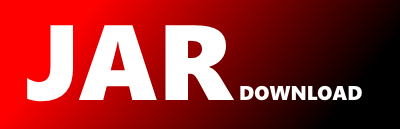
net.alantea.swing.vml.widgets.ButtonHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swingplus Show documentation
Show all versions of swingplus Show documentation
Addons over swing package.
The newest version!
package net.alantea.swing.vml.widgets;
import java.awt.Font;
import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.RenderingHints;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import java.awt.image.BufferedImage;
import javax.swing.AbstractButton;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import net.alantea.horizon.message.Messenger;
import net.alantea.liteprops.Property;
import net.alantea.swing.ui.ButtonUI;
import net.alantea.swing.vml.BaseSwingHandler;
import net.alantea.utils.exception.LntException;
import net.alantea.viewml.VmlAction;
import net.alantea.viewml.VmlException;
import net.alantea.viewml.annotations.VAttribute;
import net.alantea.viewml.annotations.VElement;
import net.alantea.viewml.internal.ActionsLoader;
@VElement("button")
public class ButtonHandler extends BaseSwingHandler
{
private ImageIcon icon;
private VmlAction action;
private String message;
private Property label = new Property<>();
public ButtonHandler()
{
super(new JButton());
init();
}
protected ButtonHandler(JButton target)
{
super(target);
init();
}
private void init()
{
((JButton)getComponent()).setUI(new ButtonUI());
label.addListener((o, n) -> setLabel(n));
getComponent().addComponentListener(new ButtonHandlerResizeListener());
((AbstractButton) getComponent()).addActionListener((e) ->
{
if (action != null)
{
action.invoke();
}
if (message != null)
{
Messenger.sendMessage(getComponent(), message, null);
}
});
}
@VAttribute("action")
private void setAction(String text)
{
try
{
action = ActionsLoader.getAction(text);
}
catch (VmlException e)
{
new LntException("Error setting action", e);
}
}
@VAttribute("message")
private void setMessage(String text)
{
this.message = text;
}
@VAttribute("text")
private void setLabel(String text)
{
((AbstractButton) getComponent()).setText(text);
}
@VAttribute("tooltip")
private void setTooltip(String text)
{
((AbstractButton) getComponent()).setToolTipText(text);
}
@VAttribute("icon")
private void setIcon(String text)
{
icon = new ImageIcon(this.getClass().getResource((text)));
((JButton) getComponent()).setIcon(icon);
}
private Image getScaledImage(ImageIcon srcImg, int w, int h)
{
BufferedImage resizedImg = new BufferedImage(w, h, BufferedImage.TYPE_INT_ARGB);
Graphics2D g2 = resizedImg.createGraphics();
g2.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
g2.drawImage(srcImg.getImage(), 0, 0, w, h, null);
g2.dispose();
return resizedImg;
}
class ButtonHandlerResizeListener extends ComponentAdapter
{
public void componentResized(ComponentEvent e)
{
JButton label = (JButton)getComponent();
int compWidth = label.getWidth();
int compHeight = label.getHeight();
if ((compWidth <= 0) || (compHeight <= 0))
{
return;
}
// Recalculate the image
int iconWidth = (icon == null) ? 0 : icon.getIconWidth();
int iconHeight = (icon == null) ? 0 : icon.getIconHeight();
Image img = getScaledImage(icon, (iconWidth * compHeight) / iconHeight, compHeight);
((JButton) getComponent()).setIcon(new ImageIcon(img));
// Recalculate the text font
int availableWidth = compWidth - ((icon == null) ? 0 : img.getWidth(null));
int availableHeight = compHeight;
Font labelFont = label.getFont();
String labelText = label.getText();
int stringWidth = label.getFontMetrics(labelFont).stringWidth(labelText);
// Find out how much the font can grow in width.
double widthRatio = (double)availableWidth / (double)stringWidth;
int newFontSize = (int)(labelFont.getSize() * widthRatio);
// Pick a new font size so it will not be larger than the height of label.
int fontSizeToUse = Math.min(newFontSize, availableHeight);
// Set the label's font size to the newly determined size.
label.setFont(new Font(labelFont.getName(), labelFont.getStyle(), fontSizeToUse));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy