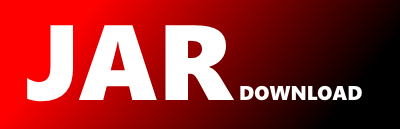
net.accelbyte.sdk.api.lobby.wrappers.Player Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of module-lobby Show documentation
Show all versions of module-lobby Show documentation
AccelByte Gaming Services Java Extend SDK generated from OpenAPI specs
/*
* Copyright (c) 2024 AccelByte Inc. All Rights Reserved
* This is licensed software from AccelByte Inc, for limitations
* and restrictions contact your company contract manager.
*
* Code generated. DO NOT EDIT.
*/
package net.accelbyte.sdk.api.lobby.wrappers;
import net.accelbyte.sdk.api.lobby.models.*;
import net.accelbyte.sdk.api.lobby.operations.player.*;
import net.accelbyte.sdk.core.HttpResponse;
import net.accelbyte.sdk.core.RequestRunner;
public class Player {
private RequestRunner sdk;
private String customBasePath = "";
public Player(RequestRunner sdk) {
this.sdk = sdk;
String configCustomBasePath =
sdk.getSdkConfiguration().getConfigRepository().getCustomServiceBasePath("lobby");
if (!configCustomBasePath.equals("")) {
this.customBasePath = configCustomBasePath;
}
}
public Player(RequestRunner sdk, String customBasePath) {
this.sdk = sdk;
this.customBasePath = customBasePath;
}
/**
* @see AdminGetLobbyCCU
*/
public ModelsGetLobbyCcuResponse adminGetLobbyCCU(AdminGetLobbyCCU input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see AdminGetBulkPlayerBlockedPlayersV1
*/
public ModelsGetBulkAllPlayerBlockedUsersResponse adminGetBulkPlayerBlockedPlayersV1(
AdminGetBulkPlayerBlockedPlayersV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see AdminGetAllPlayerSessionAttribute
*/
public ModelsGetAllPlayerSessionAttributeResponse adminGetAllPlayerSessionAttribute(
AdminGetAllPlayerSessionAttribute input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see AdminSetPlayerSessionAttribute
*/
public void adminSetPlayerSessionAttribute(AdminSetPlayerSessionAttribute input)
throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see AdminGetPlayerSessionAttribute
*/
public ModelsGetPlayerSessionAttributeResponse adminGetPlayerSessionAttribute(
AdminGetPlayerSessionAttribute input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see AdminGetPlayerBlockedPlayersV1
*/
public ModelsGetAllPlayerBlockedUsersResponse adminGetPlayerBlockedPlayersV1(
AdminGetPlayerBlockedPlayersV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see AdminGetPlayerBlockedByPlayersV1
*/
public ModelsGetAllPlayerBlockedByUsersResponse adminGetPlayerBlockedByPlayersV1(
AdminGetPlayerBlockedByPlayersV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see AdminBulkBlockPlayersV1
*/
public void adminBulkBlockPlayersV1(AdminBulkBlockPlayersV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see AdminBulkUnblockPlayersV1
*/
public void adminBulkUnblockPlayersV1(AdminBulkUnblockPlayersV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPlayerBlockPlayersV1
*/
public void publicPlayerBlockPlayersV1(PublicPlayerBlockPlayersV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicGetPlayerBlockedPlayersV1
*/
public ModelsGetAllPlayerBlockedUsersResponse publicGetPlayerBlockedPlayersV1(
PublicGetPlayerBlockedPlayersV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicGetPlayerBlockedByPlayersV1
*/
public ModelsGetAllPlayerBlockedByUsersResponse publicGetPlayerBlockedByPlayersV1(
PublicGetPlayerBlockedByPlayersV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicUnblockPlayerV1
*/
public void publicUnblockPlayerV1(PublicUnblockPlayerV1 input) throws Exception {
if (input.getCustomBasePath().equals("") && !customBasePath.equals("")) {
input.setCustomBasePath(customBasePath);
}
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy