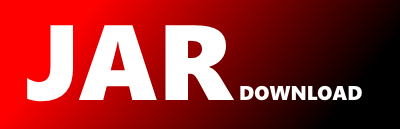
net.alantea.swing.pageelements.GUIElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.pageelements;
import java.awt.Component;
import java.util.HashMap;
import java.util.Map;
import javax.swing.JComponent;
import javax.swing.JLabel;
import javax.swing.JPanel;
import net.alantea.swing.layout.percent.PercentConstraints;
import net.alantea.swing.layout.percent.PercentLayout;
import net.alantea.swing.layout.percent.Where;
import net.alantea.utils.MultiMessages;
/**
* The Class GUIElement.
*/
public abstract class GUIElement
{
/** The decorations type. */
private String type;
/** The key. */
private String key;
/** The page. */
private String page;
/** The order. */
private int order;
/** The height. */
private int height;
/** The target. */
private Object target;
/** The editable. */
private boolean editable;
private JPanel panel;
/** The attrs. */
Map attrs = new HashMap<>();
/**
* Instantiates a new GUI element.
*
* @param type the type
* @param target the target
* @param key the key
* @param editable the editable
* @param page the page
* @param height the height
* @param order the order
* @param attrs the attrs
*/
public GUIElement(String type, Object target, String key,
boolean editable, String page, int height, int order, Map attrs)
{
this.type = type;
this.key = key;
this.page = page;
this.order = order <= 0 ? 1000000000 : order;
this.height = height;
this.target = target;
this.editable = editable;
this.attrs = attrs;
this.panel = null;
}
/**
* Gets the type.
*
* @return the type
*/
public String getType()
{
return type;
}
/**
* Gets the order.
*
* @return the order
*/
public int getOrder()
{
return order;
}
/**
* Gets the height.
*
* @return the height
*/
public int getHeight()
{
return height;
}
/**
* Gets the key.
*
* @return the key
*/
public String getKey()
{
return key;
}
/**
* Gets the page.
*
* @return the page
*/
public String getPage()
{
return page;
}
/**
* Gets the target.
*
* @return the target
*/
public Object getTarget()
{
return target;
}
/**
* Checks if is editable.
*
* @return true, if is editable
*/
public boolean isEditable()
{
return editable;
}
/**
* Gets the attribute.
*
* @param key the key
* @return the attribute
*/
public String getAttribute(String key)
{
return attrs.get(key);
}
/**
* Gets the serialversionuid.
*
* @return the serialversionuid
*/
public static long getSerialversionuid()
{
return serialVersionUID;
}
/** The Constant serialVersionUID. */
private static final long serialVersionUID = 1L;
/**
* Gets the GUI component.
*
* @return the GUI component
*/
public Component getGUIComponent()
{
if (panel == null)
{
panel = new JPanel(){
private static final long serialVersionUID = 1L;
public void repaint()
{
getSpecificGUIComponent().repaint();
super.repaint();
}
};
panel.setLayout(new PercentLayout());
JLabel label = new JLabel();
label.setText(MultiMessages.get(key));
PercentConstraints labelConstraints = new PercentConstraints(0, 0, 0.25, 1.0);
panel.add(label, labelConstraints);
JComponent fieldPanel = getSpecificGUIComponent();
PercentConstraints fieldConstraints = new PercentConstraints(0, 0, 0, 1.0);
fieldConstraints.setXReferent(label, Where.AFTER);
fieldConstraints.setWReferent(label, Where.AFTER);
panel.add(fieldPanel, fieldConstraints);
}
return panel;
}
protected abstract JComponent getSpecificGUIComponent();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy