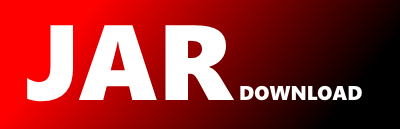
net.alantea.swing.pageelements.elements.PathElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.pageelements.elements;
import java.awt.Desktop;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.IOException;
import java.lang.reflect.Field;
import java.util.Map;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JFileChooser;
import javax.swing.JPanel;
import javax.swing.text.JTextComponent;
import net.alantea.horizon.message.Messenger;
import net.alantea.liteprops.StringProperty;
import net.alantea.swing.layout.percent.PercentConstraints;
import net.alantea.swing.layout.percent.PercentLayout;
import net.alantea.swing.pageelements.GUIFieldAnnotation;
import net.alantea.swing.pageelements.GUIFieldElement;
import net.alantea.swing.text.InstrumentedTextField;
import net.alantea.utils.MultiMessages;
import net.alantea.utils.exception.LntException;
@GUIFieldAnnotation(value="FILE", height=32)
public class PathElement extends GUIFieldElement
{
private JComponent panel;
private InstrumentedTextField textfield;
private boolean avoidCollisions = false;
PathElement(String reference, String type, Field field, Object target,
String key, boolean editable, String page, int height, int order, Map attrs)
{
super(type, field, target, key, editable, page, height, order, attrs);
panel = new JPanel();
panel.setLayout(new PercentLayout());
StringProperty prop = null;
textfield = new InstrumentedTextField();
PercentConstraints fconst = new PercentConstraints(0.0, 0.0, 0.6, 1.0);
panel.add(textfield, fconst);
((JTextComponent) textfield).setEditable(editable);
prop = ((InstrumentedTextField) textfield).getStringProperty();
JButton openButton = new JButton(MultiMessages.get(key + ".edit"));
panel.add(openButton, new PercentConstraints(0.6, 0.0, 0.2, 1.0));
openButton.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
String path = textfield.getText();
if ((path != null) && (!path.isEmpty()))
{
File file = new File(path);
try
{
file.createNewFile();
Desktop.getDesktop().open(file);
}
catch (IOException e1)
{
new LntException("Error launching editor on file", e1);
}
}
}
});
JButton searchButton = new JButton(MultiMessages.get(key + ".search"));
panel.add(searchButton, new PercentConstraints(0.8, 0.0, 0.2, 1.0));
searchButton.addActionListener(new ActionListener()
{
@Override
public void actionPerformed(ActionEvent e)
{
JFileChooser fileChooser = new JFileChooser();
String path = textfield.getText();
String dir = path;
if ((attrs != null)
&& (attrs.get("directory") != null)
&& (attrs.get("directory").equalsIgnoreCase("true")))
{
fileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
}
else if ((path != null) && (!path.isEmpty()))
{
dir = new File(dir).getParent();
}
if ((path != null) && (!path.isEmpty()))
{
fileChooser.setCurrentDirectory(new File(dir));
}
int result = fileChooser.showDialog(null, MultiMessages.get(key + ".search.label"));
if (result == JFileChooser.APPROVE_OPTION )
{
textfield.setText(fileChooser.getSelectedFile().getAbsolutePath());
}
}
});
try
{
if (field.getType().getName().equals(StringProperty.class.getName()))
{
StringProperty prop1 = (StringProperty) field.get(target);
String val = prop1.get();
((InstrumentedTextField)textfield).setText(val);
if (prop != null)
{
prop.addListener((o, v) -> {
if (!avoidCollisions)
{
if (!v.equals(((InstrumentedTextField)textfield).getText() ))
{
((InstrumentedTextField)textfield).setText(v);
}
}
avoidCollisions = false;
});
}
}
else if (field.getType().getName().equals(String.class.getName()))
{
((InstrumentedTextField)textfield).setText((String) field.get(target));
}
}
catch (IllegalArgumentException | IllegalAccessException e)
{
e.printStackTrace();
}
if (prop != null)
{
prop.addListener((o, v) -> {
try
{
String old = "";
if (field.getType().getName().equals(StringProperty.class.getName()))
{
StringProperty prop1 = (StringProperty) field.get(target);
old = prop1.get();
if ((old == null) || (!old.equals(v)))
{
avoidCollisions = true;
prop1.set(v);
}
}
else if (field.getType().getName().equals(String.class.getName()))
{
old = (String) field.get(target);
field.set(target, v);
}
if ((old == null) || (!old.equals(v)))
{
Messenger.sendMessage(target, field.getName(), v);
}
}
catch (IllegalArgumentException | IllegalAccessException e)
{
e.printStackTrace();
}
});
}
}
@Override
protected JComponent getSpecificGUIComponent()
{
return panel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy