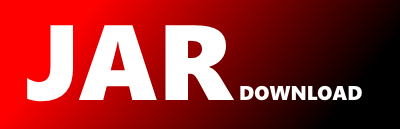
net.amygdalum.util.builders.SortedSets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compilerutils Show documentation
Show all versions of compilerutils Show documentation
Utility classes needed for search and compiler applications
The newest version!
package net.amygdalum.util.builders;
import static java.util.Arrays.asList;
import java.util.Collection;
import java.util.Comparator;
import java.util.HashSet;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
public final class SortedSets {
private SortedSet set;
private SortedSets() {
this.set = new TreeSet();
}
private SortedSets(Comparator super T> comparator) {
this.set = new TreeSet(comparator);
}
private SortedSets(Collection extends T> set) {
this.set = new TreeSet(set);
}
public static SortedSets tree(Comparator super T> comparator) {
return new SortedSets(comparator);
}
@SafeVarargs
public static SortedSets tree(T... elements) {
if (elements.length == 0) {
return new SortedSets();
}
return new SortedSets(asList(elements));
}
public static SortedSets tree(Collection set) {
return new SortedSets(set);
}
@SafeVarargs
public static SortedSet of(T... elements) {
return new TreeSet(asList(elements));
}
@SafeVarargs
public static SortedSet of(Predicate cond, T... elements) {
TreeSet list = new TreeSet();
for (T element : elements) {
if (cond.evaluate(element)) {
list.add(element);
}
}
return list;
}
public static SortedSet ofPrimitives(int... array) {
TreeSet set = new TreeSet();
for (int i : array) {
set.add(i);
}
return set;
}
public static SortedSet intersectionOf(Set set, Set other) {
return new SortedSets(set).intersect(other).build();
}
public static SortedSet unionOf(Set set, Set other) {
return new SortedSets(set).union(other).build();
}
public static SortedSet complementOf(Set set, Set minus) {
return new SortedSets(set).minus(minus).build();
}
public SortedSets union(Set add) {
return addAll(add);
}
public SortedSets add(T add) {
set.add(add);
return this;
}
public SortedSets addConditional(boolean b, T add) {
if (b) {
set.add(add);
}
return this;
}
public SortedSets addAll(Set add) {
set.addAll(add);
return this;
}
@SuppressWarnings("unchecked")
public SortedSets addAll(T... add) {
set.addAll(asList(add));
return this;
}
public SortedSets minus(Set remove) {
return removeAll(remove);
}
public SortedSets remove(T remove) {
set.remove(remove);
return this;
}
public SortedSets removeConditional(boolean b, T remove) {
if (b) {
set.remove(remove);
}
return this;
}
public SortedSets removeAll(Set remove) {
set.removeAll(remove);
return this;
}
@SuppressWarnings("unchecked")
public SortedSets removeAll(T... remove) {
set.removeAll(asList(remove));
return this;
}
public SortedSets intersect(Set retain) {
return retainAll(retain);
}
public SortedSets retain(T retain) {
Set retainAll = new HashSet();
retainAll.add(retain);
set.retainAll(retainAll);
return this;
}
public SortedSets retainConditional(boolean b, T retain) {
if (b) {
Set retainAll = new HashSet();
retainAll.add(retain);
set.retainAll(retainAll);
}
return this;
}
public SortedSets retainAll(Set retain) {
set.retainAll(retain);
return this;
}
@SuppressWarnings("unchecked")
public SortedSets retainAll(T... retain) {
set.retainAll(asList(retain));
return this;
}
public SortedSet build() {
return set;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy