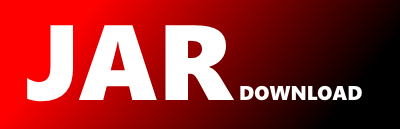
name.kevinlocke.appveyor.model.UserAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appveyor-swagger Show documentation
Show all versions of appveyor-swagger Show documentation
AppVeyor REST API Client generated from the unofficial Swagger API
definition in Java.
The newest version!
/*
* AppVeyor REST API
* AppVeyor is a hosted continuous integration service which runs on Microsoft Windows. The AppVeyor REST API provides a RESTful way to interact with the AppVeyor service. This includes managing projects, builds, deployments, and the teams that build them. Additional help and discussion of the AppVeyor REST API is available at http://help.appveyor.com/discussions This Swagger definition is an **unofficial** description of the AppVeyor REST API maintained at https://github.com/kevinoid/appveyor-swagger Please report any issues or suggestions for this Swagger definition at https://github.com/kevinoid/appveyor-swagger/issues/new #### API Conventions Fields which are missing from update operations (`PUT` requests) are typically reset to their default values. So although most fields are not technically required, they should usually be specified in practice.
*
* OpenAPI spec version: 0.20170106.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package name.kevinlocke.appveyor.model;
import java.util.Objects;
import com.google.gson.annotations.SerializedName;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import name.kevinlocke.appveyor.model.BuildNotificationFrequency;
import name.kevinlocke.appveyor.model.DeploymentNotificationFrequency;
import name.kevinlocke.appveyor.model.Timestamped;
import name.kevinlocke.appveyor.model.UserAccountSettings;
import org.joda.time.DateTime;
import javax.validation.constraints.*;
/**
* UserAccount
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2017-11-23T14:03:51.855-07:00")
public class UserAccount {
@SerializedName("created")
private DateTime created = null;
@SerializedName("updated")
private DateTime updated = null;
@SerializedName("successfulBuildNotification")
private BuildNotificationFrequency successfulBuildNotification = null;
@SerializedName("failedBuildNotification")
private BuildNotificationFrequency failedBuildNotification = null;
@SerializedName("notifyWhenBuildStatusChangedOnly")
private Boolean notifyWhenBuildStatusChangedOnly = false;
@SerializedName("successfulDeploymentNotification")
private DeploymentNotificationFrequency successfulDeploymentNotification = null;
@SerializedName("failedDeploymentNotification")
private DeploymentNotificationFrequency failedDeploymentNotification = null;
@SerializedName("notifyWhenDeploymentStatusChangedOnly")
private Boolean notifyWhenDeploymentStatusChangedOnly = false;
@SerializedName("accountId")
private Integer accountId = null;
@SerializedName("accountName")
private String accountName = null;
@SerializedName("isOwner")
private Boolean isOwner = null;
@SerializedName("isCollaborator")
private Boolean isCollaborator = null;
@SerializedName("userId")
private Integer userId = null;
@SerializedName("fullName")
private String fullName = null;
@SerializedName("email")
private String email = null;
@SerializedName("password")
private String password = null;
@SerializedName("roleId")
private Integer roleId = null;
@SerializedName("roleName")
private String roleName = null;
@SerializedName("pageSize")
private Integer pageSize = null;
/**
* Get created
* @return created
**/
@ApiModelProperty(example = "null", value = "")
public DateTime getCreated() {
return created;
}
/**
* Get updated
* @return updated
**/
@ApiModelProperty(example = "null", value = "")
public DateTime getUpdated() {
return updated;
}
public UserAccount successfulBuildNotification(BuildNotificationFrequency successfulBuildNotification) {
this.successfulBuildNotification = successfulBuildNotification;
return this;
}
/**
* Get successfulBuildNotification
* @return successfulBuildNotification
**/
@NotNull
@ApiModelProperty(example = "null", required = true, value = "")
public BuildNotificationFrequency getSuccessfulBuildNotification() {
return successfulBuildNotification;
}
public void setSuccessfulBuildNotification(BuildNotificationFrequency successfulBuildNotification) {
this.successfulBuildNotification = successfulBuildNotification;
}
public UserAccount failedBuildNotification(BuildNotificationFrequency failedBuildNotification) {
this.failedBuildNotification = failedBuildNotification;
return this;
}
/**
* Get failedBuildNotification
* @return failedBuildNotification
**/
@NotNull
@ApiModelProperty(example = "null", required = true, value = "")
public BuildNotificationFrequency getFailedBuildNotification() {
return failedBuildNotification;
}
public void setFailedBuildNotification(BuildNotificationFrequency failedBuildNotification) {
this.failedBuildNotification = failedBuildNotification;
}
public UserAccount notifyWhenBuildStatusChangedOnly(Boolean notifyWhenBuildStatusChangedOnly) {
this.notifyWhenBuildStatusChangedOnly = notifyWhenBuildStatusChangedOnly;
return this;
}
/**
* Note that this value is `true` on user creation, but behaves as `false` when not specified on update.
* @return notifyWhenBuildStatusChangedOnly
**/
@ApiModelProperty(example = "null", value = "Note that this value is `true` on user creation, but behaves as `false` when not specified on update. ")
public Boolean getNotifyWhenBuildStatusChangedOnly() {
return notifyWhenBuildStatusChangedOnly;
}
public void setNotifyWhenBuildStatusChangedOnly(Boolean notifyWhenBuildStatusChangedOnly) {
this.notifyWhenBuildStatusChangedOnly = notifyWhenBuildStatusChangedOnly;
}
public UserAccount successfulDeploymentNotification(DeploymentNotificationFrequency successfulDeploymentNotification) {
this.successfulDeploymentNotification = successfulDeploymentNotification;
return this;
}
/**
* Get successfulDeploymentNotification
* @return successfulDeploymentNotification
**/
@NotNull
@ApiModelProperty(example = "null", required = true, value = "")
public DeploymentNotificationFrequency getSuccessfulDeploymentNotification() {
return successfulDeploymentNotification;
}
public void setSuccessfulDeploymentNotification(DeploymentNotificationFrequency successfulDeploymentNotification) {
this.successfulDeploymentNotification = successfulDeploymentNotification;
}
public UserAccount failedDeploymentNotification(DeploymentNotificationFrequency failedDeploymentNotification) {
this.failedDeploymentNotification = failedDeploymentNotification;
return this;
}
/**
* Get failedDeploymentNotification
* @return failedDeploymentNotification
**/
@NotNull
@ApiModelProperty(example = "null", required = true, value = "")
public DeploymentNotificationFrequency getFailedDeploymentNotification() {
return failedDeploymentNotification;
}
public void setFailedDeploymentNotification(DeploymentNotificationFrequency failedDeploymentNotification) {
this.failedDeploymentNotification = failedDeploymentNotification;
}
public UserAccount notifyWhenDeploymentStatusChangedOnly(Boolean notifyWhenDeploymentStatusChangedOnly) {
this.notifyWhenDeploymentStatusChangedOnly = notifyWhenDeploymentStatusChangedOnly;
return this;
}
/**
* Note that this value is `true` on user creation, but behaves as `false` when not specified on update.
* @return notifyWhenDeploymentStatusChangedOnly
**/
@ApiModelProperty(example = "null", value = "Note that this value is `true` on user creation, but behaves as `false` when not specified on update. ")
public Boolean getNotifyWhenDeploymentStatusChangedOnly() {
return notifyWhenDeploymentStatusChangedOnly;
}
public void setNotifyWhenDeploymentStatusChangedOnly(Boolean notifyWhenDeploymentStatusChangedOnly) {
this.notifyWhenDeploymentStatusChangedOnly = notifyWhenDeploymentStatusChangedOnly;
}
/**
* Get accountId
* minimum: 0
* @return accountId
**/
@Min(0)
@ApiModelProperty(example = "null", value = "")
public Integer getAccountId() {
return accountId;
}
/**
* Get accountName
* @return accountName
**/
@ApiModelProperty(example = "null", value = "")
public String getAccountName() {
return accountName;
}
/**
* Get isOwner
* @return isOwner
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getIsOwner() {
return isOwner;
}
/**
* Get isCollaborator
* @return isCollaborator
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getIsCollaborator() {
return isCollaborator;
}
public UserAccount userId(Integer userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* minimum: 0
* @return userId
**/
@Min(0)
@ApiModelProperty(example = "null", value = "")
public Integer getUserId() {
return userId;
}
public void setUserId(Integer userId) {
this.userId = userId;
}
public UserAccount fullName(String fullName) {
this.fullName = fullName;
return this;
}
/**
* Get fullName
* @return fullName
**/
@NotNull
@ApiModelProperty(example = "null", required = true, value = "")
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
public UserAccount email(String email) {
this.email = email;
return this;
}
/**
* Get email
* @return email
**/
@NotNull
@ApiModelProperty(example = "null", required = true, value = "")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public UserAccount password(String password) {
this.password = password;
return this;
}
/**
* Get password
* @return password
**/
@Size(min=1)
@ApiModelProperty(example = "null", value = "")
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public UserAccount roleId(Integer roleId) {
this.roleId = roleId;
return this;
}
/**
* Get roleId
* minimum: 0
* @return roleId
**/
@Min(0)
@ApiModelProperty(example = "null", value = "")
public Integer getRoleId() {
return roleId;
}
public void setRoleId(Integer roleId) {
this.roleId = roleId;
}
/**
* Get roleName
* @return roleName
**/
@ApiModelProperty(example = "null", value = "")
public String getRoleName() {
return roleName;
}
/**
* Get pageSize
* minimum: 0
* @return pageSize
**/
@Min(0)
@ApiModelProperty(example = "null", value = "")
public Integer getPageSize() {
return pageSize;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserAccount userAccount = (UserAccount) o;
return Objects.equals(this.created, userAccount.created) &&
Objects.equals(this.updated, userAccount.updated) &&
Objects.equals(this.successfulBuildNotification, userAccount.successfulBuildNotification) &&
Objects.equals(this.failedBuildNotification, userAccount.failedBuildNotification) &&
Objects.equals(this.notifyWhenBuildStatusChangedOnly, userAccount.notifyWhenBuildStatusChangedOnly) &&
Objects.equals(this.successfulDeploymentNotification, userAccount.successfulDeploymentNotification) &&
Objects.equals(this.failedDeploymentNotification, userAccount.failedDeploymentNotification) &&
Objects.equals(this.notifyWhenDeploymentStatusChangedOnly, userAccount.notifyWhenDeploymentStatusChangedOnly) &&
Objects.equals(this.accountId, userAccount.accountId) &&
Objects.equals(this.accountName, userAccount.accountName) &&
Objects.equals(this.isOwner, userAccount.isOwner) &&
Objects.equals(this.isCollaborator, userAccount.isCollaborator) &&
Objects.equals(this.userId, userAccount.userId) &&
Objects.equals(this.fullName, userAccount.fullName) &&
Objects.equals(this.email, userAccount.email) &&
Objects.equals(this.password, userAccount.password) &&
Objects.equals(this.roleId, userAccount.roleId) &&
Objects.equals(this.roleName, userAccount.roleName) &&
Objects.equals(this.pageSize, userAccount.pageSize);
}
@Override
public int hashCode() {
return Objects.hash(created, updated, successfulBuildNotification, failedBuildNotification, notifyWhenBuildStatusChangedOnly, successfulDeploymentNotification, failedDeploymentNotification, notifyWhenDeploymentStatusChangedOnly, accountId, accountName, isOwner, isCollaborator, userId, fullName, email, password, roleId, roleName, pageSize);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserAccount {\n");
sb.append(" created: ").append(toIndentedString(created)).append("\n");
sb.append(" updated: ").append(toIndentedString(updated)).append("\n");
sb.append(" successfulBuildNotification: ").append(toIndentedString(successfulBuildNotification)).append("\n");
sb.append(" failedBuildNotification: ").append(toIndentedString(failedBuildNotification)).append("\n");
sb.append(" notifyWhenBuildStatusChangedOnly: ").append(toIndentedString(notifyWhenBuildStatusChangedOnly)).append("\n");
sb.append(" successfulDeploymentNotification: ").append(toIndentedString(successfulDeploymentNotification)).append("\n");
sb.append(" failedDeploymentNotification: ").append(toIndentedString(failedDeploymentNotification)).append("\n");
sb.append(" notifyWhenDeploymentStatusChangedOnly: ").append(toIndentedString(notifyWhenDeploymentStatusChangedOnly)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountName: ").append(toIndentedString(accountName)).append("\n");
sb.append(" isOwner: ").append(toIndentedString(isOwner)).append("\n");
sb.append(" isCollaborator: ").append(toIndentedString(isCollaborator)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" fullName: ").append(toIndentedString(fullName)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" password: ").append(toIndentedString(password)).append("\n");
sb.append(" roleId: ").append(toIndentedString(roleId)).append("\n");
sb.append(" roleName: ").append(toIndentedString(roleName)).append("\n");
sb.append(" pageSize: ").append(toIndentedString(pageSize)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy