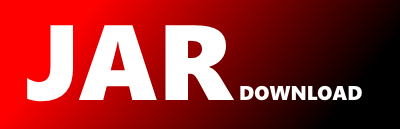
name.neuhalfen.projects.crypto.bouncycastle.openpgp.keys.generation.KeySpecBuilderInterface Maven / Gradle / Ivy
Show all versions of bouncy-gpg Show documentation
/*
* Copyright 2018 Paul Schaub.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package name.neuhalfen.projects.crypto.bouncycastle.openpgp.keys.generation;
import java.util.Collection;
import name.neuhalfen.projects.crypto.bouncycastle.openpgp.algorithms.Feature;
import name.neuhalfen.projects.crypto.bouncycastle.openpgp.algorithms.PGPCompressionAlgorithms;
import name.neuhalfen.projects.crypto.bouncycastle.openpgp.algorithms.PGPHashAlgorithms;
import name.neuhalfen.projects.crypto.bouncycastle.openpgp.algorithms.PGPSymmetricEncryptionAlgorithms;
import org.bouncycastle.bcpg.sig.Features;
public interface KeySpecBuilderInterface {
/**
* Configure the GPG keyflags for a key to allow all key usages. This is not bad or
* dangerous, but often considered bad style because normally different signature and
* encryption keys are used and recommended.
*
* GPG keys and subkeys carry flags that describe their purpose. Most commonly the master key
* carries the SIGN_DATA and CERTIFY_OTHER flags and a dedicated encryption key carries the
* ENCRYPT_STORAGE and ENCRYPT_COMMS flags.
*
*
* To quote from
* rfc4880 section 5.2.3.21:
*
* The flags in this packet may appear in self-signatures or in
* certification signatures. They mean different things depending on
* who is making the statement -- for example, a certification signature
* that has the "sign data" flag is stating that the certification is
* for that use. On the other hand, the "communications encryption"
* flag in a self-signature is stating a preference that a given key be
* used for communications. Note however, that it is a thorny issue to
* determine what is "communications" and what is "storage". This
* decision is left wholly up to the implementation; the authors of this
* document do not claim any special wisdom on the issue and realize
* that accepted opinion may change.
*
* The "split key" (0x10) and "group key" (0x80) flags are placed on a
* self-signature only; they are meaningless on a certification
* signature. They SHOULD be placed only on a direct-key signature
* (type 0x1F) or a subkey signature (type 0x18), one that refers to the
* key the flag applies to.
*
*
* @param flags Flags to be enabled for this sub- or master-key
*
* @return next step
*/
WithDetailedConfiguration allowKeyToBeUsedTo(KeyFlag... flags);
/**
* Configure the GPG keyflags for a key.
*
* GPG keys and subkeys carry flags that describe their purpose. Most commonly the master key
* carries the SIGN_DATA and CERTIFY_OTHER flags and a dedicated encryption key carries the
* ENCRYPT_STORAGE and ENCRYPT_COMMS flags.
*
*
* To quote from
* rfc4880 section 5.2.3.21:
*
* The flags in this packet may appear in self-signatures or in
* certification signatures. They mean different things depending on
* who is making the statement -- for example, a certification signature
* that has the "sign data" flag is stating that the certification is
* for that use. On the other hand, the "communications encryption"
* flag in a self-signature is stating a preference that a given key be
* used for communications. Note however, that it is a thorny issue to
* determine what is "communications" and what is "storage". This
* decision is left wholly up to the implementation; the authors of this
* document do not claim any special wisdom on the issue and realize
* that accepted opinion may change.
*
* The "split key" (0x10) and "group key" (0x80) flags are placed on a
* self-signature only; they are meaningless on a certification
* signature. They SHOULD be placed only on a direct-key signature
* (type 0x1F) or a subkey signature (type 0x18), one that refers to the
* key the flag applies to.
*
*
* @return next step
*/
WithDetailedConfiguration allowKeyToBeUsedForEverything();
/**
* Copy all key flags, algorithms, ... from the parent key.
* @return key spec.
*/
KeySpec withInheritedSubPackets();
interface WithDetailedConfiguration {
WithPreferredSymmetricAlgorithms withDetailedConfiguration();
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
*
* - preferred algorithms via recommendedAlgorithmIds()
* - enables the FEATURE_MODIFICATION_DETECTION feature
*
*
* @return keyspec
*
* @see PGPCompressionAlgorithms#recommendedAlgorithmIds()
* @see PGPSymmetricEncryptionAlgorithms#recommendedAlgorithmIds()
* @see PGPHashAlgorithms#recommendedAlgorithmIds()
* @see Features#FEATURE_MODIFICATION_DETECTION
*/
KeySpec withDefaultAlgorithms();
}
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
*
*
* This is documented in RFC4880 5.2.3.7.
* Preferred Symmetric Algorithms:
*
*
* Symmetric algorithm numbers that indicate which algorithms the key
* holder prefers to use. The subpacket body is an ordered list of
* octets with the most preferred listed first. It is assumed that only
* algorithms listed are supported by the recipient's software.
* Algorithm numbers are in Section 9. This is only found on a self-
* signature.
*
*/
interface WithPreferredSymmetricAlgorithms {
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
*
* This is documented in RFC4880 5.2.3.7.
* Preferred Symmetric Algorithms:
*
*
* Symmetric algorithm numbers that indicate which algorithms the key
* holder prefers to use. The subpacket body is an ordered list of
* octets with the most preferred listed first. It is assumed that only
* algorithms listed are supported by the recipient's software.
* Algorithm numbers are in Section 9. This is only found on a self-
* signature.
*
*
* @param algorithms set of preferred algorithms
*
* @return next builder step
*/
WithPreferredHashAlgorithms withPreferredSymmetricAlgorithms(
PGPSymmetricEncryptionAlgorithms... algorithms);
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
*
* This is documented in RFC4880 5.2.3.7.
* Preferred Symmetric Algorithms:
*
*
* Symmetric algorithm numbers that indicate which algorithms the key
* holder prefers to use. The subpacket body is an ordered list of
* octets with the most preferred listed first. It is assumed that only
* algorithms listed are supported by the recipient's software.
* Algorithm numbers are in Section 9. This is only found on a self-
* signature.
*
*
* @param algorithms set of preferred algorithms
*
* @return next builder step
*/
WithPreferredHashAlgorithms withPreferredSymmetricAlgorithms(
Collection algorithms);
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
* The default is to select algorithms that are deemed safe. {@link
* PGPSymmetricEncryptionAlgorithms#recommendedAlgorithms()}.
*
* This is documented in RFC4880 5.2.3.7.
* Preferred Symmetric Algorithms:
*
*
* Symmetric algorithm numbers that indicate which algorithms the key
* holder prefers to use. The subpacket body is an ordered list of
* octets with the most preferred listed first. It is assumed that only
* algorithms listed are supported by the recipient's software.
* Algorithm numbers are in Section 9. This is only found on a self-
* signature.
*
*
* @return next builder step
*
* @see PGPSymmetricEncryptionAlgorithms#recommendedAlgorithms()
*/
WithPreferredHashAlgorithms withDefaultSymmetricAlgorithms();
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
*
* Sets values for the following algorithms:
*
* - Hash
* - Symmetric encryption
* - Compression
*
* The default is to select algorithms that are deemed safe/sensible.
*
* This is documented in RFC4880 5.2.3.7.
* Preferred Symmetric Algorithms:
*
*
* Symmetric algorithm numbers that indicate which algorithms the key
* holder prefers to use. The subpacket body is an ordered list of
* octets with the most preferred listed first. It is assumed that only
* algorithms listed are supported by the recipient's software.
* Algorithm numbers are in Section 9. This is only found on a self-
* signature.
*
*
* @return next builder step
*
* @see PGPSymmetricEncryptionAlgorithms#recommendedAlgorithms()
* @see PGPCompressionAlgorithms#recommendedAlgorithms()
* @see PGPHashAlgorithms#recommendedAlgorithms()
*/
WithFeatures withDefaultAlgorithms();
}
interface WithPreferredHashAlgorithms {
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
* {@link PGPHashAlgorithms#recommendedAlgorithms()}.
*
* This is documented in RFC4880 5.2.3.8.
* Preferred Hash Algorithms:
*
*
* Message digest algorithm numbers that indicate which algorithms the
* key holder prefers to receive. Like the preferred symmetric
* algorithms, the list is ordered. Algorithm numbers are in Section 9.
* This is only found on a self-signature.
*
*
* @param algorithms the algorithms to set
*
* @return next builder step
*
* @see PGPHashAlgorithms#recommendedAlgorithms()
*/
WithPreferredCompressionAlgorithms withPreferredHashAlgorithms(
PGPHashAlgorithms... algorithms);
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
* {@link PGPHashAlgorithms#recommendedAlgorithms()}.
*
* This is documented in RFC4880 5.2.3.8.
* Preferred Hash Algorithms:
*
*
* Message digest algorithm numbers that indicate which algorithms the
* key holder prefers to receive. Like the preferred symmetric
* algorithms, the list is ordered. Algorithm numbers are in Section 9.
* This is only found on a self-signature.
*
*
* @param algorithms the algorithms to set
*
* @return next builder step
*
* @see PGPHashAlgorithms#recommendedAlgorithms()
*/
WithPreferredCompressionAlgorithms withPreferredHashAlgorithms(
Collection algorithms);
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
* The default is to select algorithms that are deemed safe. {@link
* PGPHashAlgorithms#recommendedAlgorithms()}.
*
* This is documented in RFC4880 5.2.3.8.
* Preferred Hash Algorithms:
*
*
* Message digest algorithm numbers that indicate which algorithms the
* key holder prefers to receive. Like the preferred symmetric
* algorithms, the list is ordered. Algorithm numbers are in Section 9.
* This is only found on a self-signature.
*
*
* @return next builder step
*
* @see PGPHashAlgorithms#recommendedAlgorithms()
*/
WithPreferredCompressionAlgorithms withDefaultHashAlgorithms();
}
interface WithPreferredCompressionAlgorithms {
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
* {@link PGPCompressionAlgorithms#recommendedAlgorithms()}.
*
* This is documented in RFC4880 5.2.3.9.
* Preferred Hash Algorithms:
*
*
* Compression algorithm numbers that indicate which algorithms the key
* holder prefers to use. Like the preferred symmetric algorithms, the
* list is ordered. Algorithm numbers are in Section 9. If this
* subpacket is not included, ZIP is preferred. A zero denotes that
* uncompressed data is preferred; the key holder's software might have
* no compression software in that implementation. This is only found
* on a self-signature.
*
*
* @param algorithms the algorithms to set
*
* @return next builder step
*
* @see PGPCompressionAlgorithms#recommendedAlgorithms()
*/
WithFeatures withPreferredCompressionAlgorithms(PGPCompressionAlgorithms... algorithms);
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
* {@link PGPCompressionAlgorithms#recommendedAlgorithms()}.
*
* This is documented in RFC4880 5.2.3.9.
* Preferred Hash Algorithms:
*
*
* Compression algorithm numbers that indicate which algorithms the key
* holder prefers to use. Like the preferred symmetric algorithms, the
* list is ordered. Algorithm numbers are in Section 9. If this
* subpacket is not included, ZIP is preferred. A zero denotes that
* uncompressed data is preferred; the key holder's software might have
* no compression software in that implementation. This is only found
* on a self-signature.
*
*
* @param algorithms the algorithms to set
*
* @return next builder step
*
* @see PGPCompressionAlgorithms#recommendedAlgorithms()
*/
WithFeatures withPreferredCompressionAlgorithms(
Collection algorithms);
/**
* Annotate the public key with the set of algorithms the key holder prefers to use. It is
* assumed that only algorithms listed are supported by the recipient's software.
* {@link PGPCompressionAlgorithms#recommendedAlgorithms()}.
*
* This is documented in RFC4880 5.2.3.9.
* Preferred Hash Algorithms:
*
*
* Compression algorithm numbers that indicate which algorithms the key
* holder prefers to use. Like the preferred symmetric algorithms, the
* list is ordered. Algorithm numbers are in Section 9. If this
* subpacket is not included, ZIP is preferred. A zero denotes that
* uncompressed data is preferred; the key holder's software might have
* no compression software in that implementation. This is only found
* on a self-signature.
*
*
* @return next builder step
*
* @see PGPCompressionAlgorithms#recommendedAlgorithms()
*/
WithFeatures withDefaultCompressionAlgorithms();
}
interface WithFeatures {
/**
* Add features to the key.
*
* @param feature the feature
*
* @return next step
*/
WithFeatures withFeature(Feature feature);
KeySpec done();
}
}