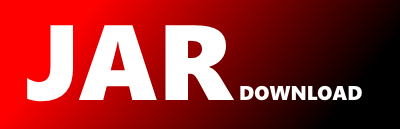
net.accelbyte.sdk.api.platform.models.AppUpdate Maven / Gradle / Ivy
/*
* Copyright (c) 2022 AccelByte Inc. All Rights Reserved
* This is licensed software from AccelByte Inc, for limitations
* and restrictions contact your company contract manager.
*
* Code generated. DO NOT EDIT.
*/
package net.accelbyte.sdk.api.platform.models;
import com.fasterxml.jackson.annotation.*;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.*;
import lombok.*;
import net.accelbyte.sdk.core.Model;
@JsonIgnoreProperties(ignoreUnknown = true)
@Builder
@Getter
@Setter
// @deprecated 2022-08-29 - All args constructor may cause problems. Use builder instead.
@AllArgsConstructor(onConstructor = @__(@Deprecated))
@NoArgsConstructor
public class AppUpdate extends Model {
@JsonProperty("carousel")
@JsonInclude(JsonInclude.Include.NON_NULL)
private List carousel;
@JsonProperty("developer")
@JsonInclude(JsonInclude.Include.NON_NULL)
private String developer;
@JsonProperty("forumUrl")
@JsonInclude(JsonInclude.Include.NON_NULL)
private String forumUrl;
@JsonProperty("genres")
@JsonInclude(JsonInclude.Include.NON_NULL)
private List genres;
@JsonProperty("localizations")
@JsonInclude(JsonInclude.Include.NON_NULL)
private Map localizations;
@JsonProperty("platformRequirements")
@JsonInclude(JsonInclude.Include.NON_NULL)
private Map> platformRequirements;
@JsonProperty("platforms")
@JsonInclude(JsonInclude.Include.NON_NULL)
private List platforms;
@JsonProperty("players")
@JsonInclude(JsonInclude.Include.NON_NULL)
private List players;
@JsonProperty("primaryGenre")
@JsonInclude(JsonInclude.Include.NON_NULL)
private String primaryGenre;
@JsonProperty("publisher")
@JsonInclude(JsonInclude.Include.NON_NULL)
private String publisher;
@JsonProperty("releaseDate")
@JsonInclude(JsonInclude.Include.NON_NULL)
private String releaseDate;
@JsonProperty("websiteUrl")
@JsonInclude(JsonInclude.Include.NON_NULL)
private String websiteUrl;
@JsonIgnore
public List getGenres() {
return this.genres;
}
@JsonIgnore
public List getGenresAsEnum() {
ArrayList en = new ArrayList();
for (String e : this.genres) en.add(Genres.valueOf(e));
return en;
}
@JsonIgnore
public void setGenres(final List genres) {
this.genres = genres;
}
@JsonIgnore
public void setGenresFromEnum(final List genres) {
ArrayList en = new ArrayList();
for (Genres e : genres) en.add(e.toString());
this.genres = en;
}
@JsonIgnore
public List getPlatforms() {
return this.platforms;
}
@JsonIgnore
public List getPlatformsAsEnum() {
ArrayList en = new ArrayList();
for (String e : this.platforms) en.add(Platforms.valueOf(e));
return en;
}
@JsonIgnore
public void setPlatforms(final List platforms) {
this.platforms = platforms;
}
@JsonIgnore
public void setPlatformsFromEnum(final List platforms) {
ArrayList en = new ArrayList();
for (Platforms e : platforms) en.add(e.toString());
this.platforms = en;
}
@JsonIgnore
public List getPlayers() {
return this.players;
}
@JsonIgnore
public List getPlayersAsEnum() {
ArrayList en = new ArrayList();
for (String e : this.players) en.add(Players.valueOf(e));
return en;
}
@JsonIgnore
public void setPlayers(final List players) {
this.players = players;
}
@JsonIgnore
public void setPlayersFromEnum(final List players) {
ArrayList en = new ArrayList();
for (Players e : players) en.add(e.toString());
this.players = en;
}
@JsonIgnore
public String getPrimaryGenre() {
return this.primaryGenre;
}
@JsonIgnore
public PrimaryGenre getPrimaryGenreAsEnum() {
return PrimaryGenre.valueOf(this.primaryGenre);
}
@JsonIgnore
public void setPrimaryGenre(final String primaryGenre) {
this.primaryGenre = primaryGenre;
}
@JsonIgnore
public void setPrimaryGenreFromEnum(final PrimaryGenre primaryGenre) {
this.primaryGenre = primaryGenre.toString();
}
@JsonIgnore
public AppUpdate createFromJson(String json) throws JsonProcessingException {
return new ObjectMapper().readValue(json, this.getClass());
}
@JsonIgnore
public List createFromJsonList(String json) throws JsonProcessingException {
return new ObjectMapper().readValue(json, new TypeReference>() {});
}
public enum Genres {
Action("Action"),
Adventure("Adventure"),
Casual("Casual"),
FreeToPlay("FreeToPlay"),
Indie("Indie"),
MassivelyMultiplayer("MassivelyMultiplayer"),
RPG("RPG"),
Racing("Racing"),
Simulation("Simulation"),
Sports("Sports"),
Strategy("Strategy");
private String value;
Genres(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
}
public enum Platforms {
Android("Android"),
IOS("IOS"),
Linux("Linux"),
MacOS("MacOS"),
Windows("Windows");
private String value;
Platforms(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
}
public enum Players {
Coop("Coop"),
CrossPlatformMulti("CrossPlatformMulti"),
LocalCoop("LocalCoop"),
MMO("MMO"),
Multi("Multi"),
Single("Single");
private String value;
Players(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
}
public enum PrimaryGenre {
Action("Action"),
Adventure("Adventure"),
Casual("Casual"),
FreeToPlay("FreeToPlay"),
Indie("Indie"),
MassivelyMultiplayer("MassivelyMultiplayer"),
RPG("RPG"),
Racing("Racing"),
Simulation("Simulation"),
Sports("Sports"),
Strategy("Strategy");
private String value;
PrimaryGenre(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
}
public static class AppUpdateBuilder {
private List genres;
private List platforms;
private List players;
private String primaryGenre;
public AppUpdateBuilder genres(final List genres) {
this.genres = genres;
return this;
}
public AppUpdateBuilder genresFromEnum(final List genres) {
ArrayList en = new ArrayList();
for (Genres e : genres) en.add(e.toString());
this.genres = en;
return this;
}
public AppUpdateBuilder platforms(final List platforms) {
this.platforms = platforms;
return this;
}
public AppUpdateBuilder platformsFromEnum(final List platforms) {
ArrayList en = new ArrayList();
for (Platforms e : platforms) en.add(e.toString());
this.platforms = en;
return this;
}
public AppUpdateBuilder players(final List players) {
this.players = players;
return this;
}
public AppUpdateBuilder playersFromEnum(final List players) {
ArrayList en = new ArrayList();
for (Players e : players) en.add(e.toString());
this.players = en;
return this;
}
public AppUpdateBuilder primaryGenre(final String primaryGenre) {
this.primaryGenre = primaryGenre;
return this;
}
public AppUpdateBuilder primaryGenreFromEnum(final PrimaryGenre primaryGenre) {
this.primaryGenre = primaryGenre.toString();
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy