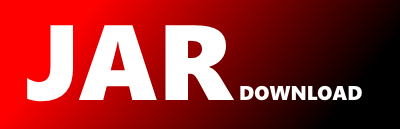
commonMain.api.grammar.Grammar.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of agl-processor-jvm8 Show documentation
Show all versions of agl-processor-jvm8 Show documentation
Dynamic, scan-on-demand, parsing; when a regular expression is just not enough
The newest version!
/**
* Copyright (C) 2018 Dr. David H. Akehurst (http://dr.david.h.akehurst.net)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.akehurst.language.api.grammar
import net.akehurst.language.agl.collections.OrderedSet
interface GrammarItem {
val grammar: Grammar
}
interface GrammarReference {
val localNamespace: Namespace
val nameOrQName: String
val resolved: Grammar?
fun resolveAs(resolved: Grammar)
}
interface GrammarOption {
val name: String
val value: String
}
/**
*
* The definition of a Grammar. A grammar defines a list of rules and may be defined to extend a number of other Grammars.
*
*/
interface Grammar {
/**
*
* the namespace of this grammar;
*/
val namespace: Namespace
/**
*
* the name of this grammar
*/
val name: String
/**
* namespace.name
*/
val qualifiedName: String
/**
* the List of grammar references directly extended by this one (non-transitive)
*/
val extends: List
val extendsResolved: List
val options: List
val defaultRule: GrammarRule
val grammarRule: List
val preferenceRule: List
val allGrammarReferencesInRules: List
/**
* the OrderedSet of grammars references extended by this one or those it extends (transitive)
*/
val allExtends: OrderedSet
/**
* the OrderedSet of grammars extended by this one or those it extends (transitive)
*/
val allExtendsResolved: OrderedSet
/**
* List of all grammar rules that belong to grammars this one extends (non-transitive)
*/
val directInheritedGrammarRule: List
/**
* List of all grammar rules (transitive over extended grammars), including those overridden
* the order of the rules is the order they are defined in with the top of the grammar extension
* hierarchy coming first (in extension order where more than one grammar is extended)
*/
val allGrammarRule: List
/**
* List of all grammar rules that belong to grammars this one extends (non-transitive)
* with best-effort to resolve repetition and override
*/
val directInheritedResolvedGrammarRule: OrderedSet
/**
* List of all grammar rules that belong to grammars this one extends (transitive)
* with best-effort to resolve repetition and override
*/
val allInheritedResolvedGrammarRule: OrderedSet
val resolvedGrammarRule: OrderedSet
/**
* the List of rules defined by this grammar and those that this grammar extends (transitive),
* with best-effort to handle repetition and overrides
* the order of the rules is the order they are defined in with the top of the grammar extension
* hierarchy coming first (in extension order where more than one grammar is extended)
*/
val allResolvedGrammarRule: OrderedSet
val allResolvedPreferenceRuleRule: OrderedSet
/**
* the Set of all non-terminal rules in this grammar and those that this grammar extends
*/
val allResolvedNonTerminalRule: Set
/**
* the Set of all terminals in this grammar and those that this grammar extends
*/
val allResolvedTerminal: Set
/**
* the Set of all terminals that are part of skip rules in this grammar and those that this grammar extends
*/
val allResolvedSkipTerminal: Set
val allResolvedEmbeddedRules: Set
val allResolvedEmbeddedGrammars: Set
/**
* find rule with given name in all rules that this grammar extends - but not in this grammar
*/
fun findAllSuperGrammarRule(ruleName: String): List
/**
* find rule with given name in all rules from this grammar and ones that this grammar extends
*/
fun findAllGrammarRuleList(ruleName: String): List
fun findAllResolvedGrammarRule(ruleName: String): GrammarRule?
fun findAllResolvedTerminalRule(terminalPattern: String): Terminal
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy