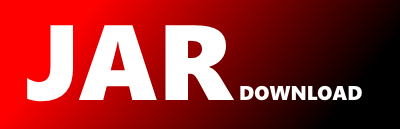
net.alantea.swing.text.TextField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.text;
import javax.swing.JTextField;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import net.alantea.liteprops.StringProperty;
import net.alantea.viewml.annotations.VAttribute;
import net.alantea.viewml.annotations.VElement;
/**
* The Class TextField.
*/
@VElement("textField")
public class TextField extends JTextField
{
/** The Constant serialVersionUID. */
private static final long serialVersionUID = 1L;
/**
* The Validator.
*/
public enum Validator
{
/** The none. */
NONE,
/** The NonBlank. */
NONBLANK,
/** The numeric. */
NUMERIC,
/** The hexadecimal. */
HEXADECIMAL,
/** The float. */
FLOAT,
/** The alphanumeric. */
ALPHANUMERIC,
/** The MAJNUMPLUS. */
MAJNUMPLUS,
}
/** The current text. */
StringProperty currentText = new StringProperty("");
/** The current caret. */
int currentCaret = 0;
/**
* The Interface SpecificValidator.
*/
@FunctionalInterface
public static interface SpecificValidator {
/**
* Do test.
*
* @param stringValue the string value
* @return true, if successful
*/
boolean doTest(String stringValue);
}
/** The validator. */
private SpecificValidator validator = this::validateNone;
/**
* Instantiates a new text field.
*/
public TextField()
{
this(Validator.NONE);
}
/**
* Instantiates a new text field.
*
* @param validatorIndex the validator index
*/
public TextField(Validator validatorIndex)
{
super();
setValidator(validatorIndex);
}
public StringProperty getTextProperty()
{
return currentText;
}
@VAttribute("text")
public void setText(String text)
{
super.setText(text);
currentText.set(text);
}
@VAttribute("validator")
public void setValidator(Validator validatorIndex)
{
setText("");
currentText.set("");
currentCaret = -1;
getDocument().addDocumentListener(new DocumentListener() {
@Override
public void changedUpdate(DocumentEvent e) {
updateLabel(e);
}
@Override
public void insertUpdate(DocumentEvent e) {
updateLabel(e);
}
@Override
public void removeUpdate(DocumentEvent e) {
updateLabel(e);
}
private void updateLabel(DocumentEvent e) {
java.awt.EventQueue.invokeLater(new Runnable()
{
public void run()
{
String text = getText();
int n = getCaretPosition();
if (!text.isEmpty())
{
if (validator.doTest(text))
{
currentText.set(text);
currentCaret = n;
}
else
{
TextField.super.setText(currentText.get());
if (currentCaret >= 0)
{
setCaretPosition(currentCaret);
}
}
} else {
setText(null);
}
}
});
}
});
switch(validatorIndex)
{
case NUMERIC :
validator = this::validateInteger;
break;
case HEXADECIMAL :
validator = this::validateHexadecimal;
break;
case FLOAT :
validator = this::validateFloat;
break;
case NONBLANK :
validator = this::validateNonBlank;
break;
case ALPHANUMERIC :
validator = this::validateAlphanumeric;
break;
case MAJNUMPLUS :
validator = this::validateMajNumPlus;
break;
case NONE :
default :
validator = this::validateNone;
break;
}
}
/**
* Validate none.
*
* @param text the text
* @return true, if successful
*/
boolean validateNone(String text)
{
return true;
}
/**
* Validate non blank.
*
* @param text the text
* @return true, if successful
*/
boolean validateNonBlank(String text)
{
return !(text.contains(" ") || text.contains("\t"));
}
/**
* Validate integer.
*
* @param text the text
* @return true, if successful
*/
boolean validateInteger(String text)
{
return text.matches("^[0-9]*$");
}
/**
* Validate hexadecimal.
*
* @param text the text
* @return true, if successful
*/
boolean validateHexadecimal(String text)
{
return text.matches("^[0-9A-F]*$");
}
/**
* Validate float.
*
* @param text the text
* @return true, if successful
*/
boolean validateFloat(String text)
{
return text.matches("^[0-9]*\\.*[0-9]*$");
}
/**
* Validate alphanumeric.
*
* @param text the text
* @return true, if successful
*/
boolean validateAlphanumeric(String text)
{
return text.matches("^\\S$");
}
/**
* Validate maj num plus.
*
* @param text the text
* @return true, if successful
*/
boolean validateMajNumPlus(String text)
{
return text.matches("^[0-9A-Z\\\\-]*$");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy