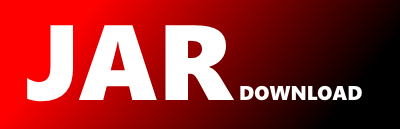
net.aequologica.neo.shakuntala.AbstractEventSpyImpl Maven / Gradle / Ivy
package net.aequologica.neo.shakuntala;
import static java.lang.Runtime.getRuntime;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.atomic.AtomicBoolean;
import javax.inject.Inject;
import javax.inject.Named;
import org.apache.maven.eventspy.EventSpy;
import net.aequologica.neo.shakuntala.Event.Level;
public abstract class AbstractEventSpyImpl implements EventSpy {
final private AtomicBoolean closed = new AtomicBoolean(false);
@Inject
private List writers;
@Inject
@Named("application.conf")
protected EventSpyConfig config;
private final Dispatcher dispatcher = new Dispatcher();
@Override
public void init(final Context context) throws Exception {
getRuntime().addShutdownHook(new Thread(new Runnable() {
@Override
public void run() {
try {
close();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}));
Constants.main(null);
dispatcher.init();
}
@Override
public void onEvent(Object object) throws Exception {
if (object == null) {
return;
}
String key = object.getClass().getSimpleName();
String value = object.toString();
dispatcher.write(key, value, null, object);
}
protected void dispatch(String key, String value, Level level, Object object) throws Exception {
if (this.dispatcher == null) {
return;
}
dispatcher.write(key, value, level, object);
}
@Override
public void close() throws Exception {
synchronized (closed) {
if (closed.get() == false) {
dispatcher.close();
closed.set(true);
}
}
}
private class Dispatcher implements EventWriter {
@Override
public void init() throws IOException {
if (AbstractEventSpyImpl.this.writers == null) {
return;
}
for (EventWriter writer : AbstractEventSpyImpl.this.writers) {
if (writer == null) {
continue;
}
writer.init();
}
}
@Override
public void write(Event event) throws IOException {
if (AbstractEventSpyImpl.this.writers == null) {
return;
}
for (EventWriter writer : AbstractEventSpyImpl.this.writers) {
if (writer == null) {
continue;
}
writer.write(event);
}
}
@Override
public void write(String key, String value, Level level, Object object) throws IOException {
if (AbstractEventSpyImpl.this.writers == null) {
return;
}
for (EventWriter writer : AbstractEventSpyImpl.this.writers) {
if (writer == null) {
continue;
}
writer.write(key, value, level, object);
}
}
@Override
public void close() throws IOException {
if (AbstractEventSpyImpl.this.writers == null) {
return;
}
for (EventWriter writer : AbstractEventSpyImpl.this.writers) {
if (writer == null) {
continue;
}
writer.close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy