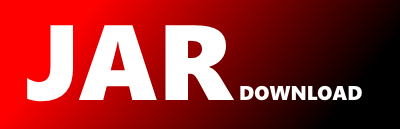
net.aequologica.neo.shakuntala.EventSpyImpl Maven / Gradle / Ivy
package net.aequologica.neo.shakuntala;
import static net.aequologica.neo.shakuntala.EventWriterDagImpl.DAG;
import static org.apache.maven.execution.ExecutionEvent.Type.SessionEnded;
import static org.apache.maven.execution.ExecutionEvent.Type.SessionStarted;
import java.net.InetAddress;
import java.nio.file.Paths;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Properties;
import java.util.Set;
import javax.inject.Named;
import javax.inject.Singleton;
import org.apache.maven.eventspy.EventSpy;
import org.apache.maven.execution.ExecutionEvent;
import org.apache.maven.execution.MavenExecutionRequest;
import org.apache.maven.execution.MavenExecutionResult;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.model.Developer;
import org.apache.maven.plugin.MojoExecution;
import org.apache.maven.project.MavenProject;
import org.apache.maven.settings.building.SettingsBuildingRequest;
import net.aequologica.neo.shakuntala.dagr.DagComputer;
@Named
@Singleton
public class EventSpyImpl extends AbstractEventSpyImpl implements EventSpy {
private Set throwables = new HashSet();
@Override
public void onEvent(Object object) throws Exception {
super.onEvent(object);
if (object == null) {
return;
}
if (object instanceof SettingsBuildingRequest) {
InetAddress address = InetAddress.getLocalHost();
if (address != null) {
dispatch("hostName", address.getHostName(), Event.Level.info, object);
dispatch("hostAddress", address.getHostAddress(), Event.Level.info, object);
}
// ----------------------------------------------------------------------------------------
// maven execution request/result
} else if (object instanceof MavenExecutionRequest) {
MavenExecutionRequest executionRequest = (MavenExecutionRequest) object;
String[] propertyKeys = new String[] {
"BUILD_URL",
"TRAVIS_BRANCH",
"TRAVIS_BUILD_ID",
"TRAVIS_COMMIT",
"TRAVIS_JOB_ID",
"TRAVIS_REPO_SLUG"
};
dispatchProperties(executionRequest, propertyKeys);
} else if (object instanceof MavenExecutionResult) {
MavenExecutionResult mavenExecutionResult = (MavenExecutionResult) object;
List exceptions = mavenExecutionResult.getExceptions();
if (exceptions != null) {
for (Throwable throwable : exceptions) {
if (!throwables.contains(throwable)) {
dispatch("error", throwable.getMessage(), Event.Level.danger, object);
throwables.add(throwable);
}
}
}
} else if (object instanceof ExecutionEvent) {
ExecutionEvent executionEvent = (ExecutionEvent)object;
MavenProject project = executionEvent.getProject();
MojoExecution mojoExecution = executionEvent.getMojoExecution();
if (mojoExecution != null && project != null) {
dispatch(project.getName(), mojoExecution.toString(), null, object);
}
Exception exception = executionEvent.getException();
if (exception != null && !throwables.contains(exception)) {
dispatch("error", exception.getMessage(), Event.Level.danger, object);
throwables.add(exception);
}
ExecutionEvent.Type type = executionEvent.getType();
if (type != null) {
if (type.equals(SessionStarted)) {
MavenSession session = executionEvent.getSession();
if (session != null) {
MavenExecutionRequest request = session.getRequest();
MavenProject topLevelProject = session.getTopLevelProject();
if (request != null) {
List goals = request.getGoals();
String goalsAsString = goals.toString();
dispatch("goals", goalsAsString, Event.Level.info, object);
String baseDirectory = request.getBaseDirectory();
dispatch("basedir", baseDirectory, Event.Level.info, object);
if (config.isActive(DAG)) {
DagComputer dagComputer = new DagComputer(
config.getDagName(),
Paths.get(baseDirectory),
session,
config.getDagDepth(),
config.getContextPath(),
config.getGitRemoteName()).compute();
dispatch("dag", "dag", Event.Level.info, dagComputer);
}
}
if (topLevelProject != null) {
// GAV
String groupId = topLevelProject.getGroupId();
String artifactId = topLevelProject.getArtifactId();
String version = topLevelProject.getVersion();
String packaging = topLevelProject.getPackaging();
dispatch("groupId", groupId, Event.Level.info, object);
dispatch("artifactId", artifactId, Event.Level.info, object);
dispatch("version", version, Event.Level.info, object);
dispatch("packaging", packaging, Event.Level.info, object);
// lead dev
List developers = topLevelProject.getDevelopers();
if (developers.size() > 0) {
Developer developer = developers.get(0);
String email = developer.getEmail();
dispatch("email", email, Event.Level.info, object);
}
}
}
} else if (type.equals(SessionEnded)) {
MavenSession session = executionEvent.getSession();
if (session != null) {
MavenExecutionResult result = session.getResult();
if (result != null) {
if (result.hasExceptions()) {
dispatch("result", Boolean.FALSE.toString(), Event.Level.danger, object);
} else {
dispatch("result", Boolean.TRUE.toString(), Event.Level.success, object);
}
}
MavenExecutionRequest request = session.getRequest();
if (request != null) {
Date startTime = request.getStartTime();
if (startTime != null) {
Date finish = new Date();
long duration = finish.getTime() - startTime.getTime();
dispatch("duration", Long.toString(duration, 10), Event.Level.info, object);
}
}
}
}
}
}
}
private void dispatchProperties(MavenExecutionRequest executionRequest, String[] propertyKeys) throws Exception {
Properties userProperties = executionRequest.getUserProperties();
Properties systemProperties = executionRequest.getSystemProperties();
for (String propertyKey : propertyKeys) {
if (userProperties != null) {
String property = userProperties.getProperty(propertyKey);
if (property != null && property.length() > 0) {
dispatch(propertyKey, property, Event.Level.info, executionRequest);
} else {
if (systemProperties != null) {
property = systemProperties.getProperty("env."+propertyKey);
if (property != null && property.length() > 0) {
dispatch(propertyKey, property, Event.Level.info, executionRequest);
}
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy