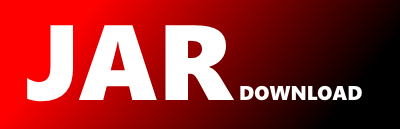
net.alantea.swing.pageelements.elements.URLElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.pageelements.elements;
import java.awt.BorderLayout;
import java.awt.Desktop;
import java.awt.event.ActionEvent;
import java.io.IOException;
import java.lang.reflect.Field;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Map;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JPanel;
import net.alantea.horizon.message.Messenger;
import net.alantea.liteprops.StringProperty;
import net.alantea.swing.pageelements.GUIFieldAnnotation;
import net.alantea.swing.pageelements.GUIFieldElement;
import net.alantea.swing.text.InstrumentedTextField;
import net.alantea.utils.MultiMessages;
import net.alantea.utils.exception.LntException;
@GUIFieldAnnotation(value="URL", height=32)
public class URLElement extends GUIFieldElement
{
private JPanel panel;
private InstrumentedTextField textfield;
private boolean avoidCollisions = false;
URLElement(String reference, String type, Field field, Object target, String key,
boolean editable, String page, int height, int order, Map attrs)
{
super(type, field, target, key, editable, page, height, order, attrs);
panel = new JPanel(new BorderLayout());
textfield = new InstrumentedTextField();
JButton seeButton = new JButton(MultiMessages.get("URLElement.button.see.label"));
seeButton.setText("...");
seeButton.addActionListener(this::seeURL);
panel.add(seeButton, BorderLayout.WEST);
panel.add(textfield, BorderLayout.CENTER);
textfield.setEditable(editable);
textfield.getStringProperty().addListener((o, v) -> {
try
{
String old = "";
if (field.getType().getName().equals(StringProperty.class.getName()))
{
avoidCollisions = true;
StringProperty prop = (StringProperty) field.get(target);
old = prop.get();
prop.set(v);
}
else if (field.getType().getName().equals(String.class.getName()))
{
old = (String) field.get(target);
field.set(target, v);
}
if ((old == null) || (!old.equals(v)))
{
Messenger.sendMessage(target, field.getName(), v);
}
}
catch (IllegalArgumentException | IllegalAccessException e)
{
new LntException("Error getting URL from field", e);
}
});
try
{
if (field.getType().getName().equals(StringProperty.class.getName()))
{
StringProperty prop = (StringProperty) field.get(target);
String val = prop.get();
textfield.setText(val);
prop.addListener((o, v) -> {
if (!avoidCollisions)
{
textfield.setText(v);
}
avoidCollisions = false;
});
}
else if (field.getType().getName().equals(String.class.getName()))
{
textfield.setText((String) field.get(target));
}
}
catch (IllegalArgumentException | IllegalAccessException e)
{
new LntException("Error reading URL field", e);
}
}
private void seeURL(ActionEvent e)
{
if (Desktop.isDesktopSupported() && Desktop.getDesktop().isSupported(Desktop.Action.BROWSE)) {
try
{
Desktop.getDesktop().browse(new URI(textfield.getText()));
}
catch (IOException | URISyntaxException e1)
{
new LntException("Error opening URL on desktop", e1);
}
}
}
@Override
protected JComponent getSpecificGUIComponent()
{
return panel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy