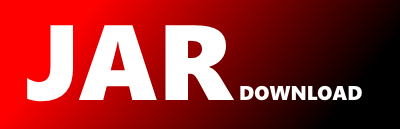
name.remal.java.lang.Class.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
Java & Kotlin tools: common
The newest version!
package name.remal
import name.remal.reflection.HierarchyUtils
import java.lang.reflect.Method
import java.lang.reflect.Modifier
import java.lang.reflect.Type
val Class<*>.resourceName get() = classNameToResourceName(name)
val Class<*>.isPublic get() = Modifier.isPublic(modifiers)
val Class<*>.isProtected get() = Modifier.isProtected(modifiers)
val Class<*>.isPackagePrivate get() = !Modifier.isPublic(modifiers) && !Modifier.isProtected(modifiers) && !Modifier.isPrivate(modifiers)
val Class<*>.isPrivate get() = Modifier.isPrivate(modifiers)
val Class<*>.isStatic get() = Modifier.isStatic(modifiers)
val Class<*>.isFinal get() = Modifier.isFinal(modifiers)
val Class<*>.isAbstract get() = Modifier.isAbstract(modifiers)
val Class<*>.isStrict get() = Modifier.isStrict(modifiers)
val Class<*>.hasSuperclass get() = superclass.let { null != it && this != it }
val Class<*>.packageName get() = name.substringBeforeLast('.', "")
val Class<*>.packageHierarchy: List get() = HierarchyUtils.getPackageHierarchy(this)
val Class.superClassHierarchy: List> get() = HierarchyUtils.getSuperClassesHierarchy(this)
val Class.hierarchy: List> get() = HierarchyUtils.getHierarchy(this)
val Class<*>.genericHierarchy: List get() = HierarchyUtils.getGenericHierarchy(this)
val Class<*>.allNotOverriddenMethods: List get() = HierarchyUtils.getAllNotOverriddenMethods(this)
fun Class<*>.unwrapPrimitive() = PrimitiveTypeUtils.unwrap(this)
fun Class<*>.wrapPrimitive() = PrimitiveTypeUtils.wrap(this)
fun Class<*>.compareByHierarchySize(other: Class<*>) = HierarchyUtils.compareByHierarchySize(this, other)
fun Class<*>.findField(name: String) = try {
getField(name)
} catch (ignored: NoSuchFieldException) {
null
}
fun Class<*>.findDeclaredField(name: String) = try {
getDeclaredField(name)
} catch (ignored: NoSuchFieldException) {
null
}
fun Class<*>.findConstructor(vararg parameterTypes: Class<*>) = try {
getConstructor(*parameterTypes)
} catch (ignored: NoSuchMethodException) {
null
}
fun Class<*>.findDeclaredConstructor(vararg parameterTypes: Class<*>) = try {
getDeclaredConstructor(*parameterTypes)
} catch (ignored: NoSuchMethodException) {
null
}
fun Class<*>.findMethod(name: String, vararg parameterTypes: Class<*>) = try {
getMethod(name, *parameterTypes)
} catch (ignored: NoSuchMethodException) {
null
}
fun Class<*>.findDeclaredMethod(name: String, vararg parameterTypes: Class<*>) = try {
getDeclaredMethod(name, *parameterTypes)
} catch (ignored: NoSuchMethodException) {
null
}