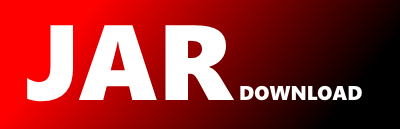
net.alloyggp.escaperope.rope.ropify.RopeList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of escape-rope Show documentation
Show all versions of escape-rope Show documentation
A library for turning structured data into strings and back.
package net.alloyggp.escaperope.rope.ropify;
import java.util.Iterator;
import java.util.List;
import javax.annotation.concurrent.Immutable;
import net.alloyggp.escaperope.rope.Rope;
/**
* A utility class for reading transformed contents of a ListRope.
* The RopeList wraps the Rope and provides useful getters for
* applying {@link RopeWeaver}s and core weavers.
*/
@Immutable
public class RopeList implements Iterable {
private final List list;
private RopeList(List list) {
this.list = list;
}
public static RopeList create(Rope listRope) {
if (!listRope.isList()) {
throw new IllegalArgumentException("Input must be a list-type Rope");
}
//This is safe because we know the list we're given by ListRope is immutable
return new RopeList(listRope.asList());
}
public int size() {
return list.size();
}
//TODO: More compact implementation?
public boolean getBoolean(int i) {
return Boolean.parseBoolean(list.get(i).asString());
}
public T get(int i, RopeWeaver weaver) {
return weaver.fromRope(list.get(i));
}
public int getInt(int i) {
return Integer.parseInt(list.get(i).asString());
}
public long getLong(int i) {
return Long.parseLong(list.get(i).asString());
}
public String getString(int i) {
return list.get(i).asString();
}
@Override
public Iterator iterator() {
return list.iterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy