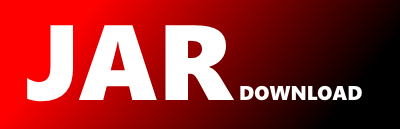
net.adamcin.httpsig.api.DefaultKeychain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of httpsig-api Show documentation
Show all versions of httpsig-api Show documentation
Library providing SSHKey API
package net.adamcin.httpsig.api;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Set;
/**
* Simple implementation of {@link net.adamcin.httpsig.api.Keychain} backed by a HashMap and
* modified via {@link Collection} methods
*/
public class DefaultKeychain implements Keychain, Collection {
private final List keys = new ArrayList();
private final Set _algorithms = new LinkedHashSet();
public DefaultKeychain() {
this(null);
}
public DefaultKeychain(Collection extends Key> identities) {
if (identities != null) {
this.addAll(identities);
}
}
public Set getAlgorithms() {
return Collections.unmodifiableSet(_algorithms);
}
public int size() {
return keys.size();
}
public boolean isEmpty() {
return keys.isEmpty();
}
public boolean contains(Object o) {
return keys.contains(o);
}
public Iterator iterator() {
return keys.iterator();
}
public Object[] toArray() {
return keys.toArray();
}
public T[] toArray(T[] a) {
return keys.toArray(a);
}
public boolean add(Key key) {
_algorithms.addAll(key.getAlgorithms());
return keys.add(key);
}
public boolean remove(Object o) {
return keys.remove(o);
}
public boolean containsAll(Collection> c) {
return keys.containsAll(c);
}
public boolean addAll(Collection extends Key> c) {
boolean changed = false;
if (c != null) {
for (Key i : c) {
if (add(i)) {
changed = true;
}
}
}
return changed;
}
public boolean removeAll(Collection> c) {
return keys.removeAll(c);
}
public boolean retainAll(Collection> c) {
return keys.retainAll(c);
}
public void clear() {
keys.clear();
_algorithms.clear();
}
public Keychain discard() {
if (isEmpty()) {
throw new NoSuchElementException("keychain is empty");
} else if (size() > 1) {
List _keys = new ArrayList(keys.size() - 1);
Iterator _idents = iterator();
_idents.next();
while (_idents.hasNext()) {
_keys.add(_idents.next());
}
return new DefaultKeychain(_keys);
} else {
return new DefaultKeychain();
}
}
/**
*
* @return
* @throws NoSuchElementException if the keychain is empty
*/
public Key currentKey() {
if (isEmpty()) {
throw new NoSuchElementException("keychain is empty");
} else {
return iterator().next();
}
}
public Keychain filterAlgorithms(Collection algorithms) {
ArrayList filteredKeys = new ArrayList();
for (Key key : this) {
for (Algorithm algorithm : key.getAlgorithms()) {
if (algorithms.contains(algorithm)) {
filteredKeys.add(key);
}
}
}
return new DefaultKeychain(filteredKeys);
}
public Map toMap(KeyId keyIdentifier) {
KeyId identifier = keyIdentifier != null ? keyIdentifier : Constants.DEFAULT_KEY_IDENTIFIER;
LinkedHashMap map = new LinkedHashMap(this.size());
for (Key key : this) {
String keyId = identifier.getId(key);
if (keyId != null) {
map.put(keyId, key);
}
}
return Collections.unmodifiableMap(map);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy