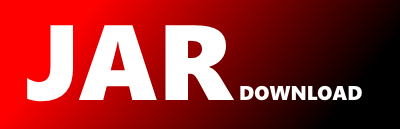
scala_maven.ScalaCompileMojo Maven / Gradle / Ivy
package scala_maven;
import java.io.File;
import java.util.LinkedList;
import java.util.List;
import org.apache.maven.model.Dependency;
/**
* Compiles a directory of Scala source. Corresponds roughly to the compile goal
* of the maven-compiler-plugin
*
* @phase compile
* @goal compile
* @requiresDependencyResolution compile
* @threadSafe
*/
public class ScalaCompileMojo extends ScalaCompilerSupport {
/**
* The directory in which to place compilation output
*
* @parameter property="project.build.outputDirectory"
*/
protected File outputDir;
/**
* The directory which contains scala/java source files
*
* @parameter default-value="${project.build.sourceDirectory}/../scala"
*/
protected File sourceDir;
/**
* Analysis cache file for incremental recompilation.
*
* @parameter property="analysisCacheFile" default-value="${project.build.directory}/analysis/compile"
*/
protected File analysisCacheFile;
@Override
protected List getSourceDirectories() throws Exception {
List sources = project.getCompileSourceRoots();
String scalaSourceDir = FileUtils.pathOf(sourceDir, useCanonicalPath);
if(!sources.contains(scalaSourceDir)) {
sources = new LinkedList(sources); //clone the list to keep the original unmodified
sources.add(scalaSourceDir);
}
return normalize(sources);
}
@Override
protected List getClasspathElements() throws Exception {
List back = project.getCompileClasspathElements();
back.remove(project.getBuild().getOutputDirectory());
//back.add(getOutputDir().getAbsolutePath());
back = TychoUtilities.addOsgiClasspathElements(project, back);
return back;
}
@Override
@Deprecated
protected List getDependencies() {
return project.getCompileDependencies();
}
@Override
protected File getOutputDir() throws Exception {
return outputDir.getAbsoluteFile();
}
@Override
protected File getAnalysisCacheFile() throws Exception {
return analysisCacheFile.getAbsoluteFile();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy