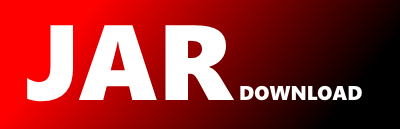
name.valery1707.validator.russian.inn.InnInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of russian-requisites-validator Show documentation
Show all versions of russian-requisites-validator Show documentation
Javax validation implementation for Russian requisites: ИНН, КПП, ОГРН
The newest version!
package name.valery1707.validator.russian.inn;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import static name.valery1707.validator.Checker.checkRange;
@SuppressWarnings("WeakerAccess")
public class InnInfo {
/**
* Код субъекта Российской Федерации согласно ст. 65 Конституции
*/
private final byte subject;
/**
* Номер местной налоговой инспекции
*/
private final byte tax;
/**
* Номер налоговой записи налогоплательщика
*/
private final int id;
/**
* Юридическое Лицо/Физическое лицо
*/
private final boolean juridical;
private transient String crc;
/**
* ИНН
*
* @param subject Код субъекта Российской Федерации
* @param tax Номер местной налоговой инспекции
* @param id Номер налоговой записи налогоплательщика
* @param juridical Юридическое Лицо/Физическое лицо
*/
public InnInfo(byte subject, byte tax, int id, boolean juridical) {
this.subject = checkRange(subject, 0, 99, "Subject");
this.tax = checkRange(tax, 0, 99, "Local tax");
this.id = checkRange(id, 0, juridical ? 99999 : 999999, "ID");
this.juridical = juridical;
}
/**
* ИНН
*
* @param subject Код субъекта Российской Федерации
* @param tax Номер местной налоговой инспекции
* @param id Номер налоговой записи налогоплательщика
* @param juridical Юридическое Лицо/Физическое лицо
*/
public InnInfo(int subject, int tax, int id, boolean juridical) {
this((byte) subject, (byte) tax, id, juridical);
}
@Nonnull
public static InnInfo parse(@Nullable String value) {
if (InnValidator.isValid(value).nonValid()) {
throw new IllegalArgumentException("Can not parse INN from string: " + value);
}
assert value != null;
boolean juridical = value.length() == 10;
byte subject = Byte.valueOf(value.substring(0, 2));
byte localTax = Byte.valueOf(value.substring(2, 4));
int id = Integer.valueOf(value.substring(4, value.length() - (juridical ? 1 : 2)));
return new InnInfo(subject, localTax, id, juridical);
}
public String format() {
String s = String.format(
isJuridical()
? "%02d%02d%05d"
: "%02d%02d%06d"
, getSubject(), getTax(), getId());
if (crc == null) {
crc = String.valueOf(InnValidator.calcCrc(s));
if (isPhysical()) {
crc += String.valueOf(InnValidator.calcCrc(s + crc));
}
}
return s + crc;
}
/**
* @return Код субъекта Российской Федерации
*/
public byte getSubject() {
return subject;
}
/**
* @return Номер местной налоговой инспекции
*/
public byte getTax() {
return tax;
}
/**
* @return Номер налоговой записи налогоплательщика
*/
public int getId() {
return id;
}
/**
* @return Юридическое Лицо
*/
public boolean isJuridical() {
return juridical;
}
/**
* @return Физическое лицо
*/
public boolean isPhysical() {
return !isJuridical();
}
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (!(other instanceof InnInfo)) {
return false;
}
InnInfo that = (InnInfo) other;
return
this.getSubject() == that.getSubject() &&
this.getTax() == that.getTax() &&
this.getId() == that.getId() &&
this.isJuridical() == that.isJuridical()
;
}
@Override
public int hashCode() {
int result = (int) getSubject();
result = 31 * result + (int) getTax();
result = 31 * result + getId();
result = 31 * result + (isJuridical() ? 1 : 0);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy