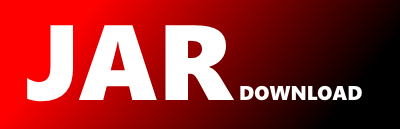
net.alantea.swing.graph.GraphConnection Maven / Gradle / Ivy
package net.alantea.swing.graph;
import java.awt.Color;
import java.awt.Component;
import net.alantea.utils.ColorNames;
/**
* The Class GraphConnection.
*/
public class GraphConnection
{
/** The start. */
private Component start;
/** The end. */
private Component end;
/** The start arrow. */
private boolean startArrow;
/** The end arrow. */
private boolean endArrow;
/** The fill color. */
private Color fillColor = Color.BLACK;
/** The hover color. */
private Color hoverColor = Color.WHITE;
/**
* Instantiates a new graph connection.
*
* @param start the start
* @param end the end
* @param startArrow the start arrow
* @param endArrow the end arrow
*/
public GraphConnection(Component start, Component end, boolean startArrow, boolean endArrow)
{
this.start = start;
this.end = end;
this.startArrow = startArrow;
this.endArrow = endArrow;
}
/**
* Gets the start.
*
* @return the start
*/
public Component getStart()
{
return start;
}
/**
* Gets the end.
*
* @return the end
*/
public Component getEnd()
{
return end;
}
/**
* Checks if is start arrow.
*
* @return true, if is start arrow
*/
public boolean isStartArrow()
{
return startArrow;
}
/**
* Checks if is end arrow.
*
* @return true, if is end arrow
*/
public boolean isEndArrow()
{
return endArrow;
}
/**
* Gets the fill color.
*
* @return the fill color
*/
public Color getFillColor()
{
return fillColor;
}
/**
* Sets the fill color.
*
* @param fillColor the new fill color
*/
public void setFillColor(String fillColor)
{
setFillColor(ColorNames.getColor(fillColor));
}
/**
* Sets the fill color.
*
* @param fillColor the new fill color
*/
public void setFillColor(Color fillColor)
{
this.fillColor = fillColor;
}
/**
* Gets the hover color.
*
* @return the hover color
*/
public Color getHoverColor()
{
return hoverColor;
}
/**
* Sets the hover color.
*
* @param hoverColor the new hover color
*/
public void setHoverColor(Color hoverColor)
{
this.hoverColor = hoverColor;
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(Object object)
{
if (object instanceof GraphConnection)
{
return ((start.equals(((GraphConnection)object).start)) && (end.equals(((GraphConnection)object).end)));
}
return false;
}
/* (non-Javadoc)
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode()
{
return start.hashCode() + end.hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy