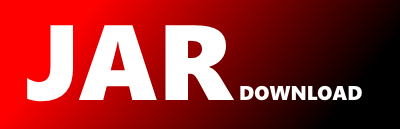
net.alantea.swing.progress.CircleProgressBar Maven / Gradle / Ivy
package net.alantea.swing.progress;
import java.awt.Color;
import javax.swing.BoundedRangeModel;
import javax.swing.JProgressBar;
/**
* The Class CircleProgressBar.
*/
@SuppressWarnings("serial")
public class CircleProgressBar extends JProgressBar
{
/** The ui. */
private ProgressCircleUI ui;
/**
* Instantiates a new circle progress bar.
*/
public CircleProgressBar()
{
super();
initUI();
}
/**
* Instantiates a new circle progress bar.
*
* @param orient the orient
*/
public CircleProgressBar(int orient)
{
super(orient);
initUI();
}
/**
* Instantiates a new circle progress bar.
*
* @param newModel the new model
*/
public CircleProgressBar(BoundedRangeModel newModel)
{
super(newModel);
initUI();
}
/**
* Instantiates a new circle progress bar.
*
* @param min the min
* @param max the max
*/
public CircleProgressBar(int min, int max)
{
super(min, max);
initUI();
}
/**
* Instantiates a new circle progress bar.
*
* @param orient the orient
* @param min the min
* @param max the max
*/
public CircleProgressBar(int orient, int min, int max)
{
super(orient, min, max);
initUI();
}
/**
* Get bar thickness.
* @return bar thickness
*/
public double getBarThickness()
{
return ui.getBarThickness();
}
/**
* Set bar thickness.
* @param barThickness bar thickness between 0.0 and 1.0
*/
public void setBarThickness(double barThickness)
{
ui.setBarThickness(barThickness);
}
/**
* Get back color.
* @return the backColor
*/
public Color getBackColor()
{
return ui.getBackColor();
}
/**
* Set back color.
* @param backColor the backColor to set
*/
public void setBackColor(Color backColor)
{
ui.setBackColor(backColor);
}
/**
* Inits the UI.
*/
private void initUI()
{
setStringPainted(true);
ui = new ProgressCircleUI();
setUI(ui);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy