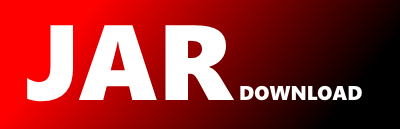
name.remal.org.w3c.dom.Node.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common-kotlin Show documentation
Show all versions of common-kotlin Show documentation
Java & Kotlin tools: common-kotlin
The newest version!
package name.remal
import org.w3c.dom.Document
import org.w3c.dom.Element
import org.w3c.dom.Node
import org.w3c.dom.Text
operator fun Node.plusAssign(node: Node) {
this.appendChild(node)
}
operator fun Node.plusAssign(text: String) {
this.appendTextNode(text)
}
fun T.remove(): T = this.apply {
this.parentNode.removeChild(this)
}
inline fun Node.getChildNodes(predicate: (node: Node) -> Boolean): List {
val result = mutableListOf()
this.childNodes.forEach {
if (predicate(it)) {
result.add(it)
}
}
return result
}
fun Node.getChildElements(tagName: String): List {
val result = mutableListOf()
this.childNodes.forEach {
if (it is Element) {
if (it.tagName == tagName || it.localName == tagName) {
result.add(it)
}
}
}
return result
}
fun Node.appendElement(tagName: String, attrs: Map = mapOf()): Element {
val ownerDocument: Document = this as? Document ?: this.ownerDocument
val element = ownerDocument.createElement(tagName)
attrs.forEach { name, value -> element.setAttribute(name, value.toString()) }
this.appendChild(element)
return element
}
fun Node.appendTextNode(data: String): Text {
val ownerDocument: Document = this as? Document ?: this.ownerDocument
val textNode = ownerDocument.createTextNode(data)
this.appendChild(textNode)
return textNode
}
fun Node.findElement(tagName: String, attrs: Map = mapOf()): Element? {
return this.getChildElements(tagName).firstOrNull { attrs.all { (name, value) -> it.getAttribute(name) == value.toString() } }
}
fun Node.findOrAppendElement(tagName: String, attrs: Map = mapOf(), onCreate: (element: Element) -> Unit = {}): Element {
return this.findElement(tagName, attrs) ?: this.appendElement(tagName, attrs).also(onCreate)
}
private fun stripSpacesImpl(node: Node) {
var child: Node? = node.firstChild
while (null != child) {
val nextChild = child.nextSibling
if (child is Text) {
if (child.wholeText?.trim().isNullOrEmpty()) {
node.removeChild(child)
}
}
child = nextChild
}
}
fun T.stripSpaces() = this.apply {
normalize()
stripSpacesImpl(this)
}
fun Node.evaluateXPathAsBoolean(xpathExpression: String) = newXPathExpression(xpathExpression).evaluateAsBoolean(this)
fun Node.evaluateXPathAsNumber(xpathExpression: String) = newXPathExpression(xpathExpression).evaluateAsNumber(this)
fun Node.evaluateXPathAsString(xpathExpression: String) = newXPathExpression(xpathExpression).evaluateAsString(this)
fun Node.evaluateXPathAsNodeList(xpathExpression: String) = newXPathExpression(xpathExpression).evaluateAsNodeList(this)
fun Node.evaluateXPathAsNode(xpathExpression: String) = newXPathExpression(xpathExpression).evaluateAsNode(this)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy