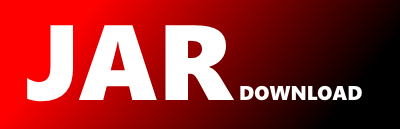
net.alloyggp.escaperope.CsvFieldEscaper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of escape-rope Show documentation
Show all versions of escape-rope Show documentation
A library for turning structured data into strings and back.
package net.alloyggp.escaperope;
import java.util.ArrayList;
import java.util.List;
//Quotes the string, turning quotes inside into double-quotes.
//Unescaping recognizes an unescaped comma as a control character.
public class CsvFieldEscaper implements Escaper {
private final NullBehavior nullBehavior;
private CsvFieldEscaper(NullBehavior nullBehavior) {
this.nullBehavior = nullBehavior;
}
public static Escaper create(NullBehavior nullBehavior) {
return new CsvFieldEscaper(nullBehavior);
}
@Override
public String escape(String input) {
input = nullBehavior.apply(input);
if (input == null) {
return "null";
}
StringBuilder sb = new StringBuilder();
sb.append('"');
sb.append(input.replaceAll("\"", "\"\""));
sb.append('"');
return sb.toString();
}
@Override
public List unescape(String input) {
if (input == null) {
throw new NullPointerException();
}
List results = new ArrayList<>();
boolean insideQuotes = false;
StringBuilder currentString = new StringBuilder();
for (int i = 0; i < input.length(); /* incremented manually */) {
int codePoint = input.codePointAt(i);
if (codePoint == '"') {
if (insideQuotes) {
if (i < input.length() - 1 && input.codePointAt(i+1) == '"') {
currentString.append('"');
i++;
} else {
insideQuotes = false;
results.add(UnescapeTextResult.create(currentString.toString()));
currentString = new StringBuilder();
}
} else {
insideQuotes = true;
}
} else if (codePoint == ',' && !insideQuotes) {
results.add(UnescapeControlResult.create(','));
} else if (!insideQuotes
&& nullBehavior == NullBehavior.KEEP
&& codePoint == 'n'
&& i + 3 < input.length()
&& input.codePointAt(i + 1) == 'u'
&& input.codePointAt(i + 2) == 'l'
&& input.codePointAt(i + 3) == 'l') {
results.add(UnescapeTextResult.create(null));
i += 3;
} else if (!insideQuotes) {
throw new IllegalArgumentException("This wasn't generated by the CsvFieldEscaper or CsvLineDelimiter, so we don't know how to deal with it");
} else {
currentString.appendCodePoint(codePoint);
}
i += Character.charCount(codePoint);
}
return results;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy