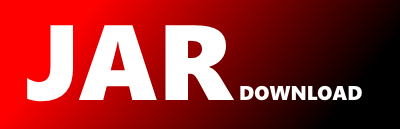
net.alantea.swing.vml.frame.Frame Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.vml.frame;
import java.awt.Color;
import java.awt.Component;
import javax.swing.JComponent;
import javax.swing.JFrame;
import net.alantea.swing.layout.percent.PercentLayout;
import net.alantea.swing.misc.ColorSpace;
import net.alantea.swing.vml.BaseSwingHandler;
import net.alantea.utils.MultiMessages;
import net.alantea.viewml.annotations.VAttribute;
import net.alantea.viewml.annotations.VElement;
import net.alantea.viewml.annotations.VEnd;
@VElement("frame")
public class Frame extends JFrame
{
// -------------------------------------------------------------------------
// ----- Constructor -------------------------------------------------------
// -------------------------------------------------------------------------
/** Default UID. */
private static final long serialVersionUID = 1L;
private static ColorSpace colorSpace;
/**
* Public constructor.
*
* @param name the name
*/
public Frame(String name)
{
super(MultiMessages.get(name));
// sets frame properties
setDefaultCloseOperation(javax.swing.JFrame.DO_NOTHING_ON_CLOSE);
setUndecorated(false);
setLayout(new PercentLayout());
}
/**
* Set full screen.
*
* @param flag the new full screen
*/
@VAttribute("fullscreen")
public void setFullScreen(boolean flag)
{
if (flag)
{
setExtendedState(JFrame.MAXIMIZED_BOTH);
setUndecorated(true);
}
else
{
setExtendedState(JFrame.NORMAL);
}
}
/**
* Set decorated.
*
* @param decorated the new decorated
*/
@VAttribute("decorated")
public void setDecorated(boolean decorated)
{
setUndecorated(! decorated);
}
@Override
public void add(Component comp, Object constraint)
{
if (comp instanceof JComponent)
{
((JComponent) comp).putClientProperty("colorSpace", getColorSpace());
}
super.add(comp, constraint);
}
/**
* Get the application color scheme.
*
* @return the color scheme
*/
public static ColorSpace getColorSpace()
{
if (colorSpace == null)
{
Color color = getColorProperty(MultiMessages.get("Application.colorSpace.base"));
colorSpace = new ColorSpace(color);
}
return colorSpace;
}
/**
* Get a specific color.
*
* @param key Property containing the color to load as #XXXXXX format.
* @return the color or a GRAY default color
*/
public static Color getColorProperty(String key)
{
Color ret = Color.GRAY;
String theKey = MultiMessages.get(key).trim();
try
{
ret = Color.decode(theKey);
}
catch (NumberFormatException e)
{
System.out.println("Bad color found : " + MultiMessages.get(theKey));
}
return ret;
}
@VEnd
private void endComponent(BaseSwingHandler handler)
{
BaseSwingHandler.addComponent((JComponent) getContentPane(), handler);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy