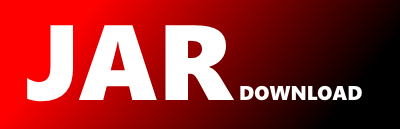
net.amygdalum.stringsearchalgorithms.search.chars.EmptyMatchFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stringsearchalgorithms Show documentation
Show all versions of stringsearchalgorithms Show documentation
Searching and Matching Strings with efficient algorithms:
- Knuth-Morris-Pratt
- Shift-And/Or
- Boyer-Moore-Horspool
- Sunday (QuickSearch)
- BNDM
- BOM
- Aho-Corasick
- Set-Horspool
- Wu-Manber
- Set-BOM
package net.amygdalum.stringsearchalgorithms.search.chars;
import static net.amygdalum.stringsearchalgorithms.search.MatchOption.LONGEST_MATCH;
import net.amygdalum.stringsearchalgorithms.search.BufferedStringFinder;
import net.amygdalum.stringsearchalgorithms.search.StringFinder;
import net.amygdalum.stringsearchalgorithms.search.StringFinderOption;
import net.amygdalum.stringsearchalgorithms.search.StringMatch;
import net.amygdalum.util.io.CharProvider;
public class EmptyMatchFinder extends BufferedStringFinder {
private StringFinder finder;
private CharProvider chars;
private boolean longest;
public EmptyMatchFinder(StringFinder finder, CharProvider chars, StringFinderOption... options) {
super(options);
this.finder = finder;
this.chars = chars;
this.longest = LONGEST_MATCH.in(options);
}
@Override
public StringMatch findNext() {
if (isBufferEmpty()) {
long start = chars.current();
StringMatch next = finder.findNext();
if (next != null) {
push(next);
for (long pos = start; pos < next.start(); pos++) {
push(new StringMatch(pos, pos, ""));
}
} else {
for (long pos = start; pos < chars.current(); pos++) {
push(new StringMatch(pos, pos, ""));
}
}
}
if (longest) {
return longestLeftMost();
} else {
return leftMost();
}
}
@Override
public void skipTo(long pos) {
finder.skipTo(pos);
clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy