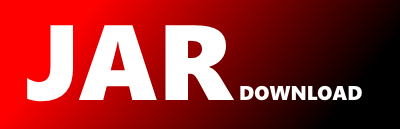
net.alantea.swing.action.ActionMultipleSelector2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.action;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.swing.BoxLayout;
import javax.swing.DefaultListModel;
import javax.swing.JComponent;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JPanel;
import javax.swing.ListSelectionModel;
import javax.swing.event.ListSelectionEvent;
import net.alantea.liteprops.Property;
import net.alantea.utils.MultiMessages;
/**
* The Class LabeledTextField.
*/
public class ActionMultipleSelector2 extends JPanel
{
/** The Constant serialVersionUID. */
private static final long serialVersionUID = 1L;
private JLabel label;
private JList list;
/** The target. */
private Object target;
/** The action key. */
private String actionKey;
private Property data = new Property<>();
private boolean allowNull = false;
/**
* Instantiates a new labeled text field.
*
* @param parent the parent
* @param target the target
* @param actionKey the action key
*/
public ActionMultipleSelector2(JPanel parent, Object target, String actionKey)
{
super();
this.setLayout(new BoxLayout(this, BoxLayout.PAGE_AXIS));
this.target = target;
this.actionKey = actionKey;
list = new JList(new DefaultListModel());
list.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION);
ListSelectionModel listSelectionModel = list.getSelectionModel();
listSelectionModel.addListSelectionListener(this::itemStateChanged);
add(list);
label = new JLabel(MultiMessages.get(actionKey));
label.setAlignmentX(JComponent.CENTER_ALIGNMENT);
add(label);
parent.add(this);
}
private void itemStateChanged(ListSelectionEvent event)
{
Map map = new HashMap<>();
for (int i = 0; i < list.getModel().getSize(); i++)
{
boolean b = list.isSelectedIndex(i);
T value = list.getModel().getElementAt(i);
map.put(value, b);
}
ManagedSelection.trigger(actionKey, target, map);
}
public void setItems(List items)
{
if (items != null)
{
DefaultListModel model = (DefaultListModel) list.getModel();
list.removeAll();
if (allowNull)
{
model.addElement(null);
}
for(T item : items)
{
model.addElement(item);
}
}
}
public void allowNull(boolean flag)
{
allowNull = flag;
}
public Property getProperty()
{
return data;
}
public List getSelectedItems()
{
return list.getSelectedValuesList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy