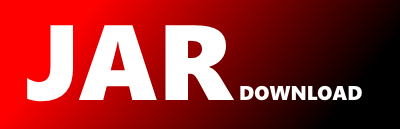
net.adamcin.oakpal.core.OrgJson Maven / Gradle / Ivy
/*
* Copyright 2018 Mark Adamcin
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.adamcin.oakpal.core;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import org.json.JSONArray;
import org.json.JSONObject;
/**
* Simple DSL for constructing org.json objects for {@link ProgressCheckFactory} configs using only three-letter identifiers.
*
* Recommend using {@code import static net.adamcin.oakpal.core.OrgJson.*;}
*/
public final class OrgJson {
private OrgJson() {
// no construction
}
/**
* Custom pojo types which should be usable within this DSL should implement this method to provide a
* {@link JSONObject}, which can be wrapped quickly by {@link #val(Object)}.
*/
public interface ObjectConvertible {
JSONObject toJSON();
}
/**
* Custom pojo types which should be usable within this DSL should implement this method to provide a
* {@link JSONArray}, which can be wrapped quickly by {@link #val(Object)}.
*/
public interface ArrayConvertible {
JSONArray toJSON();
}
/**
* Defines a method toValue which coalesces the underlying value to prevent over-wrapping by the
* {@link #val(Object)} method.
*/
public interface HasValue {
Value toValue();
}
/**
* Defines a get method returns the constructed type parameter.
*
* @param the return type of the get method
*/
public interface As {
TYPE get();
}
/**
* Type which allows a different fluent style for building keys, i.e. {@code key(String).val(obj)}.
*/
public interface Cursor {
String getKey();
}
/**
* Ensures that an object reference is wrapped appropriately for use in the DSL.
*
* @param value null, an instance of {@link HasValue}, or some type supported by {@link JSONObject#wrap(Object)}.
* @return a DSL-wrapped value
*/
public static Value val(Object value) {
if (value instanceof Cursor) {
throw new IllegalArgumentException("dangling cursor for key: " + ((Cursor) value).getKey());
} else if (value instanceof ObjectConvertible) {
return val(((ObjectConvertible) value).toJSON());
} else if (value instanceof ArrayConvertible) {
return val(((ArrayConvertible) value).toJSON());
} else if (value instanceof HasValue) {
return ((HasValue) value).toValue();
} else {
return new Value(value);
}
}
/**
* Create an object with the provided key-value pairs.
*
* @param keys 0-many key-value pairs
* @return a new obj
*/
public static Obj obj(final Key... keys) {
return new Obj(keys);
}
/**
* Create an object, and add all the keys from the provided json object to it.
*
* @param jsonObject a starting point
* @return a new obj
*/
public static Obj obj(final JSONObject jsonObject) {
if (jsonObject != null) {
return obj(jsonObject.toMap());
} else {
return new Obj();
}
}
/**
* Create an object, and add all the keys from the provided map to it.
*
* @param map a starting point
* @return a new obj
*/
public static Obj obj(final Map, ?> map) {
return new Obj().and(map);
}
/**
* Creates a key-value pair for use with {@link #obj(Key...)}. See also {@link Obj#key(String, Object)}.
*
* @param key the key
* @param value the value
* @return a key-value pair.
*/
public static Key key(final String key, final Object value) {
return new Key(key, value);
}
/**
* Begin a key-value pair without providing a value argument. {@code val(Object)} must be called to provide a value
* for the key-value pair before more keys are appended.
*
* @param key the key
* @return a cursor waiting for a value
*/
public static KeyCursor key(final String key) {
return new KeyCursor(key);
}
/**
* Creates an array with the provided values.
*
* @param values the value elements of the array
* @return a new array
*/
public static Arr arr(Object... values) {
if (values != null) {
return new Arr(Arrays.asList(values));
} else {
return new Arr(Collections.emptyList());
}
}
/**
* Discrete value wrapper. Delegates to {@link JSONObject#wrap(Object)} for null-handling, etc.
*/
public static final class Value implements HasValue, As