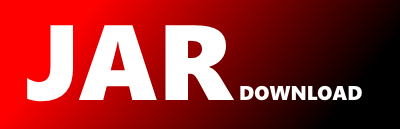
name.remal.annotation.AnnotationAttribute Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
Java & Kotlin tools: common
The newest version!
package name.remal.annotation;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.lang.annotation.Annotation;
import java.util.Objects;
import static name.remal.ArrayUtils.*;
class AnnotationAttribute {
@Nonnull
private final Class extends Annotation> annotationType;
@Nonnull
private final String name;
@Nullable
private final Object value;
public AnnotationAttribute(@Nonnull Class extends Annotation> annotationType, @Nonnull String name, @Nullable Object value) {
this.annotationType = annotationType;
this.name = name;
this.value = value;
}
@Nonnull
public Class extends Annotation> getAnnotationType() {
return annotationType;
}
@Nonnull
public String getName() {
return name;
}
@Nullable
public Object getValue() {
return value;
}
@Override
public boolean equals(Object object) {
if (this == object) return true;
if (!(object instanceof AnnotationAttribute)) return false;
AnnotationAttribute other = (AnnotationAttribute) object;
return Objects.equals(annotationType, other.annotationType)
&& Objects.equals(name, other.name)
&& arrayEquals(value, other.value);
}
@Override
public int hashCode() {
int result = 1;
result = 31 * result + annotationType.hashCode();
result = 31 * result + name.hashCode();
result = 31 * result + arrayHashCode(value);
return result;
}
@Nonnull
@Override
public String toString() {
return AnnotationAttribute.class.getSimpleName() + '('
+ "annotationType=" + annotationType
+ ", name='" + name + '\''
+ ", value=" + arrayToString(value)
+ '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy