throws ApiException {
ApiResponse resp = getProjectStatusBadgeWithHttpInfo(statusBadgeId, svg, retina, passingText, failingText, pendingText);
return resp.getData();
}
/**
* Get project status badge image
*
* @param statusBadgeId ID of the status badge (`statusBadgeId` from `ProjectWithConfiguration`). (required)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @return ApiResponse<File>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getProjectStatusBadgeWithHttpInfo( @NotNull @Size(min=1) String statusBadgeId, Boolean svg, Boolean retina, @Size(min=1) String passingText, @Size(min=1) String failingText, @Size(min=1) String pendingText) throws ApiException {
com.squareup.okhttp.Call call = getProjectStatusBadgeValidateBeforeCall(statusBadgeId, svg, retina, passingText, failingText, pendingText, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get project status badge image (asynchronously)
*
* @param statusBadgeId ID of the status badge (`statusBadgeId` from `ProjectWithConfiguration`). (required)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectStatusBadgeAsync(String statusBadgeId, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectStatusBadgeValidateBeforeCall(statusBadgeId, svg, retina, passingText, failingText, pendingText, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getProjects */
private com.squareup.okhttp.Call getProjectsCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getProjectsValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { };
Method method = this.getClass().getMethod("getProjectsWithHttpInfo");
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getProjectsCall(progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get projects
*
* @return List<Project>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List getProjects() throws ApiException {
ApiResponse> resp = getProjectsWithHttpInfo();
return resp.getData();
}
/**
* Get projects
*
* @return ApiResponse<List<Project>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> getProjectsWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = getProjectsValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get projects (asynchronously)
*
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getProjectsAsync(final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getProjectsValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getPublicProjectStatusBadge */
private com.squareup.okhttp.Call getPublicProjectStatusBadgeCall(String badgeRepoProvider, String repoAccountName, String repoSlug, String branch, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/projects/status/{badgeRepoProvider}/{repoAccountName}/{repoSlug}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "badgeRepoProvider" + "\\}", apiClient.escapeString(badgeRepoProvider.toString()))
.replaceAll("\\{" + "repoAccountName" + "\\}", apiClient.escapeString(repoAccountName.toString()))
.replaceAll("\\{" + "repoSlug" + "\\}", apiClient.escapeString(repoSlug.toString()));
List localVarQueryParams = new ArrayList();
if (branch != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "branch", branch));
if (svg != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "svg", svg));
if (retina != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "retina", retina));
if (passingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "passingText", passingText));
if (failingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "failingText", failingText));
if (pendingText != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "pendingText", pendingText));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"image/svg+xml", "image/png"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getPublicProjectStatusBadgeValidateBeforeCall(String badgeRepoProvider, String repoAccountName, String repoSlug, String branch, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { badgeRepoProvider, repoAccountName, repoSlug, branch, svg, retina, passingText, failingText, pendingText };
Method method = this.getClass().getMethod("getPublicProjectStatusBadgeWithHttpInfo", String.class, String.class, String.class, String.class, Boolean.class, Boolean.class, String.class, String.class, String.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = getPublicProjectStatusBadgeCall(badgeRepoProvider, repoAccountName, repoSlug, branch, svg, retina, passingText, failingText, pendingText, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Get status badge image for a project with a public repository
*
* @param badgeRepoProvider Repository provider supported for badges (required)
* @param repoAccountName Account name with repository provider (required)
* @param repoSlug Slug (URL component) of repository. (required)
* @param branch Repository Branch (optional)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @return File
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public File getPublicProjectStatusBadge(String badgeRepoProvider, String repoAccountName, String repoSlug, String branch, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText) throws ApiException {
ApiResponse resp = getPublicProjectStatusBadgeWithHttpInfo(badgeRepoProvider, repoAccountName, repoSlug, branch, svg, retina, passingText, failingText, pendingText);
return resp.getData();
}
/**
* Get status badge image for a project with a public repository
*
* @param badgeRepoProvider Repository provider supported for badges (required)
* @param repoAccountName Account name with repository provider (required)
* @param repoSlug Slug (URL component) of repository. (required)
* @param branch Repository Branch (optional)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @return ApiResponse<File>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getPublicProjectStatusBadgeWithHttpInfo( @NotNull String badgeRepoProvider, @NotNull @Size(min=1) String repoAccountName, @NotNull @Size(min=1) String repoSlug, @Size(min=1) String branch, Boolean svg, Boolean retina, @Size(min=1) String passingText, @Size(min=1) String failingText, @Size(min=1) String pendingText) throws ApiException {
com.squareup.okhttp.Call call = getPublicProjectStatusBadgeValidateBeforeCall(badgeRepoProvider, repoAccountName, repoSlug, branch, svg, retina, passingText, failingText, pendingText, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get status badge image for a project with a public repository (asynchronously)
*
* @param badgeRepoProvider Repository provider supported for badges (required)
* @param repoAccountName Account name with repository provider (required)
* @param repoSlug Slug (URL component) of repository. (required)
* @param branch Repository Branch (optional)
* @param svg Return an SVG image instead of PNG? Exclusive with `retina`. (optional, default to false)
* @param retina Return a larger image suitable for retina displays? Exclusive with `svg`. (optional, default to false)
* @param passingText Text to show in badge when build is passing. (optional)
* @param failingText Text to show in badge when build is failing. (optional)
* @param pendingText Text to show in badge when build is pending. (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getPublicProjectStatusBadgeAsync(String badgeRepoProvider, String repoAccountName, String repoSlug, String branch, Boolean svg, Boolean retina, String passingText, String failingText, String pendingText, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getPublicProjectStatusBadgeValidateBeforeCall(badgeRepoProvider, repoAccountName, repoSlug, branch, svg, retina, passingText, failingText, pendingText, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for updateProject */
private com.squareup.okhttp.Call updateProjectCall(ProjectWithConfiguration body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/projects".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateProjectValidateBeforeCall(ProjectWithConfiguration body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { body };
Method method = this.getClass().getMethod("updateProjectWithHttpInfo", ProjectWithConfiguration.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = updateProjectCall(body, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Update project
*
* @param body (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateProject(ProjectWithConfiguration body) throws ApiException {
updateProjectWithHttpInfo(body);
}
/**
* Update project
*
* @param body (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateProjectWithHttpInfo( @NotNull ProjectWithConfiguration body) throws ApiException {
com.squareup.okhttp.Call call = updateProjectValidateBeforeCall(body, null, null);
return apiClient.execute(call);
}
/**
* Update project (asynchronously)
*
* @param body (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateProjectAsync(ProjectWithConfiguration body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateProjectValidateBeforeCall(body, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for updateProjectBuildNumber */
private com.squareup.okhttp.Call updateProjectBuildNumberCall(String accountName, String projectSlug, ProjectBuildNumberUpdate body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/settings/build-number".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateProjectBuildNumberValidateBeforeCall(String accountName, String projectSlug, ProjectBuildNumberUpdate body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug, body };
Method method = this.getClass().getMethod("updateProjectBuildNumberWithHttpInfo", String.class, String.class, ProjectBuildNumberUpdate.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = updateProjectBuildNumberCall(accountName, projectSlug, body, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Update project build number
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateProjectBuildNumber(String accountName, String projectSlug, ProjectBuildNumberUpdate body) throws ApiException {
updateProjectBuildNumberWithHttpInfo(accountName, projectSlug, body);
}
/**
* Update project build number
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateProjectBuildNumberWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug, @NotNull ProjectBuildNumberUpdate body) throws ApiException {
com.squareup.okhttp.Call call = updateProjectBuildNumberValidateBeforeCall(accountName, projectSlug, body, null, null);
return apiClient.execute(call);
}
/**
* Update project build number (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateProjectBuildNumberAsync(String accountName, String projectSlug, ProjectBuildNumberUpdate body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateProjectBuildNumberValidateBeforeCall(accountName, projectSlug, body, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for updateProjectEnvironmentVariables */
private com.squareup.okhttp.Call updateProjectEnvironmentVariablesCall(String accountName, String projectSlug, List body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/settings/environment-variables".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateProjectEnvironmentVariablesValidateBeforeCall(String accountName, String projectSlug, List body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug, body };
Method method = this.getClass().getMethod("updateProjectEnvironmentVariablesWithHttpInfo", String.class, String.class, java.util.List.class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = updateProjectEnvironmentVariablesCall(accountName, projectSlug, body, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Update project environment variables
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateProjectEnvironmentVariables(String accountName, String projectSlug, List body) throws ApiException {
updateProjectEnvironmentVariablesWithHttpInfo(accountName, projectSlug, body);
}
/**
* Update project environment variables
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateProjectEnvironmentVariablesWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug, @NotNull List body) throws ApiException {
com.squareup.okhttp.Call call = updateProjectEnvironmentVariablesValidateBeforeCall(accountName, projectSlug, body, null, null);
return apiClient.execute(call);
}
/**
* Update project environment variables (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateProjectEnvironmentVariablesAsync(String accountName, String projectSlug, List body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateProjectEnvironmentVariablesValidateBeforeCall(accountName, projectSlug, body, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for updateProjectSettingsYaml */
private com.squareup.okhttp.Call updateProjectSettingsYamlCall(String accountName, String projectSlug, byte[] body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/projects/{accountName}/{projectSlug}/settings/yaml".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "accountName" + "\\}", apiClient.escapeString(accountName.toString()))
.replaceAll("\\{" + "projectSlug" + "\\}", apiClient.escapeString(projectSlug.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json", "application/xml"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"text/plain"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "apiToken" };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call updateProjectSettingsYamlValidateBeforeCall(String accountName, String projectSlug, byte[] body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
ExecutableValidator executableValidator = factory.getValidator().forExecutables();
Object[] parameterValues = { accountName, projectSlug, body };
Method method = this.getClass().getMethod("updateProjectSettingsYamlWithHttpInfo", String.class, String.class, byte[].class);
Set> violations = executableValidator.validateParameters(this, method,
parameterValues);
if (violations.size() == 0) {
com.squareup.okhttp.Call call = updateProjectSettingsYamlCall(accountName, projectSlug, body, progressListener, progressRequestListener);
return call;
} else {
throw new BeanValidationException((Set) violations);
}
} catch (NoSuchMethodException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
} catch (SecurityException e) {
e.printStackTrace();
throw new ApiException(e.getMessage());
}
}
/**
* Update project settings in YAML
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body The body of requests should contain YAML data. It is unclear how to specify this since the OpenAPI spec requires `schema` without `type` for `in: body` parameters and does not allow `type: file` in `schema`. See https://github.com/OAI/OpenAPI-Specification/issues/326 swagger-codegen (for Java, probably others) allows a binary string body parameter with non-application/json `consumes` to be passed through in the request body without conversion to JSON. (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void updateProjectSettingsYaml(String accountName, String projectSlug, byte[] body) throws ApiException {
updateProjectSettingsYamlWithHttpInfo(accountName, projectSlug, body);
}
/**
* Update project settings in YAML
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body The body of requests should contain YAML data. It is unclear how to specify this since the OpenAPI spec requires `schema` without `type` for `in: body` parameters and does not allow `type: file` in `schema`. See https://github.com/OAI/OpenAPI-Specification/issues/326 swagger-codegen (for Java, probably others) allows a binary string body parameter with non-application/json `consumes` to be passed through in the request body without conversion to JSON. (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse updateProjectSettingsYamlWithHttpInfo( @NotNull @Size(min=1) String accountName, @NotNull String projectSlug, @NotNull byte[] body) throws ApiException {
com.squareup.okhttp.Call call = updateProjectSettingsYamlValidateBeforeCall(accountName, projectSlug, body, null, null);
return apiClient.execute(call);
}
/**
* Update project settings in YAML (asynchronously)
*
* @param accountName AppVeyor account name (`accountName` property of `UserAccount`) (required)
* @param projectSlug Project Slug (required)
* @param body The body of requests should contain YAML data. It is unclear how to specify this since the OpenAPI spec requires `schema` without `type` for `in: body` parameters and does not allow `type: file` in `schema`. See https://github.com/OAI/OpenAPI-Specification/issues/326 swagger-codegen (for Java, probably others) allows a binary string body parameter with non-application/json `consumes` to be passed through in the request body without conversion to JSON. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call updateProjectSettingsYamlAsync(String accountName, String projectSlug, byte[] body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = updateProjectSettingsYamlValidateBeforeCall(accountName, projectSlug, body, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy