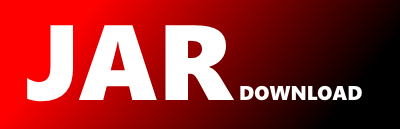
name.kevinlocke.appveyor.model.Build Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appveyor-swagger Show documentation
Show all versions of appveyor-swagger Show documentation
AppVeyor REST API Client generated from the unofficial Swagger API
definition in Java.
The newest version!
/*
* AppVeyor REST API
* AppVeyor is a hosted continuous integration service which runs on Microsoft Windows. The AppVeyor REST API provides a RESTful way to interact with the AppVeyor service. This includes managing projects, builds, deployments, and the teams that build them. Additional help and discussion of the AppVeyor REST API is available at http://help.appveyor.com/discussions This Swagger definition is an **unofficial** description of the AppVeyor REST API maintained at https://github.com/kevinoid/appveyor-swagger Please report any issues or suggestions for this Swagger definition at https://github.com/kevinoid/appveyor-swagger/issues/new #### API Conventions Fields which are missing from update operations (`PUT` requests) are typically reset to their default values. So although most fields are not technically required, they should usually be specified in practice.
*
* OpenAPI spec version: 0.20170106.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package name.kevinlocke.appveyor.model;
import java.util.Objects;
import com.google.gson.annotations.SerializedName;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import name.kevinlocke.appveyor.model.BuildJob;
import name.kevinlocke.appveyor.model.BuildLookupModel;
import name.kevinlocke.appveyor.model.BuildMessage;
import name.kevinlocke.appveyor.model.Status;
import name.kevinlocke.appveyor.model.Timestamped;
import org.joda.time.DateTime;
import javax.validation.constraints.*;
/**
* Build
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2017-11-23T14:03:51.855-07:00")
public class Build {
@SerializedName("branch")
private String branch = null;
@SerializedName("buildId")
private Integer buildId = null;
@SerializedName("message")
private String message = null;
@SerializedName("version")
private String version = null;
@SerializedName("created")
private DateTime created = null;
@SerializedName("updated")
private DateTime updated = null;
@SerializedName("jobs")
private List jobs = new ArrayList();
@SerializedName("buildNumber")
private Integer buildNumber = null;
@SerializedName("messageExtended")
private String messageExtended = null;
@SerializedName("isTag")
private Boolean isTag = null;
@SerializedName("commitId")
private String commitId = null;
@SerializedName("authorName")
private String authorName = null;
@SerializedName("authorUsername")
private String authorUsername = null;
@SerializedName("committerName")
private String committerName = null;
@SerializedName("committerUsername")
private String committerUsername = null;
@SerializedName("committed")
private DateTime committed = null;
@SerializedName("pullRequestId")
private Integer pullRequestId = null;
@SerializedName("pullRequestName")
private String pullRequestName = null;
@SerializedName("messages")
private List messages = new ArrayList();
@SerializedName("status")
private Status status = null;
@SerializedName("started")
private DateTime started = null;
@SerializedName("finished")
private DateTime finished = null;
public Build branch(String branch) {
this.branch = branch;
return this;
}
/**
* Get branch
* @return branch
**/
@ApiModelProperty(example = "null", value = "")
public String getBranch() {
return branch;
}
public void setBranch(String branch) {
this.branch = branch;
}
public Build buildId(Integer buildId) {
this.buildId = buildId;
return this;
}
/**
* Get buildId
* minimum: 0
* @return buildId
**/
@Min(0)
@ApiModelProperty(example = "null", value = "")
public Integer getBuildId() {
return buildId;
}
public void setBuildId(Integer buildId) {
this.buildId = buildId;
}
public Build message(String message) {
this.message = message;
return this;
}
/**
* Get message
* @return message
**/
@ApiModelProperty(example = "null", value = "")
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public Build version(String version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
**/
@ApiModelProperty(example = "null", value = "")
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
/**
* Get created
* @return created
**/
@ApiModelProperty(example = "null", value = "")
public DateTime getCreated() {
return created;
}
/**
* Get updated
* @return updated
**/
@ApiModelProperty(example = "null", value = "")
public DateTime getUpdated() {
return updated;
}
public Build jobs(List jobs) {
this.jobs = jobs;
return this;
}
public Build addJobsItem(BuildJob jobsItem) {
this.jobs.add(jobsItem);
return this;
}
/**
* Always empty in getProjectHistory and startDeployment responses.
* @return jobs
**/
@ApiModelProperty(example = "null", value = "Always empty in getProjectHistory and startDeployment responses.")
public List getJobs() {
return jobs;
}
public void setJobs(List jobs) {
this.jobs = jobs;
}
public Build buildNumber(Integer buildNumber) {
this.buildNumber = buildNumber;
return this;
}
/**
* Get buildNumber
* minimum: 0
* @return buildNumber
**/
@Min(0)
@ApiModelProperty(example = "null", value = "")
public Integer getBuildNumber() {
return buildNumber;
}
public void setBuildNumber(Integer buildNumber) {
this.buildNumber = buildNumber;
}
public Build messageExtended(String messageExtended) {
this.messageExtended = messageExtended;
return this;
}
/**
* Get messageExtended
* @return messageExtended
**/
@ApiModelProperty(example = "null", value = "")
public String getMessageExtended() {
return messageExtended;
}
public void setMessageExtended(String messageExtended) {
this.messageExtended = messageExtended;
}
public Build isTag(Boolean isTag) {
this.isTag = isTag;
return this;
}
/**
* Get isTag
* @return isTag
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getIsTag() {
return isTag;
}
public void setIsTag(Boolean isTag) {
this.isTag = isTag;
}
public Build commitId(String commitId) {
this.commitId = commitId;
return this;
}
/**
* Get commitId
* @return commitId
**/
@ApiModelProperty(example = "null", value = "")
public String getCommitId() {
return commitId;
}
public void setCommitId(String commitId) {
this.commitId = commitId;
}
public Build authorName(String authorName) {
this.authorName = authorName;
return this;
}
/**
* Get authorName
* @return authorName
**/
@ApiModelProperty(example = "null", value = "")
public String getAuthorName() {
return authorName;
}
public void setAuthorName(String authorName) {
this.authorName = authorName;
}
public Build authorUsername(String authorUsername) {
this.authorUsername = authorUsername;
return this;
}
/**
* Get authorUsername
* @return authorUsername
**/
@ApiModelProperty(example = "null", value = "")
public String getAuthorUsername() {
return authorUsername;
}
public void setAuthorUsername(String authorUsername) {
this.authorUsername = authorUsername;
}
public Build committerName(String committerName) {
this.committerName = committerName;
return this;
}
/**
* Get committerName
* @return committerName
**/
@ApiModelProperty(example = "null", value = "")
public String getCommitterName() {
return committerName;
}
public void setCommitterName(String committerName) {
this.committerName = committerName;
}
public Build committerUsername(String committerUsername) {
this.committerUsername = committerUsername;
return this;
}
/**
* Get committerUsername
* @return committerUsername
**/
@ApiModelProperty(example = "null", value = "")
public String getCommitterUsername() {
return committerUsername;
}
public void setCommitterUsername(String committerUsername) {
this.committerUsername = committerUsername;
}
public Build committed(DateTime committed) {
this.committed = committed;
return this;
}
/**
* Get committed
* @return committed
**/
@ApiModelProperty(example = "null", value = "")
public DateTime getCommitted() {
return committed;
}
public void setCommitted(DateTime committed) {
this.committed = committed;
}
public Build pullRequestId(Integer pullRequestId) {
this.pullRequestId = pullRequestId;
return this;
}
/**
* Get pullRequestId
* minimum: 1
* @return pullRequestId
**/
@Min(1)
@ApiModelProperty(example = "null", value = "")
public Integer getPullRequestId() {
return pullRequestId;
}
public void setPullRequestId(Integer pullRequestId) {
this.pullRequestId = pullRequestId;
}
public Build pullRequestName(String pullRequestName) {
this.pullRequestName = pullRequestName;
return this;
}
/**
* Get pullRequestName
* @return pullRequestName
**/
@ApiModelProperty(example = "null", value = "")
public String getPullRequestName() {
return pullRequestName;
}
public void setPullRequestName(String pullRequestName) {
this.pullRequestName = pullRequestName;
}
public Build messages(List messages) {
this.messages = messages;
return this;
}
public Build addMessagesItem(BuildMessage messagesItem) {
this.messages.add(messagesItem);
return this;
}
/**
* Get messages
* @return messages
**/
@ApiModelProperty(example = "null", value = "")
public List getMessages() {
return messages;
}
public void setMessages(List messages) {
this.messages = messages;
}
public Build status(Status status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@ApiModelProperty(example = "null", value = "")
public Status getStatus() {
return status;
}
public void setStatus(Status status) {
this.status = status;
}
public Build started(DateTime started) {
this.started = started;
return this;
}
/**
* Get started
* @return started
**/
@ApiModelProperty(example = "null", value = "")
public DateTime getStarted() {
return started;
}
public void setStarted(DateTime started) {
this.started = started;
}
public Build finished(DateTime finished) {
this.finished = finished;
return this;
}
/**
* Get finished
* @return finished
**/
@ApiModelProperty(example = "null", value = "")
public DateTime getFinished() {
return finished;
}
public void setFinished(DateTime finished) {
this.finished = finished;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Build build = (Build) o;
return Objects.equals(this.branch, build.branch) &&
Objects.equals(this.buildId, build.buildId) &&
Objects.equals(this.message, build.message) &&
Objects.equals(this.version, build.version) &&
Objects.equals(this.created, build.created) &&
Objects.equals(this.updated, build.updated) &&
Objects.equals(this.jobs, build.jobs) &&
Objects.equals(this.buildNumber, build.buildNumber) &&
Objects.equals(this.messageExtended, build.messageExtended) &&
Objects.equals(this.isTag, build.isTag) &&
Objects.equals(this.commitId, build.commitId) &&
Objects.equals(this.authorName, build.authorName) &&
Objects.equals(this.authorUsername, build.authorUsername) &&
Objects.equals(this.committerName, build.committerName) &&
Objects.equals(this.committerUsername, build.committerUsername) &&
Objects.equals(this.committed, build.committed) &&
Objects.equals(this.pullRequestId, build.pullRequestId) &&
Objects.equals(this.pullRequestName, build.pullRequestName) &&
Objects.equals(this.messages, build.messages) &&
Objects.equals(this.status, build.status) &&
Objects.equals(this.started, build.started) &&
Objects.equals(this.finished, build.finished);
}
@Override
public int hashCode() {
return Objects.hash(branch, buildId, message, version, created, updated, jobs, buildNumber, messageExtended, isTag, commitId, authorName, authorUsername, committerName, committerUsername, committed, pullRequestId, pullRequestName, messages, status, started, finished);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Build {\n");
sb.append(" branch: ").append(toIndentedString(branch)).append("\n");
sb.append(" buildId: ").append(toIndentedString(buildId)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" updated: ").append(toIndentedString(updated)).append("\n");
sb.append(" jobs: ").append(toIndentedString(jobs)).append("\n");
sb.append(" buildNumber: ").append(toIndentedString(buildNumber)).append("\n");
sb.append(" messageExtended: ").append(toIndentedString(messageExtended)).append("\n");
sb.append(" isTag: ").append(toIndentedString(isTag)).append("\n");
sb.append(" commitId: ").append(toIndentedString(commitId)).append("\n");
sb.append(" authorName: ").append(toIndentedString(authorName)).append("\n");
sb.append(" authorUsername: ").append(toIndentedString(authorUsername)).append("\n");
sb.append(" committerName: ").append(toIndentedString(committerName)).append("\n");
sb.append(" committerUsername: ").append(toIndentedString(committerUsername)).append("\n");
sb.append(" committed: ").append(toIndentedString(committed)).append("\n");
sb.append(" pullRequestId: ").append(toIndentedString(pullRequestId)).append("\n");
sb.append(" pullRequestName: ").append(toIndentedString(pullRequestName)).append("\n");
sb.append(" messages: ").append(toIndentedString(messages)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" started: ").append(toIndentedString(started)).append("\n");
sb.append(" finished: ").append(toIndentedString(finished)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy