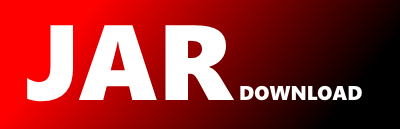
net.abstractfactory.plum.view.web.PlumForm Maven / Gradle / Ivy
package net.abstractfactory.plum.view.web;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import net.abstractfactory.common.ListValueMap;
import org.apache.log4j.Logger;
/**
* populate PLUM specific HTML FORM elements.
*
* @author jack
*
*/
public class PlumForm {
private Logger logger = Logger.getLogger(getClass());
public static final String SPECIAL_PARAMETER_PREFIX = "_plum_";
static final String COMPONENT = SPECIAL_PARAMETER_PREFIX + "component";
static final String TAG = SPECIAL_PARAMETER_PREFIX + "tag";
static final String EVENT = SPECIAL_PARAMETER_PREFIX + "event";
public static final String EVENT_PARAMETER = SPECIAL_PARAMETER_PREFIX + "event_parameter";
public static final String VIEW_VERSION = SPECIAL_PARAMETER_PREFIX + "view_version";
static Set specialParameterNames = new HashSet();
static {
specialParameterNames.add(COMPONENT);
specialParameterNames.add(TAG);
specialParameterNames.add(EVENT);
specialParameterNames.add(EVENT_PARAMETER);
specialParameterNames.add(VIEW_VERSION);
}
private Map specialParameters = new HashMap();
public PlumForm(ListValueMap parameterMap) {
processComponentInputs(parameterMap);
}
// group by web component
private Map> componentParameters;
private void processComponentInputs(ListValueMap parameterMap) {
componentParameters = new HashMap>();
for (String name : parameterMap.keySet()) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy