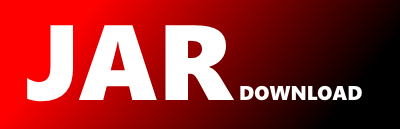
org.ggp.base.apps.exponent.PerMatchLogSummaryGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alloy-ggp-base Show documentation
Show all versions of alloy-ggp-base Show documentation
A modified version of the GGP-Base library for Alloy.
The newest version!
package org.ggp.base.apps.exponent;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.ObjectInputStream;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.ggp.base.util.logging.LogSummaryGenerator;
import org.ggp.base.util.logging.PerMatchTimeSeriesLogger;
import com.google.common.base.Function;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
public class PerMatchLogSummaryGenerator extends LogSummaryGenerator {
private final int playerPort;
public PerMatchLogSummaryGenerator(int playerPort) {
this.playerPort = playerPort;
}
@Override
public String getLogSummary(String matchId) {
return getSummaryFromLogsDirectory(matchId);
}
//Returns info recorded by the perMatchLogger.
@Override
public String getSummaryFromLogsDirectory(String matchId) {
// TODO Implement
//First, load everything from the directory for the match
File matchFolder = PerMatchTimeSeriesLogger.getMatchFolder(matchId, playerPort);
//Wait a few seconds for everything to get written out, because we can...
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
//This is probably bad...
e.printStackTrace();
throw new RuntimeException(e);
}
Map> timeSeries = loadAllTimeSeries(matchFolder);
Map> pointsTimeSeries = toPointsTimeSeries(timeSeries);
return toJson(pointsTimeSeries);
}
private Map> toPointsTimeSeries(
Map> timeSeries) {
Map> result = Maps.newHashMap();
for (String key : timeSeries.keySet()) {
Map series = timeSeries.get(key);
result.put(key, toPointSeries(series));
}
return result;
}
private List toPointSeries(Map series) {
List result = Lists.newArrayList();
for (Entry entry : series.entrySet()) {
result.add(new DoublePair(entry));
}
Collections.sort(result);
return result;
}
private static class DoublePair implements Comparable {
public final long x;
public final double y;
public DoublePair(long x, double y) {
this.x = x;
this.y = y;
}
public DoublePair(Entry entry) {
this.x = entry.getKey();
this.y = entry.getValue();
}
@Override
public String toString() {
return "[" + x + ", " + y + "]";
}
@Override
public int compareTo(DoublePair o) {
return Long.compare(x, o.x);
}
}
private String toJson(Map> pointsTimeSeries) {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
for (Entry> ts : pointsTimeSeries.entrySet()) {
sb.append(" \"");
sb.append(ts.getKey());
sb.append("\": ");
sb.append(ts.getValue().toString());
sb.append(",\n");
}
if (sb.length() > 3) {
sb.delete(sb.length() - 2, sb.length());
}
sb.append("\n}");
return sb.toString();
}
private String toJsonOriginal(Map> timeSeries) {
StringBuilder sb = new StringBuilder();
sb.append("{\n");
for (Entry> ts : timeSeries.entrySet()) {
sb.append(" \"");
sb.append(ts.getKey());
sb.append("\": ");
sb.append(ts.getValue().toString());
sb.append(",\n");
}
if (sb.length() > 3) {
sb.delete(sb.length() - 2, sb.length());
}
sb.append("\n}");
return sb.toString();
}
private Map> loadAllTimeSeries(File matchFolder) {
Map> rawTimeSeries = Maps.newHashMap();
// For now, all files should be time series
for (File tsFile : matchFolder.listFiles()) {
try {
try (ObjectInputStream in = new ObjectInputStream(new BufferedInputStream(new FileInputStream(tsFile)))) {
Map recordedTimeSeries = (Map) in.readObject();
rawTimeSeries.put(tsFile.getName().split("\\.")[0], recordedTimeSeries);
}
} catch (Exception e) {
e.printStackTrace();
}
}
return toStandardizedTimeSeries(rawTimeSeries);
}
private Map> toStandardizedTimeSeries(
Map> rawTimeSeries) {
final long firstRecordedTime = getFirstRecordedTime(rawTimeSeries);
return Maps.transformValues(rawTimeSeries, new Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy