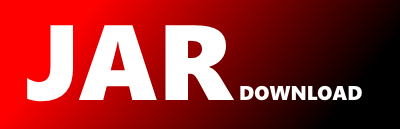
name.neuhalfen.projects.crypto.symmetric.keygeneration.impl.derivation.HKDFSHA256 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bouncy-gpg Show documentation
Show all versions of bouncy-gpg Show documentation
Make using Bouncy Castle with OpenPGP fun again!
The newest version!
package name.neuhalfen.projects.crypto.symmetric.keygeneration.impl.derivation;
import static java.util.Objects.requireNonNull;
import java.security.GeneralSecurityException;
import java.util.Arrays;
import javax.annotation.Nullable;
import name.neuhalfen.projects.crypto.internal.Preconditions;
import org.bouncycastle.crypto.DerivationParameters;
import org.bouncycastle.crypto.digests.SHA256Digest;
import org.bouncycastle.crypto.generators.HKDFBytesGenerator;
import org.bouncycastle.crypto.params.HKDFParameters;
/**
* Implements HKDF with SHA256 as hashing algorithm. The master key ("IKM - input key material") is
* stored as part of the instance.
* s
*
* - Key derivation
* function on Wikipedia.
* - RFC 5869 HMAC-based Extract-and-Expand Key
* Derivation Function (HKDF)
* - NIST
* Recommendation for Key Derivation through Extraction-then-Expansion, Special Publication
* SP800-56C
* - BSI
* TR-02102-1 Kryptographische Verfahren: Empfehlungen und Schlussellaengen
*
*/
public class HKDFSHA256 implements KeyDerivationFunction {
private final byte[] masterKey;
public HKDFSHA256(byte[] masterKey) {
requireNonNull(masterKey, "masterKey must not be null");
this.masterKey = Arrays.copyOf(masterKey, masterKey.length);
}
@Override
public byte[] deriveKey(@Nullable byte[] salt, byte[] info, int desiredKeyLengthInBits)
throws GeneralSecurityException {
requireNonNull(info, "info must not be null");
Preconditions.checkArgument(desiredKeyLengthInBits % 8 == 0,
"desiredKeyLengthInBits must be multiple of 8 but is " + desiredKeyLengthInBits);
final int desiredKeyLengthInBytes = desiredKeyLengthInBits / 8;
final DerivationParameters derivationParameters = new HKDFParameters(masterKey, salt, info);
final SHA256Digest digest = new SHA256Digest();
final HKDFBytesGenerator hkdfGenerator = new HKDFBytesGenerator(digest);
hkdfGenerator.init(derivationParameters);
final byte[] hkdf = new byte[desiredKeyLengthInBytes];
final int generatedKeyLength = hkdfGenerator.generateBytes(hkdf, 0, hkdf.length);
if (generatedKeyLength != desiredKeyLengthInBytes) {
throw new GeneralSecurityException(String
.format("Failed to derive key. Expected %d bytes, generated %d ", desiredKeyLengthInBytes,
generatedKeyLength));
}
return hkdf;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy