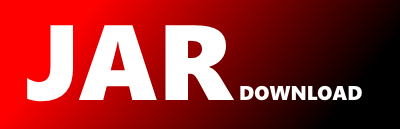
main.name.remal.gradle_plugins.plugins.generate_sources.GeneratingClassWriterInterface.kt Maven / Gradle / Ivy
package name.remal.gradle_plugins.plugins.generate_sources
import groovy.lang.Closure
import groovy.lang.Closure.DELEGATE_FIRST
import groovy.lang.DelegatesTo
import name.remal.gradle_plugins.dsl.extensions.toConfigureKotlinFunction
import java.io.Closeable
import java.io.StringWriter
import kotlin.reflect.KFunction
import kotlin.reflect.jvm.javaMethod
import name.remal.escapeJava as escapeJavaImpl
import name.remal.escapeRegex as escapeRegexImpl
interface GeneratingClassWriterInterface> : GeneratingWriterInterface {
val packageName: String
fun escapeJava(string: String) = escapeJavaImpl(string)
fun escapeRegex(string: String) = escapeRegexImpl(string)
fun writeSuppressWarnings(vararg warnings: String)
fun writeSuppressWarnings() = writeSuppressWarnings("all")
fun writePackage()
fun writeImport(canonicalClassName: String)
fun writeImport(clazz: Class<*>) = writeImport(clazz.canonicalName ?: clazz.name)
fun writeStaticImport(canonicalClassName: String, member: String)
fun writeStaticImport(clazz: Class<*>, member: String) = writeStaticImport(clazz.canonicalName ?: clazz.name, member)
fun writeStaticImport(kFunction: KFunction<*>) = kFunction.javaMethod.let {
if (it == null) throw IllegalArgumentException("$kFunction doesn't refer to Java method")
writeStaticImport(it.declaringClass, it.name)
}
fun writeBlock(expression: String, blockAction: Self.() -> Unit)
fun writeBlock(expression: String) = writeBlock(expression, {})
fun writeBlock(blockAction: Self.() -> Unit) = writeBlock("", blockAction)
fun writeBlock() = writeBlock("")
fun writeBlock(expression: String, @DelegatesTo(strategy = DELEGATE_FIRST) blockAction: Closure<*>) = writeBlock(expression, blockAction.toConfigureKotlinFunction())
fun writeBlock(@DelegatesTo(strategy = DELEGATE_FIRST) blockAction: Closure<*>) = writeBlock(blockAction.toConfigureKotlinFunction())
}
fun > GeneratingClassWriterInterface<*>.writeBlock(
wrapperFunc: (stringWriter: StringWriter) -> Self,
expression: String,
blockAction: Self.() -> Unit
) {
expression.trimEnd().let {
if (it.isNotBlank()) {
append(it).append(' ')
}
}
append('{')
val content = StringWriter()
.also { stringWriter ->
val wrapped = wrapperFunc(stringWriter)
try {
blockAction(wrapped)
} finally {
if (wrapped is Closeable) {
wrapped.close()
}
}
}
.toString().trimEnd()
if (content.isNotBlank()) {
append('\n')
content.split('\n').forEach { append(" ").append(it.trimEnd()).append('\n') }
}
append("}\n")
}
fun GeneratingClassWriterInterface<*>.writeBlock(
newSubWriterFunc: () -> Self,
expression: String,
blockAction: Self.() -> Unit
) where Self : GeneratingClassWriterInterface, Self : StringWriter {
expression.trimEnd().let {
if (it.isNotBlank()) {
append(it).append(' ')
}
}
append('{')
val content = newSubWriterFunc()
.also { it.use(blockAction) }
.toString().trimEnd()
if (content.isNotBlank()) {
append('\n')
content.split('\n').forEach { append(" ").append(it.trimEnd()).append('\n') }
}
append("}\n")
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy