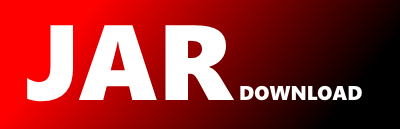
net.aequologica.neo.buildhub.persist.model.Build Maven / Gradle / Ivy
package net.aequologica.neo.buildhub.persist.model;
import static javax.persistence.TemporalType.TIMESTAMP;
import java.util.Date;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Temporal;
@Entity
@Table(name="BUILD")
/*
CREATE COLUMN TABLE "NEO_AUWAES2EZOZRTXVG15Z9MQ8C7"."BUILD" (
"ID" VARCHAR(36) CS_STRING NOT NULL,
"CALENDAR_WEEK" INT CS_INT,
"DURATION" BIGINT CS_FIXED,
"END_TIME" LONGDATE CS_LONGDATE,
"GAV" VARCHAR(175) CS_STRING,
"GOALS" VARCHAR(1024) CS_STRING,
"HOST" VARCHAR(80) CS_STRING,
"START_TIME" LONGDATE CS_LONGDATE,
"STATUS" VARCHAR(50) CS_STRING,
PRIMARY KEY ( "ID" ) )
*/
public class Build {
/*
http://stackoverflow.com/questions/13397038/uuid-max-character-length
Section 3 of RFC4122 provides the formal definition of UUID string representations. It's 36 characters - 32 hex digits + 4 dashes.
http://www.ietf.org/rfc/rfc4122.txt
*/
@Id
@Column(name = "ID", length = 36) private String id;
@Column(name = "GAV", length = 175) private String gav;
@Column(name = "GOALS", length = 1024) private String goals;
@Column(name = "HOST", length = 80) private String host;
@Column(name = "DURATION") private long duration;
@Column(name = "START_TIME") @Temporal(TIMESTAMP) private Date start;
@Column(name = "END_TIME") @Temporal(TIMESTAMP) private Date end;
@Column(name = "STATUS", length = 50) private String status;
@Column(name = "CALENDAR_WEEK") private int week;
public Build() {
}
public String getId() {
return id;
}
public void setBuildId(String buildId) {
this.id = buildId;
}
public String getGav() {
return gav;
}
public void setGav(String gav) {
this.gav = gav;
}
public String getGoals() {
return goals;
}
public void setGoals(String goals) {
this.goals = goals;
}
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public long getDuration() {
return duration;
}
public void setDuration(long duration) {
this.duration = duration;
}
public Date getStart() {
return start;
}
public void setStart(Date start) {
this.start = start;
}
public Date getEnd() {
return end;
}
public void setEnd(Date end) {
this.end = end;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public int getWeek() {
return week;
}
public void setWeek(int calendarWeek) {
this.week = calendarWeek;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy