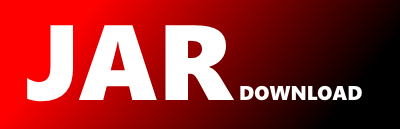
net.abstractfactory.plum.view.web.layout.WebGridLayout Maven / Gradle / Ivy
package net.abstractfactory.plum.view.web.layout;
import java.util.List;
import net.abstractfactory.plum.view.component.Component;
import net.abstractfactory.plum.view.layout.GridLayout;
import net.abstractfactory.plum.view.web.component.AbstractWebComponent;
import org.jsoup.nodes.Element;
import org.jsoup.parser.Tag;
public class WebGridLayout extends AbstractWebComponent {
Element table;
Element head;
Element body;
Element currentTR;
List trList;
public WebGridLayout(String id, Component component) {
super(id, component);
}
private GridLayout getGridLayout() {
return (GridLayout) component;
}
@Override
public void createHtmlElement() {
table = new Element(Tag.valueOf("table"), "");
table.attr("class", "table");
if (getGridLayout().isHeaderEnabled()) {
// head
head = new Element(Tag.valueOf("thead"), "");
table.appendChild(head);
currentTR = new Element(Tag.valueOf("tr"), "");
head.appendChild(currentTR);
// body
body = new Element(Tag.valueOf("tbody"), "");
table.appendChild(body);
} else {
// body
body = new Element(Tag.valueOf("tbody"), "");
table.appendChild(body);
currentTR = new Element(Tag.valueOf("tr"), "");
body.appendChild(currentTR);
}
htmlOuterElement = table;
}
private Element createCellElement(int rowIndex) {
Element cell;
if (rowIndex == 0 && getGridLayout().isHeaderEnabled())
cell = new Element(Tag.valueOf("th"), "");
else
cell = new Element(Tag.valueOf("td"), "");
return cell;
}
private Element getCurrentContainer(int rowIndex) {
Element container;
if (rowIndex == 0 && getGridLayout().isHeaderEnabled())
container = head;
else
container = body;
return container;
}
@Override
protected Element getHtmlInnerElementForNewChild() {
GridLayout grid = getGridLayout();
int currentChildSize = getChildren().size();
int currentRowIndex = (currentChildSize - 1) / grid.getCols();
// int currentCols = currentChildSize % grid.getCols();
// next child
int nextChildSize = currentChildSize + 1;
int nextRowIndex = (nextChildSize - 1) / grid.getCols();
// int nextCols = nextChildSize % grid.getCols();
if (nextRowIndex > currentRowIndex) {
// create new row
currentTR = new Element(Tag.valueOf("tr"), "");
getCurrentContainer(nextRowIndex).appendChild(currentTR);
}
Element cell = createCellElement(nextRowIndex);
currentTR.appendChild(cell);
return cell;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy