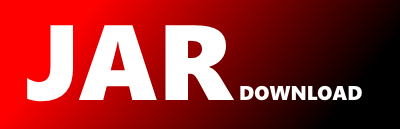
org.ggp.base.util.gdl.model.ImmutableSentenceFormModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alloy-ggp-base Show documentation
Show all versions of alloy-ggp-base Show documentation
A modified version of the GGP-Base library for Alloy.
The newest version!
package org.ggp.base.util.gdl.model;
import javax.annotation.concurrent.Immutable;
import org.ggp.base.util.gdl.grammar.Gdl;
import org.ggp.base.util.gdl.grammar.GdlRule;
import org.ggp.base.util.gdl.grammar.GdlSentence;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMultimap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.ImmutableSetMultimap;
@Immutable
public class ImmutableSentenceFormModel implements SentenceFormModel {
private final ImmutableList gameDescription;
private final ImmutableSet sentenceForms;
private final ImmutableSet constantSentenceForms;
private final ImmutableSet independentSentenceForms;
private final ImmutableSetMultimap dependencyGraph;
private final ImmutableSetMultimap rulesByForm;
private final ImmutableSetMultimap trueSentencesByForm;
public ImmutableSentenceFormModel(ImmutableList gameDescription,
ImmutableSet sentenceForms,
ImmutableSet constantSentenceForms,
ImmutableSet independentSentenceForms,
ImmutableSetMultimap dependencyGraph,
ImmutableSetMultimap rulesByForm,
ImmutableSetMultimap trueSentencesByForm) {
Preconditions.checkArgument(sentenceForms.containsAll(independentSentenceForms));
Preconditions.checkArgument(independentSentenceForms.containsAll(constantSentenceForms));
Preconditions.checkArgument(sentenceForms.containsAll(dependencyGraph.keySet()));
Preconditions.checkArgument(sentenceForms.containsAll(dependencyGraph.values()));
Preconditions.checkArgument(sentenceForms.containsAll(rulesByForm.keySet()));
Preconditions.checkArgument(sentenceForms.containsAll(trueSentencesByForm.keySet()));
this.gameDescription = gameDescription;
this.sentenceForms = sentenceForms;
this.constantSentenceForms = constantSentenceForms;
this.independentSentenceForms = independentSentenceForms;
this.dependencyGraph = dependencyGraph;
this.rulesByForm = rulesByForm;
this.trueSentencesByForm = trueSentencesByForm;
}
/**
* Returns an ImmutableSentenceFormModel with the same contents as the
* given SentenceFormModel.
*
* May not actually create a copy if the input is immutable.
*/
public static ImmutableSentenceFormModel copyOf(SentenceFormModel other) {
if (other instanceof ImmutableSentenceDomainModel) {
return copyOf(((ImmutableSentenceDomainModel) other).getFormModel());
} else if (other instanceof ImmutableSentenceFormModel) {
return (ImmutableSentenceFormModel) other;
}
ImmutableSetMultimap.Builder rulesByForm = ImmutableSetMultimap.builder();
ImmutableSetMultimap.Builder trueSentencesByForm = ImmutableSetMultimap.builder();
for (SentenceForm form : other.getSentenceForms()) {
rulesByForm.putAll(form, other.getRules(form));
trueSentencesByForm.putAll(form, other.getSentencesListedAsTrue(form));
}
return new ImmutableSentenceFormModel(ImmutableList.copyOf(other.getDescription()),
ImmutableSet.copyOf(other.getSentenceForms()),
ImmutableSet.copyOf(other.getConstantSentenceForms()),
ImmutableSet.copyOf(other.getIndependentSentenceForms()),
ImmutableSetMultimap.copyOf(other.getDependencyGraph()),
rulesByForm.build(),
trueSentencesByForm.build());
}
//TODO: Come up with an implementation that can save memory relative to this
//(i.e. where we can reuse SentenceForm references)
@Override
public SentenceForm getSentenceForm(GdlSentence sentence) {
return SimpleSentenceForm.create(sentence);
}
@Override
public ImmutableSet getIndependentSentenceForms() {
return independentSentenceForms;
}
@Override
public ImmutableSet getConstantSentenceForms() {
return constantSentenceForms;
}
@Override
public ImmutableMultimap getDependencyGraph() {
return dependencyGraph;
}
@Override
public ImmutableSet getSentencesListedAsTrue(SentenceForm form) {
return trueSentencesByForm.get(form);
}
@Override
public ImmutableSet getRules(SentenceForm form) {
return rulesByForm.get(form);
}
@Override
public ImmutableSet getSentenceForms() {
return sentenceForms;
}
@Override
public ImmutableList getDescription() {
return gameDescription;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy