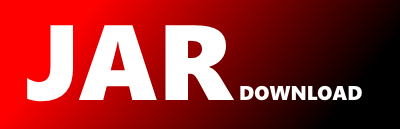
name.kevinlocke.appveyor.model.NotificationSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appveyor-swagger Show documentation
Show all versions of appveyor-swagger Show documentation
AppVeyor REST API Client generated from the unofficial Swagger API
definition in Java.
The newest version!
/*
* AppVeyor REST API
* AppVeyor is a hosted continuous integration service which runs on Microsoft Windows. The AppVeyor REST API provides a RESTful way to interact with the AppVeyor service. This includes managing projects, builds, deployments, and the teams that build them. Additional help and discussion of the AppVeyor REST API is available at http://help.appveyor.com/discussions This Swagger definition is an **unofficial** description of the AppVeyor REST API maintained at https://github.com/kevinoid/appveyor-swagger Please report any issues or suggestions for this Swagger definition at https://github.com/kevinoid/appveyor-swagger/issues/new #### API Conventions Fields which are missing from update operations (`PUT` requests) are typically reset to their default values. So although most fields are not technically required, they should usually be specified in practice.
*
* OpenAPI spec version: 0.20170106.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package name.kevinlocke.appveyor.model;
import java.util.Objects;
import com.google.gson.annotations.SerializedName;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import name.kevinlocke.appveyor.model.HttpMethodRestricted;
import name.kevinlocke.appveyor.model.NotificationSettingsType;
import name.kevinlocke.appveyor.model.StoredNameValue;
import name.kevinlocke.appveyor.model.StoredValue;
import name.kevinlocke.appveyor.model.StringValueObject;
import javax.validation.constraints.*;
/**
* This type is the union of the settings types for each of the various notification types supported by the API. The properties correspond to the following notification types: #### All Types - onBuildSuccess - onBuildFailure - onBuildStatusChanged #### Campfire - account - authToken - room - template #### Email - subjectTemplate - bodyTemplate - recipients - recipientsValue #### GitHubPullRequest - authToken - template #### HipChat - authToken - from - room - template - serverUrl #### Slack - incomingWebhookUrl - authToken - channel - template #### Webhook - method - url - headers - headersValue - addCustomRequestBody - customRequestBodyContentType - customRequestBody #### VSOTeamRoom - vsoAccount - username - password - room - template
*/
@ApiModel(description = "This type is the union of the settings types for each of the various notification types supported by the API. The properties correspond to the following notification types: #### All Types - onBuildSuccess - onBuildFailure - onBuildStatusChanged #### Campfire - account - authToken - room - template #### Email - subjectTemplate - bodyTemplate - recipients - recipientsValue #### GitHubPullRequest - authToken - template #### HipChat - authToken - from - room - template - serverUrl #### Slack - incomingWebhookUrl - authToken - channel - template #### Webhook - method - url - headers - headersValue - addCustomRequestBody - customRequestBodyContentType - customRequestBody #### VSOTeamRoom - vsoAccount - username - password - room - template ")
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2017-11-23T14:03:51.855-07:00")
public class NotificationSettings {
@SerializedName("$type")
private NotificationSettingsType type = null;
@SerializedName("onBuildSuccess")
private Boolean onBuildSuccess = null;
@SerializedName("onBuildFailure")
private Boolean onBuildFailure = null;
@SerializedName("onBuildStatusChanged")
private Boolean onBuildStatusChanged = null;
@SerializedName("account")
private String account = null;
@SerializedName("authToken")
private StoredValue authToken = null;
@SerializedName("room")
private String room = null;
@SerializedName("template")
private String template = null;
@SerializedName("subjectTemplate")
private String subjectTemplate = null;
@SerializedName("bodyTemplate")
private String bodyTemplate = null;
@SerializedName("recipients")
private List recipients = new ArrayList();
@SerializedName("recipientsValue")
private String recipientsValue = null;
@SerializedName("from")
private String from = null;
@SerializedName("serverUrl")
private String serverUrl = null;
@SerializedName("incomingWebhookUrl")
private String incomingWebhookUrl = null;
@SerializedName("channel")
private String channel = null;
@SerializedName("method")
private HttpMethodRestricted method = null;
@SerializedName("url")
private String url = null;
@SerializedName("headers")
private List headers = new ArrayList();
@SerializedName("headersValue")
private String headersValue = null;
@SerializedName("addCustomRequestBody")
private Boolean addCustomRequestBody = null;
@SerializedName("customRequestBodyContentType")
private String customRequestBodyContentType = null;
@SerializedName("customRequestBody")
private String customRequestBody = null;
@SerializedName("vsoAccount")
private String vsoAccount = null;
@SerializedName("username")
private String username = null;
@SerializedName("password")
private StoredValue password = null;
public NotificationSettings type(NotificationSettingsType type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@ApiModelProperty(example = "null", value = "")
public NotificationSettingsType getType() {
return type;
}
public void setType(NotificationSettingsType type) {
this.type = type;
}
public NotificationSettings onBuildSuccess(Boolean onBuildSuccess) {
this.onBuildSuccess = onBuildSuccess;
return this;
}
/**
* Get onBuildSuccess
* @return onBuildSuccess
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getOnBuildSuccess() {
return onBuildSuccess;
}
public void setOnBuildSuccess(Boolean onBuildSuccess) {
this.onBuildSuccess = onBuildSuccess;
}
public NotificationSettings onBuildFailure(Boolean onBuildFailure) {
this.onBuildFailure = onBuildFailure;
return this;
}
/**
* Get onBuildFailure
* @return onBuildFailure
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getOnBuildFailure() {
return onBuildFailure;
}
public void setOnBuildFailure(Boolean onBuildFailure) {
this.onBuildFailure = onBuildFailure;
}
public NotificationSettings onBuildStatusChanged(Boolean onBuildStatusChanged) {
this.onBuildStatusChanged = onBuildStatusChanged;
return this;
}
/**
* Get onBuildStatusChanged
* @return onBuildStatusChanged
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getOnBuildStatusChanged() {
return onBuildStatusChanged;
}
public void setOnBuildStatusChanged(Boolean onBuildStatusChanged) {
this.onBuildStatusChanged = onBuildStatusChanged;
}
public NotificationSettings account(String account) {
this.account = account;
return this;
}
/**
* Get account
* @return account
**/
@ApiModelProperty(example = "null", value = "")
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public NotificationSettings authToken(StoredValue authToken) {
this.authToken = authToken;
return this;
}
/**
* Get authToken
* @return authToken
**/
@ApiModelProperty(example = "null", value = "")
public StoredValue getAuthToken() {
return authToken;
}
public void setAuthToken(StoredValue authToken) {
this.authToken = authToken;
}
public NotificationSettings room(String room) {
this.room = room;
return this;
}
/**
* Get room
* @return room
**/
@ApiModelProperty(example = "null", value = "")
public String getRoom() {
return room;
}
public void setRoom(String room) {
this.room = room;
}
public NotificationSettings template(String template) {
this.template = template;
return this;
}
/**
* Get template
* @return template
**/
@ApiModelProperty(example = "null", value = "")
public String getTemplate() {
return template;
}
public void setTemplate(String template) {
this.template = template;
}
public NotificationSettings subjectTemplate(String subjectTemplate) {
this.subjectTemplate = subjectTemplate;
return this;
}
/**
* Get subjectTemplate
* @return subjectTemplate
**/
@ApiModelProperty(example = "null", value = "")
public String getSubjectTemplate() {
return subjectTemplate;
}
public void setSubjectTemplate(String subjectTemplate) {
this.subjectTemplate = subjectTemplate;
}
public NotificationSettings bodyTemplate(String bodyTemplate) {
this.bodyTemplate = bodyTemplate;
return this;
}
/**
* Get bodyTemplate
* @return bodyTemplate
**/
@ApiModelProperty(example = "null", value = "")
public String getBodyTemplate() {
return bodyTemplate;
}
public void setBodyTemplate(String bodyTemplate) {
this.bodyTemplate = bodyTemplate;
}
public NotificationSettings recipients(List recipients) {
this.recipients = recipients;
return this;
}
public NotificationSettings addRecipientsItem(StringValueObject recipientsItem) {
this.recipients.add(recipientsItem);
return this;
}
/**
* Get recipients
* @return recipients
**/
@ApiModelProperty(example = "null", value = "")
public List getRecipients() {
return recipients;
}
public void setRecipients(List recipients) {
this.recipients = recipients;
}
public NotificationSettings recipientsValue(String recipientsValue) {
this.recipientsValue = recipientsValue;
return this;
}
/**
* Get recipientsValue
* @return recipientsValue
**/
@ApiModelProperty(example = "null", value = "")
public String getRecipientsValue() {
return recipientsValue;
}
public void setRecipientsValue(String recipientsValue) {
this.recipientsValue = recipientsValue;
}
public NotificationSettings from(String from) {
this.from = from;
return this;
}
/**
* Get from
* @return from
**/
@ApiModelProperty(example = "null", value = "")
public String getFrom() {
return from;
}
public void setFrom(String from) {
this.from = from;
}
public NotificationSettings serverUrl(String serverUrl) {
this.serverUrl = serverUrl;
return this;
}
/**
* Get serverUrl
* @return serverUrl
**/
@ApiModelProperty(example = "null", value = "")
public String getServerUrl() {
return serverUrl;
}
public void setServerUrl(String serverUrl) {
this.serverUrl = serverUrl;
}
public NotificationSettings incomingWebhookUrl(String incomingWebhookUrl) {
this.incomingWebhookUrl = incomingWebhookUrl;
return this;
}
/**
* Get incomingWebhookUrl
* @return incomingWebhookUrl
**/
@ApiModelProperty(example = "null", value = "")
public String getIncomingWebhookUrl() {
return incomingWebhookUrl;
}
public void setIncomingWebhookUrl(String incomingWebhookUrl) {
this.incomingWebhookUrl = incomingWebhookUrl;
}
public NotificationSettings channel(String channel) {
this.channel = channel;
return this;
}
/**
* Get channel
* @return channel
**/
@ApiModelProperty(example = "null", value = "")
public String getChannel() {
return channel;
}
public void setChannel(String channel) {
this.channel = channel;
}
public NotificationSettings method(HttpMethodRestricted method) {
this.method = method;
return this;
}
/**
* Get method
* @return method
**/
@ApiModelProperty(example = "null", value = "")
public HttpMethodRestricted getMethod() {
return method;
}
public void setMethod(HttpMethodRestricted method) {
this.method = method;
}
public NotificationSettings url(String url) {
this.url = url;
return this;
}
/**
* Get url
* @return url
**/
@ApiModelProperty(example = "null", value = "")
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public NotificationSettings headers(List headers) {
this.headers = headers;
return this;
}
public NotificationSettings addHeadersItem(StoredNameValue headersItem) {
this.headers.add(headersItem);
return this;
}
/**
* Get headers
* @return headers
**/
@ApiModelProperty(example = "null", value = "")
public List getHeaders() {
return headers;
}
public void setHeaders(List headers) {
this.headers = headers;
}
public NotificationSettings headersValue(String headersValue) {
this.headersValue = headersValue;
return this;
}
/**
* Get headersValue
* @return headersValue
**/
@ApiModelProperty(example = "null", value = "")
public String getHeadersValue() {
return headersValue;
}
public void setHeadersValue(String headersValue) {
this.headersValue = headersValue;
}
public NotificationSettings addCustomRequestBody(Boolean addCustomRequestBody) {
this.addCustomRequestBody = addCustomRequestBody;
return this;
}
/**
* Get addCustomRequestBody
* @return addCustomRequestBody
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getAddCustomRequestBody() {
return addCustomRequestBody;
}
public void setAddCustomRequestBody(Boolean addCustomRequestBody) {
this.addCustomRequestBody = addCustomRequestBody;
}
public NotificationSettings customRequestBodyContentType(String customRequestBodyContentType) {
this.customRequestBodyContentType = customRequestBodyContentType;
return this;
}
/**
* Get customRequestBodyContentType
* @return customRequestBodyContentType
**/
@ApiModelProperty(example = "null", value = "")
public String getCustomRequestBodyContentType() {
return customRequestBodyContentType;
}
public void setCustomRequestBodyContentType(String customRequestBodyContentType) {
this.customRequestBodyContentType = customRequestBodyContentType;
}
public NotificationSettings customRequestBody(String customRequestBody) {
this.customRequestBody = customRequestBody;
return this;
}
/**
* Get customRequestBody
* @return customRequestBody
**/
@ApiModelProperty(example = "null", value = "")
public String getCustomRequestBody() {
return customRequestBody;
}
public void setCustomRequestBody(String customRequestBody) {
this.customRequestBody = customRequestBody;
}
public NotificationSettings vsoAccount(String vsoAccount) {
this.vsoAccount = vsoAccount;
return this;
}
/**
* Get vsoAccount
* @return vsoAccount
**/
@ApiModelProperty(example = "null", value = "")
public String getVsoAccount() {
return vsoAccount;
}
public void setVsoAccount(String vsoAccount) {
this.vsoAccount = vsoAccount;
}
public NotificationSettings username(String username) {
this.username = username;
return this;
}
/**
* Get username
* @return username
**/
@ApiModelProperty(example = "null", value = "")
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public NotificationSettings password(StoredValue password) {
this.password = password;
return this;
}
/**
* Get password
* @return password
**/
@ApiModelProperty(example = "null", value = "")
public StoredValue getPassword() {
return password;
}
public void setPassword(StoredValue password) {
this.password = password;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
NotificationSettings notificationSettings = (NotificationSettings) o;
return Objects.equals(this.type, notificationSettings.type) &&
Objects.equals(this.onBuildSuccess, notificationSettings.onBuildSuccess) &&
Objects.equals(this.onBuildFailure, notificationSettings.onBuildFailure) &&
Objects.equals(this.onBuildStatusChanged, notificationSettings.onBuildStatusChanged) &&
Objects.equals(this.account, notificationSettings.account) &&
Objects.equals(this.authToken, notificationSettings.authToken) &&
Objects.equals(this.room, notificationSettings.room) &&
Objects.equals(this.template, notificationSettings.template) &&
Objects.equals(this.subjectTemplate, notificationSettings.subjectTemplate) &&
Objects.equals(this.bodyTemplate, notificationSettings.bodyTemplate) &&
Objects.equals(this.recipients, notificationSettings.recipients) &&
Objects.equals(this.recipientsValue, notificationSettings.recipientsValue) &&
Objects.equals(this.from, notificationSettings.from) &&
Objects.equals(this.serverUrl, notificationSettings.serverUrl) &&
Objects.equals(this.incomingWebhookUrl, notificationSettings.incomingWebhookUrl) &&
Objects.equals(this.channel, notificationSettings.channel) &&
Objects.equals(this.method, notificationSettings.method) &&
Objects.equals(this.url, notificationSettings.url) &&
Objects.equals(this.headers, notificationSettings.headers) &&
Objects.equals(this.headersValue, notificationSettings.headersValue) &&
Objects.equals(this.addCustomRequestBody, notificationSettings.addCustomRequestBody) &&
Objects.equals(this.customRequestBodyContentType, notificationSettings.customRequestBodyContentType) &&
Objects.equals(this.customRequestBody, notificationSettings.customRequestBody) &&
Objects.equals(this.vsoAccount, notificationSettings.vsoAccount) &&
Objects.equals(this.username, notificationSettings.username) &&
Objects.equals(this.password, notificationSettings.password);
}
@Override
public int hashCode() {
return Objects.hash(type, onBuildSuccess, onBuildFailure, onBuildStatusChanged, account, authToken, room, template, subjectTemplate, bodyTemplate, recipients, recipientsValue, from, serverUrl, incomingWebhookUrl, channel, method, url, headers, headersValue, addCustomRequestBody, customRequestBodyContentType, customRequestBody, vsoAccount, username, password);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class NotificationSettings {\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" onBuildSuccess: ").append(toIndentedString(onBuildSuccess)).append("\n");
sb.append(" onBuildFailure: ").append(toIndentedString(onBuildFailure)).append("\n");
sb.append(" onBuildStatusChanged: ").append(toIndentedString(onBuildStatusChanged)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" authToken: ").append(toIndentedString(authToken)).append("\n");
sb.append(" room: ").append(toIndentedString(room)).append("\n");
sb.append(" template: ").append(toIndentedString(template)).append("\n");
sb.append(" subjectTemplate: ").append(toIndentedString(subjectTemplate)).append("\n");
sb.append(" bodyTemplate: ").append(toIndentedString(bodyTemplate)).append("\n");
sb.append(" recipients: ").append(toIndentedString(recipients)).append("\n");
sb.append(" recipientsValue: ").append(toIndentedString(recipientsValue)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" serverUrl: ").append(toIndentedString(serverUrl)).append("\n");
sb.append(" incomingWebhookUrl: ").append(toIndentedString(incomingWebhookUrl)).append("\n");
sb.append(" channel: ").append(toIndentedString(channel)).append("\n");
sb.append(" method: ").append(toIndentedString(method)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" headers: ").append(toIndentedString(headers)).append("\n");
sb.append(" headersValue: ").append(toIndentedString(headersValue)).append("\n");
sb.append(" addCustomRequestBody: ").append(toIndentedString(addCustomRequestBody)).append("\n");
sb.append(" customRequestBodyContentType: ").append(toIndentedString(customRequestBodyContentType)).append("\n");
sb.append(" customRequestBody: ").append(toIndentedString(customRequestBody)).append("\n");
sb.append(" vsoAccount: ").append(toIndentedString(vsoAccount)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append(" password: ").append(toIndentedString(password)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy