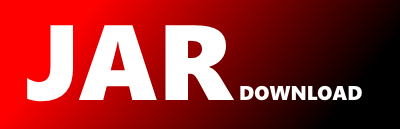
net.accelbyte.sdk.api.session.wrappers.Party Maven / Gradle / Ivy
/*
* Copyright (c) 2022 AccelByte Inc. All Rights Reserved
* This is licensed software from AccelByte Inc, for limitations
* and restrictions contact your company contract manager.
*
* Code generated. DO NOT EDIT.
*/
package net.accelbyte.sdk.api.session.wrappers;
import net.accelbyte.sdk.api.session.models.*;
import net.accelbyte.sdk.api.session.operations.party.*;
import net.accelbyte.sdk.core.AccelByteSDK;
import net.accelbyte.sdk.core.HttpResponse;
public class Party {
private AccelByteSDK sdk;
public Party(AccelByteSDK sdk) {
this.sdk = sdk;
}
/**
* @see AdminQueryParties
*/
public ApimodelsPartyQueryResponse adminQueryParties(AdminQueryParties input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPartyJoinCode
*/
public ApimodelsPartySessionResponse publicPartyJoinCode(PublicPartyJoinCode input)
throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicGetParty
*/
public ApimodelsPartySessionResponse publicGetParty(PublicGetParty input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicUpdateParty
*/
public ApimodelsPartySessionResponse publicUpdateParty(PublicUpdateParty input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPatchUpdateParty
*/
public ApimodelsPartySessionResponse publicPatchUpdateParty(PublicPatchUpdateParty input)
throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicGeneratePartyCode
*/
public ApimodelsPartySessionResponse publicGeneratePartyCode(PublicGeneratePartyCode input)
throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicRevokePartyCode
*/
public void publicRevokePartyCode(PublicRevokePartyCode input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPartyInvite
*/
public void publicPartyInvite(PublicPartyInvite input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPromotePartyLeader
*/
public ApimodelsPartySessionResponse publicPromotePartyLeader(PublicPromotePartyLeader input)
throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPartyJoin
*/
public ApimodelsPartySessionResponse publicPartyJoin(PublicPartyJoin input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPartyLeave
*/
public void publicPartyLeave(PublicPartyLeave input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPartyReject
*/
public void publicPartyReject(PublicPartyReject input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
input.handleEmptyResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicPartyKick
*/
public ApimodelsKickResponse publicPartyKick(PublicPartyKick input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicCreateParty
*/
public ApimodelsPartySessionResponse publicCreateParty(PublicCreateParty input) throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
/**
* @see PublicQueryMyParties
*/
public ApimodelsPartyQueryResponse publicQueryMyParties(PublicQueryMyParties input)
throws Exception {
final HttpResponse httpResponse = sdk.runRequest(input);
return input.parseResponse(
httpResponse.getCode(), httpResponse.getContentType(), httpResponse.getPayload());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy