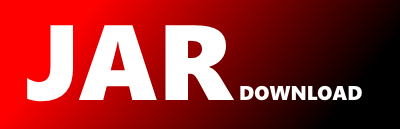
net.alantea.swing.judith.JudithRibbon Maven / Gradle / Ivy
package net.alantea.swing.judith;
import java.awt.Color;
import java.awt.Font;
import java.awt.event.ActionEvent;
import javax.swing.JEditorPane;
import javax.swing.text.SimpleAttributeSet;
import javax.swing.text.StyleConstants;
import javax.swing.text.StyledEditorKit;
import javax.swing.text.StyledEditorKit.StyledTextAction;
import net.alantea.swing.action.ActionButton;
import net.alantea.swing.action.ActionManager;
import net.alantea.swing.ribbon.Ribbon;
import net.alantea.swing.ribbon.RibbonGroup;
import net.alantea.swing.ribbon.RibbonTab;
@SuppressWarnings("serial")
public class JudithRibbon extends Ribbon
{
public JudithRibbon(EditorPanel editorPanel)
{
ActionManager.instrumentAction("JudithRibbon.normal", editorPanel, new NormalAction());
ActionManager.instrumentAction("JudithRibbon.bold", editorPanel, new StyledEditorKit.BoldAction());
ActionManager.instrumentAction("JudithRibbon.italic", editorPanel, new StyledEditorKit.ItalicAction());
ActionManager.instrumentAction("JudithRibbon.underline", editorPanel, new StyledEditorKit.UnderlineAction());
ActionManager.instrumentAction("JudithRibbon.size.10", editorPanel, new StyledEditorKit.FontSizeAction("10", 10));
ActionManager.instrumentAction("JudithRibbon.size.12", editorPanel, new StyledEditorKit.FontSizeAction("12", 12));
ActionManager.instrumentAction("JudithRibbon.size.14", editorPanel, new StyledEditorKit.FontSizeAction("14", 14));
ActionManager.instrumentAction("JudithRibbon.size.18", editorPanel, new StyledEditorKit.FontSizeAction("18", 18));
ActionManager.instrumentAction("JudithRibbon.foreground.red", editorPanel, new StyledEditorKit.ForegroundAction("Red", Color.red));
ActionManager.instrumentAction("JudithRibbon.foreground.orange", editorPanel, new StyledEditorKit.ForegroundAction("Orange", Color.orange));
ActionManager.instrumentAction("JudithRibbon.foreground.yellow", editorPanel, new StyledEditorKit.ForegroundAction("Yellow", Color.yellow));
ActionManager.instrumentAction("JudithRibbon.foreground.green", editorPanel, new StyledEditorKit.ForegroundAction("Green", Color.green));
ActionManager.instrumentAction("JudithRibbon.foreground.blue", editorPanel, new StyledEditorKit.ForegroundAction("Blue", Color.blue));
ActionManager.instrumentAction("JudithRibbon.foreground.magenta", editorPanel, new StyledEditorKit.ForegroundAction("Magenta", Color.magenta));
ActionManager.instrumentAction("JudithRibbon.foreground.black", editorPanel, new StyledEditorKit.ForegroundAction("Black", Color.black));
ActionManager.instrumentAction("JudithRibbon.foreground.cyan", editorPanel, new StyledEditorKit.ForegroundAction("Cyan", Color.cyan));
ActionManager.instrumentAction("JudithRibbon.foreground.darkgray", editorPanel, new StyledEditorKit.ForegroundAction("DarkGray", Color.darkGray));
ActionManager.instrumentAction("JudithRibbon.foreground.gray", editorPanel, new StyledEditorKit.ForegroundAction("Gray", Color.gray));
ActionManager.instrumentAction("JudithRibbon.foreground.lightgray", editorPanel, new StyledEditorKit.ForegroundAction("LightGray", Color.lightGray));
ActionManager.instrumentAction("JudithRibbon.foreground.pink", editorPanel, new StyledEditorKit.ForegroundAction("Pink", Color.pink));
ActionManager.instrumentAction("JudithRibbon.foreground.white", editorPanel, new StyledEditorKit.ForegroundAction("White", Color.white));
ActionManager.instrumentAction("JudithRibbon.font.serif", editorPanel, new StyledEditorKit.FontFamilyAction("Serif", Font.SERIF));
ActionManager.instrumentAction("JudithRibbon.font.sans", editorPanel, new StyledEditorKit.FontFamilyAction("Serif", Font.SANS_SERIF));
ActionManager.instrumentAction("JudithRibbon.font.mono", editorPanel, new StyledEditorKit.FontFamilyAction("Serif", Font.MONOSPACED));
RibbonTab style = new RibbonTab(this, null, "JudithRibbon.style");
RibbonGroup group = new RibbonGroup(style, "JudithRibbon.police");
new ActionButton(group, editorPanel, "JudithRibbon.normal");
new ActionButton(group, editorPanel, "JudithRibbon.bold");
new ActionButton(group, editorPanel, "JudithRibbon.italic");
new ActionButton(group, editorPanel, "JudithRibbon.underline");
RibbonGroup group1 = new RibbonGroup(style, "JudithRibbon.size");
new ActionButton(group1, editorPanel, "JudithRibbon.size.10");
new ActionButton(group1, editorPanel, "JudithRibbon.size.12");
new ActionButton(group1, editorPanel, "JudithRibbon.size.14");
new ActionButton(group1, editorPanel, "JudithRibbon.size.18");
RibbonTab foreground = new RibbonTab(this, null, "JudithRibbon.foreground");
new ActionButton(foreground, editorPanel, "JudithRibbon.foreground.red");
new ActionButton(foreground, editorPanel, "JudithRibbon.foreground.orange");
new ActionButton(foreground, editorPanel, "JudithRibbon.foreground.yellow");
new ActionButton(foreground, editorPanel, "JudithRibbon.foreground.green");
new ActionButton(foreground, editorPanel, "JudithRibbon.foreground.blue");
new ActionButton(foreground, editorPanel, "JudithRibbon.foreground.magenta");
new ActionButton(foreground, editorPanel, "JudithRibbon.foreground.cyan");
new ActionButton(foreground, editorPanel, "JudithRibbon.foreground.pink");
RibbonTab grays = new RibbonTab(this, null, "JudithRibbon.grays");
new ActionButton(grays, editorPanel, "JudithRibbon.foreground.black");
new ActionButton(grays, editorPanel, "JudithRibbon.foreground.darkgray");
new ActionButton(grays, editorPanel, "JudithRibbon.foreground.gray");
new ActionButton(grays, editorPanel, "JudithRibbon.foreground.lightgray");
new ActionButton(grays, editorPanel, "JudithRibbon.foreground.white");
RibbonTab misc = new RibbonTab(this, null, "JudithRibbon.misc");
RibbonGroup actions = new RibbonGroup(misc, "JudithRibbon.actions");
new ActionButton(actions, editorPanel, "JudithRibbon.actions.undo");
new ActionButton(actions, editorPanel, "JudithRibbon.actions.redo");
RibbonGroup fonts = new RibbonGroup(misc, "JudithRibbon.fonts");
new ActionButton(fonts, editorPanel, "JudithRibbon.font.serif");
new ActionButton(fonts, editorPanel, "JudithRibbon.font.sans");
new ActionButton(fonts, editorPanel, "JudithRibbon.font.mono");
}
public static class NormalAction extends StyledTextAction
{
/**
* Constructs a new BoldAction.
*/
public NormalAction()
{
super("font-normal");
}
/**
* Toggles the attributes.
*
* @param e the action event
*/
public void actionPerformed(ActionEvent e)
{
JEditorPane editor = getEditor(e);
if (editor != null)
{
SimpleAttributeSet sas = new SimpleAttributeSet();
StyleConstants.setBold(sas, false);
StyleConstants.setItalic(sas, false);
StyleConstants.setUnderline(sas, false);
setCharacterAttributes(editor, sas, false);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy