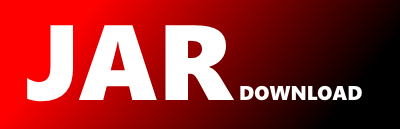
net.alantea.swing.ui.TextTool Maven / Gradle / Ivy
package net.alantea.swing.ui;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Rectangle;
import java.util.HashMap;
import java.util.Map;
import javax.swing.JComponent;
public class TextTool
{
private static Map fontMap = new HashMap<>();
public static void drawString(JComponent comp, Graphics g, String text, Rectangle view)
{
Font fontToUse = getBestFontFor(comp, text, view);
g.setFont(fontToUse);
int refWidth = comp.getFontMetrics(fontToUse).stringWidth(text);
int refHeight = comp.getFontMetrics(fontToUse).getHeight();
int x = view.x + ((view.width - refWidth) / 2);
int y = view.y + ((view.height - refHeight) / 2 + comp.getFontMetrics(fontToUse).getAscent());
g.drawString(text, x, y);
}
public static Font getBestFontFor(JComponent comp, String text, Rectangle view)
{
Font font = comp.getFont();
int refWidth = comp.getFontMetrics(font).stringWidth(text);
int refHeight = comp.getFontMetrics(font).getHeight();
double hRatio = view.width / ((double) refWidth);
double vRatio = view.height / ((double) refHeight);
double ratio = Math.min(hRatio, vRatio);
int targetHeight = (int) (font.getSize() * ratio);
Font targetFont = getResizedFont(font, targetHeight);
int calcWidth = comp.getFontMetrics(targetFont).stringWidth(text);
int calcHeight = comp.getFontMetrics(targetFont).getHeight();
while ((calcWidth < view.width) && (calcHeight < view.height))
{
targetFont = getResizedFont(font, targetHeight + 1);
calcWidth = comp.getFontMetrics(targetFont).stringWidth(text);
calcHeight = comp.getFontMetrics(targetFont).getHeight();
if ((calcWidth < view.width) && (calcHeight < view.height))
{
targetHeight++;
}
}
while ((calcWidth > view.width) && (calcHeight > view.height))
{
targetFont = getResizedFont(font, targetHeight - 1);
calcWidth = comp.getFontMetrics(targetFont).stringWidth(text);
calcHeight = comp.getFontMetrics(targetFont).getHeight();
if ((calcWidth > view.width) && (calcHeight > view.height))
{
targetHeight--;
}
}
return targetFont;
}
private static Font getResizedFont(Font font, int height)
{
Font targetFont = fontMap.get(font.getFamily() + "-" + font.getStyle() + "-" + height);
if (targetFont == null)
{
targetFont = font.deriveFont((float)height);
fontMap.put(font.getFamily() + "-" + font.getStyle() + "-" + height, targetFont);
}
return targetFont;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy