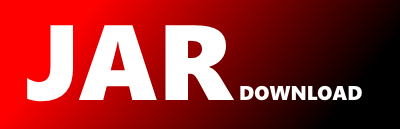
org.ggp.base.util.propnet.factory.PropNetOrderingBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alloy-ggp-base Show documentation
Show all versions of alloy-ggp-base Show documentation
A modified version of the GGP-Base library for Alloy.
The newest version!
package org.ggp.base.util.propnet.factory;
import java.util.List;
import java.util.Set;
import org.ggp.base.util.gdl.model.DependencyGraphs;
import org.ggp.base.util.propnet.architecture.Component;
import org.ggp.base.util.propnet.architecture.PropNet;
import org.ggp.base.util.propnet.architecture.components.Transition;
import com.google.common.base.Predicates;
import com.google.common.collect.HashMultimap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.ImmutableSetMultimap;
import com.google.common.collect.Lists;
import com.google.common.collect.SetMultimap;
import com.google.common.collect.Sets;
public class PropNetOrderingBuilder {
private final ImmutableSet allComponents;
private final ImmutableSetMultimap dependencies;
private final List layers = Lists.newArrayList();
private static interface Layer {
List orderComponents(Set addedSoFar) throws InterruptedException;
}
private PropNetOrderingBuilder(ImmutableSet allComponents,
ImmutableSetMultimap dependencies) {
this.allComponents = allComponents;
this.dependencies = dependencies;
}
public static PropNetOrderingBuilder builder(PropNet pn) {
return new PropNetOrderingBuilder(ImmutableSet.copyOf(pn.getComponents()), computeDependencies(pn.getComponents()));
}
private static ImmutableSetMultimap computeDependencies(Set components) {
SetMultimap dependencies = HashMultimap.create();
for (Component component : components) {
if (!(component instanceof Transition)) {
//Add its outputs
for (Component output : component.getOutputs()) {
dependencies.put(output, component);
}
}
}
return ImmutableSetMultimap.copyOf(dependencies);
}
public PropNetOrderingBuilder addComponents(Set extends Component> components) {
layers.add(new Layer() {
@Override
public List orderComponents(Set addedSoFar) throws InterruptedException {
List ordering = Lists.newArrayList();
List> strata = DependencyGraphs.toposortSafe(components, dependencies);
for (Set stratum : strata) {
for (Component component : stratum) {
if (!addedSoFar.contains(component)) {
//TODO: Verify that all dependencies have already been added
ordering.add(component);
}
}
}
return ordering;
}
});
return this;
}
public PropNetOrderingBuilder addComponentsAndAncestors(Set extends Component> components) {
addAncestorsOf(components);
addComponents(components);
return this;
}
public PropNetOrderingBuilder addComponentsAndAncestors(Component component, Component... moreComponents) {
return addComponentsAndAncestors(Sets.newHashSet(Lists.asList(component, moreComponents)));
}
public PropNetOrderingBuilder addComponentsInOrder(List extends Component> components) {
layers.add(new Layer() {
@Override
public List orderComponents(Set addedSoFar) throws InterruptedException {
List ordering = Lists.newArrayList();
for (Component component : components) {
if (!addedSoFar.contains(component)) {
//TODO: Verify that all dependencies have already been added
ordering.add(component);
}
}
return ordering;
}
});
return this;
}
public PropNetOrderingBuilder addAncestorsOf(Set extends Component> components) {
ImmutableSet matchingAndUpstream = DependencyGraphs.getMatchingAndUpstream(allComponents, dependencies, Predicates.in(components));
Set upstreamOnly = ImmutableSet.copyOf(Sets.difference(matchingAndUpstream, components));
return addComponents(upstreamOnly);
}
public PropNetOrderingBuilder addAncestorsOf(Component component, Component... moreComponents) {
return addAncestorsOf(Sets.newHashSet(Lists.asList(component, moreComponents)));
}
public List build() throws InterruptedException {
List ordering = Lists.newArrayList();
Set addedSoFar = Sets.newHashSet();
for (Layer layer : layers) {
List order = layer.orderComponents(addedSoFar);
ordering.addAll(order);
addedSoFar.addAll(order);
}
return ordering;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy