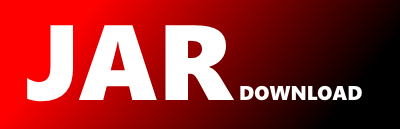
net.alantea.swing.ui.ImageTool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.ui;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Rectangle;
import java.awt.RenderingHints;
import javax.swing.ImageIcon;
public class ImageTool
{
public static void drawImageInto(Graphics g, ImageIcon icon, Rectangle view)
{
if (icon != null)
{
int posx = view.x;
int posy = view.y;
double ratioImg = (double) icon.getIconHeight() / (double) icon.getIconWidth();
int originalWidth = icon.getIconWidth();
int originalHeight = icon.getIconHeight();
double xRatio = (double) view.width / (double) originalWidth;
double yRatio = (double) view.height / (double) originalHeight;
double ratio = 1.0;
if (xRatio < yRatio)
{
ratio = xRatio;
}
else
{
ratio = yRatio;
}
// Calculate the img size for the currentText resolution
double resizedImgWidth = ratio * (float) icon.getIconWidth();
double resizedImgHeight = ratioImg * resizedImgWidth;
if (posx == Integer.MIN_VALUE)
{
posx = (view.width - (int) resizedImgWidth) / 2;
}
if (posy == Integer.MIN_VALUE)
{
posy = (view.height - (int) resizedImgHeight) / 2;
}
// Redimension of the image
Graphics2D g2 = (Graphics2D) g;
g2.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
g2.drawImage(icon.getImage(), posx, posy, (int) resizedImgWidth, (int) resizedImgHeight, null);
}
}
public static void getBestRectangleFor(Rectangle maxView, ImageIcon icon, Rectangle output)
{
int iWidth = icon.getIconWidth();
int iHeight = icon.getIconHeight();
double hRatio = maxView.getWidth() / ((double) iWidth);
double vRatio = maxView.getHeight() / ((double) iHeight);
double ratio = Math.min(hRatio, vRatio);
output.width = (int) (iWidth * ratio);
output.height = (int) (iHeight * ratio);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy