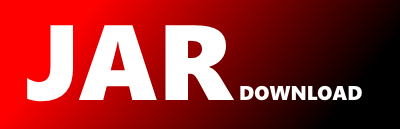
net.alantea.swing.panels.FoldablePanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.panels;
import java.awt.Component;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JPanel;
import net.alantea.swing.misc.ResourceManager;
/**
* The Class FoldablePanel.
*/
@SuppressWarnings("serial")
public class FoldablePanel extends JPanel
{
/** The pane. */
private JComponent pane;
/** The up. */
private JButton up;
/** The down. */
private JButton down;
/**
* Instantiates a new foldable panel.
*
* @param content the content
*/
public FoldablePanel(JComponent content)
{
super();
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
pane = content;
super.add(pane);
up = new JButton();
up.setIcon(ResourceManager.getIcon("ribbon-up.png"));
up.addActionListener(e -> showContent(false));
super.add(up);
down = new JButton();
down.setIcon(ResourceManager.getIcon("ribbon-down.png"));
down.addActionListener(e -> showContent(true));
super.add(down);
down.setVisible(false);
}
/**
* Gets the content component.
*
* @return the content component
*/
public JComponent getContent()
{
return pane;
}
/**
* Show content.
*
* @param flag the flag
*/
public void showContent(boolean flag)
{
pane.setVisible(flag);
up.setVisible(flag);
down.setVisible(!flag);
}
/**
* Adds the.
*
* @param comp the comp
* @return the component
*/
public Component add(Component comp)
{
return pane.add(comp);
}
/**
* Adds the.
*
* @param name the name
* @param comp the comp
* @return the component
*/
public Component add(String name, Component comp)
{
return pane.add(name, comp);
}
/**
* Adds the.
*
* @param comp the comp
* @param index the index
* @return the component
*/
public Component add(Component comp, int index)
{
return pane.add(comp, index);
}
/**
* Adds the.
*
* @param comp the comp
* @param constraints the constraints
*/
public void add(Component comp, Object constraints)
{
pane.add(comp, constraints);
}
/**
* Adds the.
*
* @param comp the comp
* @param constraints the constraints
* @param index the index
*/
public void add(Component comp, Object constraints, int index)
{
pane.add(comp, constraints, index);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy