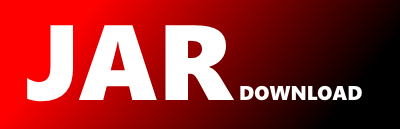
net.alantea.swing.misc.FileChooser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.misc;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JPanel;
import net.alantea.swing.layout.percent.PercentConstraints;
import net.alantea.swing.layout.percent.PercentLayout;
import net.alantea.swing.text.TextField;
import net.alantea.utils.MultiMessages;
@SuppressWarnings("serial")
public class FileChooser extends JPanel
{
private TextField textfield;
public FileChooser(String key, boolean editable, boolean directory)
{
setLayout(new PercentLayout());
textfield = new TextField();
textfield.setEditable(editable);
PercentConstraints fconst = new PercentConstraints(0.0, 0.0, 0.6, 1.0);
add(textfield, fconst);
JButton searchButton = new JButton(MultiMessages.get(key));
add(searchButton, new PercentConstraints(0.8, 0.0, 0.2, 1.0));
searchButton.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
JFileChooser fileChooser = new JFileChooser();
String path = textfield.getText();
String dir = path;
if (directory)
{
fileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
}
else if ((path != null) && (!path.isEmpty()))
{
dir = new File(dir).getParent();
}
if ((path != null) && (!path.isEmpty()))
{
fileChooser.setCurrentDirectory(new File(dir));
}
int result = fileChooser.showDialog(null, MultiMessages.get(key + ".label"));
if (result == JFileChooser.APPROVE_OPTION )
{
textfield.setText(fileChooser.getSelectedFile().getAbsolutePath());
}
}
});
}
public void setPath(String path)
{
textfield.setText(path);
}
public String getPath()
{
return textfield.getText();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy