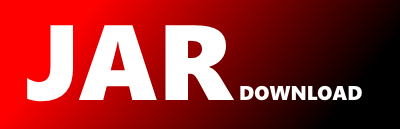
commonMain.agl.grammar.style.AglStyleSyntaxAnalyser.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of agl-processor-jvm8 Show documentation
Show all versions of agl-processor-jvm8 Show documentation
Dynamic, scan-on-demand, parsing; when a regular expression is just not enough
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy