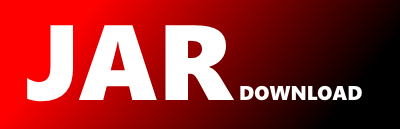
net.aequologica.neo.shakuntala.EventWriterLocalImpl Maven / Gradle / Ivy
package net.aequologica.neo.shakuntala;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.EnumMap;
import java.util.EnumSet;
import java.util.Iterator;
import javax.inject.Inject;
import javax.inject.Named;
import javax.inject.Singleton;
import org.eclipse.aether.RepositoryEvent.EventType;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.io.Files;
@Singleton
@Named(value = "local")
public class EventWriterLocalImpl extends AbstractEventWriter implements EventWriter {
private static Logger log = LoggerFactory.getLogger(EventWriterLocalImpl.class);
@Inject
@Named("repositoryEventFilter")
private RepositoryEventFilter repositoryEventFilter;
@Inject
@Named("repositoryEventCodec")
private EventCodec codec;
@Inject
@Named("uuid")
private UUIDProducer uuid;
@Inject
@Named("application.conf")
private EventSpyConfig config;
private final Id id = new Id();
EnumMap names = new EnumMap(EventType.class);
@Override
public void init() throws IOException {
super.init(); // must call super to set the shutdownHook
if (config == null) {
throw new RuntimeException();
}
if (!config.isActive("local")) {
return;
}
}
private class Id {
private String groupId = null;
private String artifactId = null;
private String version = null;
private String packaging = null;
private File getFile(String eventType) {
if (this.groupId == null ||
this.artifactId == null ||
this.version == null ||
this.packaging == null ||
eventType == null
) {
return null;
}
Path path = Paths.get(
this.groupId.replace(".", "/"),
this.artifactId,
this.version,
this.artifactId + "-" +
this.version + "." +
this.packaging + "." +
eventType +
".json");
return path.toFile();
}
private void accumulate(String key, String value) {
if (key.equals("groupId") && this.groupId == null) { this.groupId = value; } else
if (key.equals("artifactId") && this.artifactId == null) { this.artifactId = value; } else
if (key.equals("version") && this.version == null) { this.version = value; } else
if (key.equals("packaging") && this.packaging == null) { this.packaging = value; }
}
@Override
public String toString() {
return "Id [groupId=" + groupId + ", artifactId=" + artifactId + ", version=" + version + ", packaging=" + packaging + "]";
}
}
private class Name {
int written = 0;
BufferedWriter writer = null;
File file = null;
private Name(String eventType) throws IOException {
if (eventType == null || eventType.length()==0) {
throw new IllegalArgumentException("null or empty eventType");
}
this.file = new File(config.getLocalOutput(), eventType+"-"+uuid.getRandomUUID() + ".json");
this.writer = new BufferedWriter(new FileWriter( this.file ));
this.writer.write("[");
}
private void writeEvent(final Event event) throws IOException {
final String stringified;
if (codec != null) {
stringified = codec.toString(event);
} else {
stringified = event.toString();
}
if (stringified == null || stringified.length() == 0) {
return;
}
if (this.writer == null) {
return;
}
if (0 < written) {
writer.write(",");
writer.newLine();
}
writer.write(stringified);
written++;
}
}
@Override
public void write(final Event event) throws IOException {
if (event == null) {
return;
}
if (!config.isActive("local")) {
return;
}
try {
id.accumulate(event.getKey(), event.getValue());
if (this.repositoryEventFilter != null ) {
if (this.repositoryEventFilter.accept(event, EnumSet.of(EventType.ARTIFACT_RESOLVED))) {
Name name = names.get(EventType.ARTIFACT_RESOLVED);
if (name == null) {
name = new Name(EventType.ARTIFACT_RESOLVED.toString());
names.put(EventType.ARTIFACT_RESOLVED, name);
}
name.writeEvent(event);
}
if (this.repositoryEventFilter.accept(event, EnumSet.of(EventType.ARTIFACT_INSTALLED))) {
Name name = names.get(EventType.ARTIFACT_INSTALLED);
if (name == null) {
name = new Name(EventType.ARTIFACT_INSTALLED.toString());
names.put(EventType.ARTIFACT_INSTALLED, name);
}
name.writeEvent(event);
}
}
} catch (Exception e) {
log.warn("[shakuntala] " + event.toString(), e);
}
}
@Override
public void close() throws IOException {
super.close();
if (!config.isActive("local")) {
return;
}
synchronized (names) {
Iterator enumKeySet = names.keySet().iterator();
while (enumKeySet.hasNext()){
EventType eventType = enumKeySet.next();
Name name = names.get(eventType);
if (name == null) {
return;
}
if (name.writer != null) {
name.writer.newLine();
name.writer.write("]");
name.writer.flush();
name.writer.close();
name.writer = null;
}
try {
if (name.file == null) {
return;
}
File newFile = id.getFile(eventType.toString());
if (newFile == null) {
return;
}
if (newFile.exists()) {
newFile.delete();
}
Path oldPath = name.file.toPath();
Path newPath = oldPath.resolveSibling(newFile.toPath());
newPath.getParent().toFile().mkdirs();
Files.move(name.file, newPath.toFile());
name.file = null;
log.info("[shakuntala] saved BOM to {}", newPath.toFile().getCanonicalPath());
} catch (Exception e) {
e.printStackTrace();
}
}
names.clear();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy