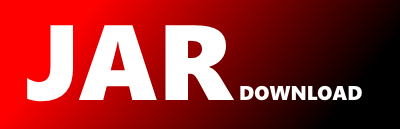
main.name.remal.gradle_plugins.plugins.vcs.VcsOperations.kt Maven / Gradle / Ivy
package name.remal.gradle_plugins.plugins.vcs
import name.remal.default
import name.remal.firstOrNull
import name.remal.gradle_plugins.dsl.utils.registerIfCloseable
import name.remal.gradle_plugins.plugins.vcs.VcsOperationsCustomizer.Companion.VCS_OPERATIONS_CUSTOMIZERS
import name.remal.loadServicesList
import org.gradle.api.Project
import java.io.File
import java.net.URI
import java.util.stream.Stream
abstract class VcsOperations {
companion object {
private val vcsOperationsFactories = loadServicesList(VcsOperationsFactory::class.java)
private fun createVcsOperationsImpl(dir: File): VcsOperations? {
val absoluteDir = dir.absoluteFile
vcsOperationsFactories.forEach { factory ->
factory.get(absoluteDir)?.let { vcsOperations ->
VCS_OPERATIONS_CUSTOMIZERS.forEach { it.customize(vcsOperations) }
return registerIfCloseable(vcsOperations)
}
}
return null
}
@JvmStatic
fun createVcsOperations(dir: File): VcsOperations {
val absoluteDir = dir.absoluteFile
return createVcsOperationsImpl(absoluteDir) ?: VcsOperationsUnsupported(absoluteDir)
}
@JvmStatic
fun createVcsOperations(project: Project): VcsOperations {
return createVcsOperationsImpl(project.projectDir) ?: VcsOperationsUnsupported(project)
}
private val BRANCH_ESCAPING_REGEX = Regex("\\W")
@JvmStatic
fun createBranchSlug(branch: String): String {
return BRANCH_ESCAPING_REGEX.replace(branch, "-")
.let { if (63 < it.length) it.substring(0, 63) else it }
.trim('-')
.toLowerCase()
}
}
abstract val vcsRootDir: File
open var overwriteMasterBranch: String? = null
protected abstract val trueMasterBranch: String
val masterBranch: String get() = overwriteMasterBranch ?: trueMasterBranch
open var overwriteCurrentBranch: String? = null
protected abstract val trueCurrentBranch: String?
val currentBranch: String? get() = overwriteCurrentBranch ?: trueCurrentBranch
abstract val isCommitted: Boolean
val isNotCommitted: Boolean get() = !isCommitted
abstract var commitAuthor: CommitAuthor?
abstract fun walkCommits(): Stream
open fun getCurrentCommit() = walkCommits().firstOrNull()
abstract fun commitFiles(message: String, files: Collection)
fun commitFiles(message: String, vararg files: File) = commitFiles(message, files.toList())
protected open fun pathsToFiles(filePaths: Collection): Collection = filePaths.map(::File)
fun commit(message: String, filePaths: Collection) = commitFiles(message, pathsToFiles(filePaths))
fun commit(message: String, vararg filePaths: String) = commit(message, filePaths.toList())
fun commit(message: String) = commitFiles(message, emptyList())
abstract fun getAllTagNames(): Set
abstract fun createTag(commitId: String, tagName: String, message: String)
fun createTag(commitId: String, tagName: String) = createTag(commitId, tagName, "")
fun createTag(commit: Commit, tagName: String, message: String) = createTag(commit.id, tagName, message)
fun createTag(commit: Commit, tagName: String) = createTag(commit, tagName, "")
fun createTagForCurrentCommit(tagName: String, message: String) = createTag(getCurrentCommit()?.id.default(), tagName, message)
fun createTagForCurrentCommit(tagName: String) = createTagForCurrentCommit(tagName, "")
abstract fun findTagWithDepth(predicate: (tagName: String) -> Boolean): TagsWithDepth?
abstract fun setUnauthorizedRemoteURI(uri: String)
fun setUnauthorizedRemoteURI(uri: URI) = setUnauthorizedRemoteURI(uri.toString())
abstract fun setUsernamePasswordAuth(username: String, password: CharArray)
fun setUsernamePasswordAuth(username: String, password: String) = setUsernamePasswordAuth(username, password.toCharArray())
fun setUsernamePasswordAuth(username: String) = setUsernamePasswordAuth(username, charArrayOf())
abstract fun setSSHAuth(privateKeyFile: File, password: CharArray?)
fun setSSHAuth(privateKeyFile: File, password: String?) = setSSHAuth(privateKeyFile, password?.toCharArray())
fun setSSHAuth(privateKeyFile: File) = setSSHAuth(privateKeyFile, null as CharArray?)
fun setRemoteURI(uri: String, vcsAuth: AbstractVcsAuth) {
setUnauthorizedRemoteURI(uri)
when (vcsAuth) {
is UsernamePasswordVcsAuth -> setUsernamePasswordAuth(vcsAuth.username, vcsAuth.password)
is SSHVcsAuth -> setSSHAuth(vcsAuth.privateKeyFile, vcsAuth.password)
}
}
fun setRemoteURI(uri: URI, vcsAuth: AbstractVcsAuth) = setRemoteURI(uri.toString(), vcsAuth)
fun calculateCacheKey() = VcsCacheKey(getCurrentCommit(), currentBranch, masterBranch, getAllTagNames())
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy